Building Sliders and Carousels with jQuery
In today’s web development landscape, creating visually appealing and interactive content is essential to engage users and keep them immersed in your website’s experience. One effective way to achieve this is by incorporating sliders and carousels into your design. These dynamic elements allow you to showcase multiple pieces of content within a limited space, making it ideal for highlighting key features, products, or stories.
Table of Contents
In this tutorial, we’ll dive into the world of sliders and carousels, exploring how to build them from scratch using the popular JavaScript library, jQuery. Whether you’re a seasoned developer or just starting your coding journey, this guide will provide you with the knowledge and tools to create captivating sliders and carousels that enhance your website’s user experience.
1. Introduction to Sliders and Carousels
1.1. Understanding the Purpose and Benefits
Sliders and carousels are widely used UI components that enable you to display multiple pieces of content in a confined space. They are particularly useful when you want to highlight important features, showcase products, or tell a visual story within a limited area of your website. Sliders and carousels catch the users’ attention and encourage them to explore more content, enhancing user engagement and interaction.
1.2. Examples of Sliders and Carousels in Websites
You’ve likely encountered sliders and carousels on various websites. E-commerce platforms often use carousels to display featured products, discounts, or new arrivals. News websites utilize sliders to present top stories or breaking news updates. Even portfolio websites make use of sliders to showcase a collection of projects or artworks.
These examples demonstrate the versatility and effectiveness of sliders and carousels in delivering content to users in an engaging and visually appealing manner.
2. Setting Up the Project
2.1. Creating the HTML Structure
To start building our slider or carousel, we need to set up the basic HTML structure. Let’s assume we’re building a simple image slider. Here’s an example of how your HTML could look:
html <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Image Slider</title> <link rel="stylesheet" href="styles.css"> </head> <body> <div class="slider"> <div class="slide"><img src="image1.jpg" alt="Slide 1"></div> <div class="slide"><img src="image2.jpg" alt="Slide 2"></div> <div class="slide"><img src="image3.jpg" alt="Slide 3"></div> </div> <script src="jquery.min.js"></script> <script src="script.js"></script> </body> </html>
In this example, we’ve created a basic HTML structure with a container div for the slider and three slide divs, each containing an image. Make sure to replace the image sources with actual image URLs.
2.2. Linking jQuery Library
To use jQuery in our project, we need to include the jQuery library. You can download the jQuery library and host it on your server or use a Content Delivery Network (CDN) link. Place the following script tag just before the closing </body> tag in your HTML:
html <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
With the basic setup in place, we can now proceed to build our image slider using jQuery.
3. Building a Basic Slider
3.1. Writing the CSS for Styling
Before we dive into jQuery code, let’s style our slider with some CSS. Create a styles.css file and add the following styles:
css /* Reset some default styles */ body, ul { margin: 0; padding: 0; list-style: none; } /* Style the slider container */ .slider { width: 100%; overflow: hidden; position: relative; } /* Style individual slides */ .slide { width: 100%; height: auto; display: none; }
In this CSS, we set up the basic styles for the slider container and individual slides. We hide the slides by default using display: none;.
3.2. Using jQuery to Implement the Slider Logic
Next, let’s create a script.js file and add the jQuery code to implement the slider functionality:
javascript $(document).ready(function() { // Get all slide elements var slides = $('.slide'); // Initialize index for tracking the current slide var currentIndex = 0; // Show the first slide and hide the rest $(slides[currentIndex]).show(); // Function to display the next slide function showNextSlide() { $(slides[currentIndex]).fadeOut(); currentIndex = (currentIndex + 1) % slides.length; $(slides[currentIndex]).fadeIn(); } // Interval for automatic slide transition var slideInterval = setInterval(showNextSlide, 3000); // Pause the slide transition on hover $('.slider').hover(function() { clearInterval(slideInterval); }, function() { slideInterval = setInterval(showNextSlide, 3000); }); });
In this jQuery code, we wait for the document to be ready using $(document).ready(). We select all the slide elements and initialize a variable currentIndex to keep track of the currently displayed slide.
The showNextSlide function hides the current slide, updates the index to the next slide, and then fades in the new slide. We use the modulo operator to loop back to the first slide after reaching the last slide.
We also set an interval using setInterval to automatically transition to the next slide every 3 seconds. Additionally, we pause the slide transition when hovering over the slider using the .hover() function.
With these pieces of code in place, you’ve successfully created a basic image slider using jQuery. Users will see a fading transition between slides, and the slider will automatically advance to the next slide every few seconds.
4. Creating a Carousel with Navigation
4.1. Structuring Carousel Items
While a slider displays one slide at a time, a carousel usually displays multiple items simultaneously. Let’s transform our basic slider into a carousel that displays three slides at a time. Update your HTML structure like this:
html <div class="carousel"> <div class="carousel-container"> <div class="carousel-item"><img src="image1.jpg" alt="Item 1"></div> <div class="carousel-item"><img src="image2.jpg" alt="Item 2"></div> <div class="carousel-item"><img src="image3.jpg" alt="Item 3"></div> <div class="carousel-item"><img src="image4.jpg" alt="Item 4"></div> <!-- Add more items as needed --> </div> </div>
4.2. Implementing Navigation Buttons
Now, let’s add navigation buttons to allow users to move the carousel left and right. Update your script.js file with the following code:
javascript $(document).ready(function() { var carousel = $('.carousel'); var carouselContainer = $('.carousel-container'); var carouselItems = $('.carousel-item'); // Initialize index for tracking the current carousel position var currentPosition = 0; // Show the initial set of carousel items carouselContainer.css('transform', `translateX(${currentPosition}%)`); // Function to move the carousel left function moveLeft() { if (currentPosition < 0) { currentPosition += 100; carouselContainer.css('transform', `translateX(${currentPosition}%)`); } } // Function to move the carousel right function moveRight() { if (currentPosition > -100 * (carouselItems.length - 3)) { currentPosition -= 100; carouselContainer.css('transform', `translateX(${currentPosition}%)`); } } // Attach click handlers to navigation buttons $('.prev-btn').click(moveLeft); $('.next-btn').click(moveRight); });
In this code, we’ve added two functions, moveLeft and moveRight, which adjust the currentPosition and move the carousel container accordingly using the translateX property in CSS. We’ve also attached click handlers to previous and next buttons to trigger these functions.
Additionally, we’ve updated the HTML to include navigation buttons:
html <div class="carousel"> <div class="carousel-container"> <!-- carousel items --> </div> <button class="prev-btn">Previous</button> <button class="next-btn">Next</button> </div>
With these changes, your image slider has transformed into a functional carousel with navigation buttons that allow users to move left and right through the carousel items.
5. Adding Animation and Transitions
5.1. Using CSS Transitions for Smooth Animation
To enhance the user experience, let’s add smooth sliding animations using CSS transitions. Update your styles.css file with the following changes:
css /* Style the carousel container */ .carousel-container { width: calc(100% * 4); /* Total width of all items */ display: flex; transition: transform 0.5s ease-in-out; }
In this CSS, we set the transition property for the transform property. This enables a smooth transition effect when the transform property changes, creating a sliding animation.
5.2. Applying Transition Effects to Slides
To make the animation smoother, we’ll update the moveLeft and moveRight functions in your script.js file:
javascript // Function to move the carousel left function moveLeft() { if (currentPosition < 0) { currentPosition += 100; carouselContainer.css('transform', `translateX(${currentPosition}%)`); } } // Function to move the carousel right function moveRight() { if (currentPosition > -100 * (carouselItems.length - 3)) { currentPosition -= 100; carouselContainer.css('transform', `translateX(${currentPosition}%)`); } }
By updating the transform property with the new currentPosition, the CSS transition takes care of the smooth animation between slides.
With these changes, your carousel now features sleek sliding animations when navigating through items.
Conclusion
Creating sliders and carousels using jQuery can significantly enhance your website’s user experience by showcasing content in an engaging and visually appealing manner. By following the steps outlined in this tutorial, you’ve learned how to build a basic image slider and transform it into a carousel with navigation and animation effects. Remember that customization possibilities are vast, allowing you to tailor your sliders and carousels to match your website’s design and functionality requirements.
As you continue to explore jQuery and front-end development, don’t hesitate to experiment with various techniques and features to further refine your sliders and carousels. By incorporating these dynamic elements into your web projects, you can captivate your audience and deliver an immersive browsing experience that leaves a lasting impression.
Table of Contents
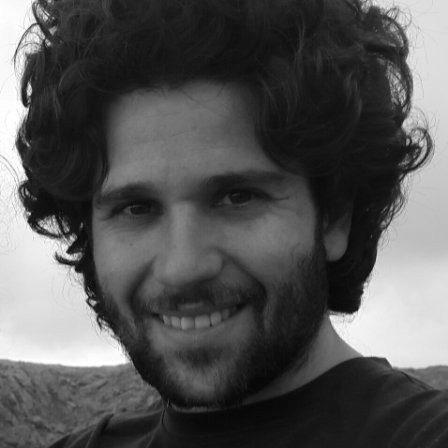
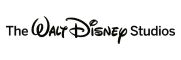