Building Responsive Websites with jQuery
In today’s digital landscape, it is essential for websites to be responsive. With the increasing number of devices and screen sizes, users expect websites to adapt seamlessly to their screens. Responsive web design ensures that websites look and function optimally, regardless of the device being used. One powerful tool that can aid in building responsive websites is jQuery. In this blog post, we will explore the importance of responsive design, discuss responsive techniques, and dive into the implementation of responsive websites using jQuery.
1. Understanding Responsive Web Design
Responsive web design is an approach that focuses on creating websites that automatically adjust their layout and content based on the user’s screen size and orientation. It ensures an optimal viewing experience by providing easy navigation, legible text, and well-aligned elements across different devices.
2. Key Responsive Design Techniques
Before diving into jQuery, it’s important to understand the key techniques used in responsive web design:
2.1 Fluid Grids
Fluid grids allow the layout of a website to adapt dynamically to different screen sizes. Instead of using fixed pixel-based widths, fluid grids utilize percentage-based widths, ensuring that elements resize proportionally to fit the screen. This technique is essential for achieving a flexible and responsive layout.
2.2 Media Queries
Media queries enable the adaptation of a website’s layout and styles based on specific conditions, such as screen size, resolution, and device orientation. By using media queries, developers can define different stylesheets or apply CSS rules selectively, optimizing the website’s appearance for each device.
2.3 Flexible Images
Images can pose a challenge when it comes to responsive design, as they often have fixed dimensions. To make images responsive, CSS techniques like max-width: 100% can be applied to ensure they scale proportionally and fit within their containers. This allows images to adjust fluidly as the screen size changes.
2.4 Mobile-First Approach
The mobile-first approach involves designing and developing a website primarily for mobile devices and then progressively enhancing it for larger screens. By starting with a mobile-centric design, the focus is on delivering essential content and functionality in a lightweight manner. This approach ensures a better user experience on small screens and simplifies the scaling process for larger screens.
3. Getting Started with jQuery
jQuery is a popular JavaScript library that simplifies the process of creating interactive and dynamic web pages. It provides a wide range of functions and methods that make it easier to manipulate the Document Object Model (DOM) and handle events. Before incorporating jQuery into a responsive website, it’s essential to include the library in the project.
To add jQuery to your project, you can either download it from the official website or use a Content Delivery Network (CDN) to link to the hosted version. For example, you can include jQuery using the following code snippet:
html <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
Once jQuery is included, you can start leveraging its features to build responsive components and enhance your website’s responsiveness.
4. Building Responsive Websites with jQuery
Now, let’s explore some practical examples of building responsive components using jQuery:
4.1 Responsive Navigation Menu
A responsive navigation menu is crucial for a seamless user experience across devices. jQuery can simplify the process of creating a responsive menu that collapses into a toggleable button on smaller screens. Here’s an example:
html <nav> <div class="menu-toggle"> <i class="fa fa-bars"></i> </div> <ul class="menu"> <li><a href="#">Home</a></li> <li><a href="#">About</a></li> <li><a href="#">Services</a></li> <li><a href="#">Contact</a></li> </ul> </nav> <script> $(document).ready(function() { $(".menu-toggle").click(function() { $(".menu").slideToggle(); }); }); </script>
In the above example, when the menu toggle button is clicked, the slideToggle() function is used to show or hide the menu.
4.2 Responsive Image Slider
Image sliders are commonly used to showcase multiple images or featured content. With jQuery, you can create a responsive image slider that adjusts its size and navigation based on the screen width. Here’s a sample code snippet:
html <div class="slider"> <div class="slide"><img src="image1.jpg" alt="Image 1"></div> <div class="slide"><img src="image2.jpg" alt="Image 2"></div> <div class="slide"><img src="image3.jpg" alt="Image 3"></div> </div> <script> $(document).ready(function() { $(".slider").slick({ dots: true, responsive: [ { breakpoint: 768, settings: { arrows: false } } ] }); }); </script>
In this example, we’re using the popular jQuery plugin called Slick to create the responsive image slider. The responsive option allows us to define different settings for different breakpoints.
4.3 Responsive Tables
Tables can become challenging to read and navigate on small screens. jQuery can help transform traditional tables into responsive ones by converting them into scrollable or collapsible elements. Here’s an example:
html <table class="responsive-table"> <thead> <tr> <th>Header 1</th> <th>Header 2</th> <th>Header 3</th> </tr> </thead> <tbody> <tr> <td>Data 1</td> <td>Data 2</td> <td>Data 3</td> </tr> <!-- more table rows --> </tbody> </table> <script> $(document).ready(function() { $(".responsive-table").stacktable(); }); </script>
The .stacktable() function from the Stacktable jQuery plugin is used to convert the table into a responsive format, displaying each table row as a stacked element on small screens.
Conclusion
In this blog post, we have explored the importance of responsive web design and discussed key techniques involved in building responsive websites. We have also seen how jQuery can be used to enhance responsiveness and create responsive components like navigation menus, image sliders, and tables. By leveraging the power of jQuery, developers can build websites that adapt seamlessly to different devices, providing an optimal user experience across the board. So, embrace the flexibility and interactivity offered by jQuery and start building your responsive websites today!
Table of Contents
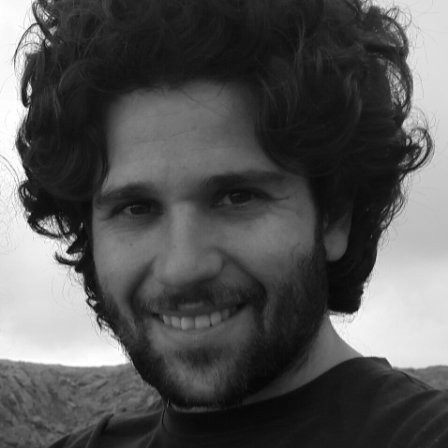
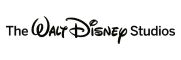