Creating Custom Animations with jQuery
In the dynamic world of web development, animations play a crucial role in enhancing user experience and engagement. They breathe life into static interfaces, making them more interactive and visually appealing. While there are various libraries and frameworks available for creating animations, jQuery remains a popular choice due to its simplicity and versatility. In this tutorial, we’ll delve into the art of creating custom animations with jQuery, exploring various techniques, code samples, and real-world examples.
Table of Contents
1. Why jQuery for Animations?
Before diving into the intricacies of creating custom animations, let’s briefly discuss why jQuery is a valuable tool for this purpose. jQuery, a fast and lightweight JavaScript library, simplifies HTML document traversal and manipulation, event handling, and animation. It provides a user-friendly syntax that abstracts complex JavaScript functions, making it accessible for both beginners and experienced developers.
2. Getting Started: Including jQuery
To begin using jQuery for animations, you need to include it in your HTML document. You can download the jQuery library and host it on your server, or you can use a content delivery network (CDN) to link to it directly. Here’s an example of how you can include jQuery using a CDN:
html <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>jQuery Animations</title> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> </head> <body> <!-- Your HTML content here --> </body> </html>
With jQuery properly included, you’re now ready to dive into creating custom animations.
3. Basic Animations with jQuery
3.1. Fading Elements
One of the simplest animations you can create using jQuery is a fading effect. This effect gradually changes the opacity of an element, making it appear or disappear smoothly. The following code demonstrates a basic fading animation:
javascript $(document).ready(function() { $("#fadeInButton").click(function() { $("#targetElement").fadeIn(); }); $("#fadeOutButton").click(function() { $("#targetElement").fadeOut(); }); });
In this example, when a button with the ID fadeInButton is clicked, the element with the ID targetElement will fade in. Similarly, clicking the button with the ID fadeOutButton will trigger a fade-out effect for the same element.
3.2. Sliding Elements
Slide animations are another way to introduce movement to your website. You can slide elements up, down, left, or right using jQuery. Here’s a simple example:
javascript $(document).ready(function() { $("#slideUpButton").click(function() { $("#targetElement").slideUp(); }); $("#slideDownButton").click(function() { $("#targetElement").slideDown(); }); });
In this code, clicking the slideUpButton will slide the targetElement upwards, while clicking the slideDownButton will slide it back down.
4. Advanced Animations with Custom Properties
4.1. Animating Custom Properties
jQuery allows you to animate not only standard CSS properties like opacity and width but also custom properties. This opens up a world of possibilities for creating unique and captivating animations. To animate custom properties, you can use the animate() function. Let’s say you want to create a pulsating effect by changing an element’s size and color alternately:
javascript $(document).ready(function() { function pulseAnimation() { $("#pulsatingElement").animate({ width: "200px", height: "200px", backgroundColor: "#FF5733" }, 1000, function() { $(this).animate({ width: "150px", height: "150px", backgroundColor: "#337ab7" }, 1000, pulseAnimation); }); } pulseAnimation(); });
In this example, the pulsatingElement gradually changes its width, height, and background color in a pulsating manner.
4.2. Animating Multiple Properties
Animating multiple properties simultaneously can create more complex and visually appealing animations. Consider an example where you want to animate the rotation and opacity of an element simultaneously:
javascript $(document).ready(function() { $("#animateButton").click(function() { $("#animatedElement").animate({ rotate: "180deg", opacity: 0.5 }, 1000); }); });
In this code, clicking the animateButton will rotate the animatedElement by 180 degrees and reduce its opacity to 50% over a duration of 1000 milliseconds.
5. Chaining Animations for a Smooth Flow
jQuery allows you to chain animations, creating a smooth flow of transitions between different states. This technique is particularly useful when you want to create a sequence of animations. Here’s an example of chaining animations:
javascript $(document).ready(function() { $("#startButton").click(function() { $("#chainedElement") .slideUp() .slideDown() .fadeOut() .fadeIn(); }); });
In this code, clicking the startButton will trigger a sequence of animations: sliding up, sliding down, fading out, and finally fading in for the chainedElement.
6. Real-World Example: Interactive Navigation Menu
Let’s put our newfound knowledge to practical use by creating an interactive navigation menu that responds to user interaction:
html <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Interactive Menu Example</title> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> <style> /* Add your CSS styles here */ </style> </head> <body> <nav> <ul> <li><a href="#">Home</a></li> <li><a href="#">Services</a></li> <li><a href="#">Portfolio</a></li> <li><a href="#">Contact</a></li> </ul> </nav> <script> $(document).ready(function() { $("nav li").hover(function() { $(this).find("a").animate({ fontSize: "18px" }, 300); }, function() { $(this).find("a").animate({ fontSize: "16px" }, 300); }); }); </script> </body> </html>
In this example, when a user hovers over a navigation menu item, the font size of the associated link smoothly increases, providing a visually appealing and interactive experience.
Conclusion
jQuery remains a powerful tool for creating custom animations that add interactivity and engagement to your web projects. From simple fade and slide effects to complex animations with custom properties, jQuery’s flexibility empowers developers to create captivating user experiences. By incorporating these techniques into your toolkit, you’ll be well-equipped to enhance your websites with eye-catching animations that leave a lasting impression on your users. So, why wait? Start experimenting with jQuery animations and take your web development skills to the next level!
Table of Contents
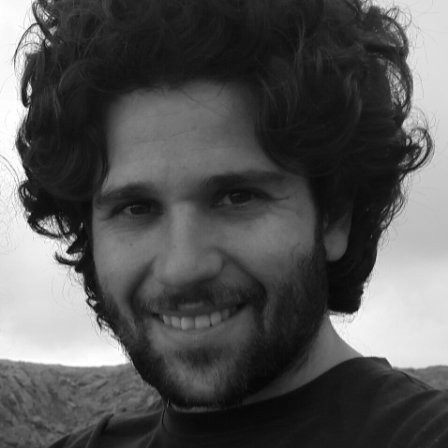
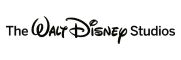