Building Single-Page Applications with jQuery and AJAX
In the fast-paced world of web development, Single-Page Applications (SPAs) have gained immense popularity due to their ability to provide a fluid and engaging user experience. These applications load a single HTML page and dynamically update content without the need for full page reloads. One of the key technologies that facilitate the creation of SPAs is jQuery, combined with AJAX (Asynchronous JavaScript and XML). In this article, we’ll explore how to build compelling SPAs using jQuery and AJAX, covering essential concepts, techniques, and code samples.
Table of Contents
1. Understanding Single-Page Applications (SPAs)
Traditional web applications involve navigating between different pages, resulting in frequent loading times and disruptions in user interaction. SPAs, on the other hand, offer a seamless experience by fetching data and updating only specific sections of the page as needed. This is achieved through asynchronous communication with the server, primarily using AJAX.
2. The Power of AJAX
AJAX, short for Asynchronous JavaScript and XML, is a set of web development techniques that allow data to be retrieved from the server without requiring a full page reload. While XML is used less frequently nowadays, the concept has evolved to include various data formats, such as JSON, which is widely adopted due to its simplicity and compatibility with JavaScript objects.
3. Leveraging jQuery for Enhanced Interactivity
jQuery, a fast and feature-rich JavaScript library, simplifies DOM manipulation, event handling, and AJAX requests. It provides a concise syntax that abstracts browser inconsistencies and complexities, allowing developers to focus on building functionality rather than worrying about low-level details.
4. Getting Started with jQuery
To get started, include the jQuery library in your HTML file. You can either download it and host it locally or use a content delivery network (CDN) for faster loading:
html <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
Once jQuery is included, you can start using its powerful features. For example, to select an element by its ID and update its content:
javascript $("#elementID").text("New content");
5. Making AJAX Requests with jQuery
AJAX is at the core of creating dynamic SPAs. jQuery simplifies the process of making AJAX requests using the $.ajax() function. Let’s take a look at a basic example of fetching data from a server and updating the page content:
javascript $.ajax({ url: "https://api.example.com/data", method: "GET", dataType: "json", success: function(data) { $("#content").html(data.content); }, error: function(error) { console.log("An error occurred: " + error); } });
In this example, we’re fetching JSON data from a server and updating the content of an element with the ID content. The success function is executed when the request is successful, and the error function handles any errors that may occur during the request.
6. Building a Simple SPA
Let’s walk through the process of building a basic SPA that fetches and displays content without a page reload.
6.1. HTML Structure
Start by setting up the HTML structure for your SPA. You’ll need a container where the content will be displayed and navigation elements to switch between different sections:
html <!DOCTYPE html> <html> <head> <title>Simple SPA</title> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> </head> <body> <header> <nav> <a href="#" class="nav-link" data-section="home">Home</a> <a href="#" class="nav-link" data-section="about">About</a> <a href="#" class="nav-link" data-section="contact">Contact</a> </nav> </header> <main> <div id="content"></div> </main> <script src="spa.js"></script> </body> </html>
6.2. Implementing the JavaScript
Create a new file named spa.js and implement the functionality using jQuery and AJAX:
javascript $(document).ready(function() { $(".nav-link").click(function(event) { event.preventDefault(); var section = $(this).data("section"); loadContent(section); }); function loadContent(section) { $.ajax({ url: "https://api.example.com/content/" + section, method: "GET", dataType: "html", success: function(data) { $("#content").html(data); }, error: function(error) { console.log("An error occurred: " + error); } }); } });
In this JavaScript code, we’re attaching a click event listener to the navigation links. When a link is clicked, we prevent the default link behavior and retrieve the data-section attribute to identify the section to load. The loadContent() function makes an AJAX request to fetch the corresponding HTML content and updates the #content element.
7. Enhancing the SPA
To make your SPA more dynamic and interactive, consider implementing features like client-side routing, animations, and real-time updates using web sockets. Additionally, ensure that your application gracefully handles errors and provides meaningful feedback to users.
Conclusion
Building Single-Page Applications with jQuery and AJAX opens the door to creating seamless, responsive, and engaging user experiences. By leveraging jQuery’s powerful DOM manipulation and AJAX capabilities, developers can craft dynamic applications that fetch and display content without the need for full page reloads. Whether you’re developing a simple portfolio website or a complex web application, mastering these techniques will enable you to deliver a smooth and modern user experience. Remember to keep exploring and experimenting with different approaches to continually improve your SPA development skills. Happy coding!
Table of Contents
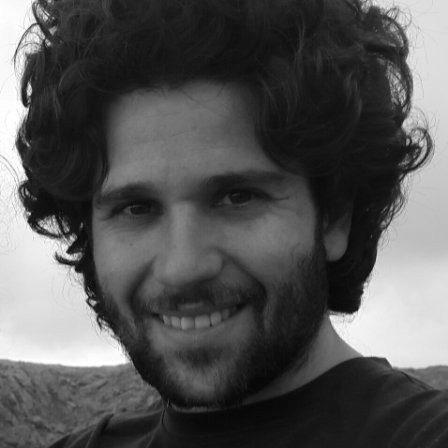
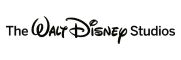