Creating Interactive Maps with jQuery and Google Maps API
In today’s digital age, interactive maps have become an essential element of many websites. Whether you run a business, manage a travel blog, or want to display geospatial data, interactive maps can significantly enhance user experience and engagement. In this blog, we will explore how to create captivating interactive maps using jQuery and the powerful Google Maps API.
Table of Contents
Before we dive into the technical details, let’s understand the technologies we’ll be using:
jQuery
jQuery is a fast, small, and feature-rich JavaScript library that simplifies HTML document traversing, event handling, and animation. It’s widely used for web development due to its ease of use and cross-browser compatibility.
Google Maps API
The Google Maps API provides developers with a versatile platform to integrate Google Maps into their websites. With a plethora of features and functionalities, it enables the creation of custom maps with dynamic data.
1. Setting Up the Environment
To get started, create a new HTML file and include the necessary dependencies:
html <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Interactive Map with jQuery and Google Maps API</title> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> <script src="https://maps.googleapis.com/maps/api/js?key=YOUR_API_KEY&callback=initMap" async defer></script> <style> #map { height: 500px; width: 100%; } </style> </head> <body> <h1>Interactive Map with jQuery and Google Maps API</h1> <div id="map"></div> </body> </html>
Make sure to replace YOUR_API_KEY with your actual Google Maps API key. If you don’t have one, you can obtain it by creating a project in the Google Cloud Console and enabling the Maps JavaScript API.
2. Initializing the Map
Next, let’s initialize the map using jQuery and the Google Maps API:
html <script> function initMap() { const mapOptions = { center: { lat: 40.7128, lng: -74.0060 }, zoom: 12 }; const map = new google.maps.Map(document.getElementById('map'), mapOptions); } </script>
In this example, we set the initial center of the map to New York City (latitude: 40.7128, longitude: -74.0060) and zoom level to 12. Feel free to adjust these values to match your desired location and zoom level.
3. Adding Markers to the Map
Markers are essential for indicating specific locations on the map. Let’s add some markers to our interactive map:
html <script> function initMap() { const mapOptions = { center: { lat: 40.7128, lng: -74.0060 }, zoom: 12 }; const map = new google.maps.Map(document.getElementById('map'), mapOptions); // Add markers to the map const markers = [ { lat: 40.7128, lng: -74.0060, title: 'New York City' }, { lat: 34.0522, lng: -118.2437, title: 'Los Angeles' }, { lat: 41.8781, lng: -87.6298, title: 'Chicago' }, // Add more markers as needed ]; markers.forEach(markerData => { const marker = new google.maps.Marker({ position: { lat: markerData.lat, lng: markerData.lng }, map: map, title: markerData.title }); }); } </script>
Now, the map will display markers for New York City, Los Angeles, and Chicago. You can add more markers by extending the markers array with additional locations.
4. Customizing Markers
To make our markers more visually appealing and distinctive, we can customize their appearance. Let’s change the marker icon and add animations on mouseover:
html <script> function initMap() { // Same initialization code as before const map = new google.maps.Map(document.getElementById('map'), mapOptions); // Same marker code as before markers.forEach(markerData => { const marker = new google.maps.Marker({ position: { lat: markerData.lat, lng: markerData.lng }, map: map, title: markerData.title, icon: 'path/to/custom/icon.png' // Replace with your custom icon path }); // Add animation on mouseover marker.addListener('mouseover', () => { marker.setAnimation(google.maps.Animation.BOUNCE); }); // Remove animation on mouseout marker.addListener('mouseout', () => { marker.setAnimation(null); }); }); } </script>
Now, the markers will have custom icons, and they will bounce with animation when the user hovers over them. You can create or use your own marker icons and replace the ‘path/to/custom/icon.png’ with the actual path.
5. Info Windows for Markers
Info windows provide additional information about a specific location when a user clicks on its marker. Let’s add info windows to our markers:
html <script> function initMap() { // Same initialization code as before const map = new google.maps.Map(document.getElementById('map'), mapOptions); // Same marker code as before markers.forEach(markerData => { const marker = new google.maps.Marker({ position: { lat: markerData.lat, lng: markerData.lng }, map: map, title: markerData.title, icon: 'path/to/custom/icon.png' }); // Add animation on mouseover // Remove animation on mouseout // Add info window on marker click const infoWindow = new google.maps.InfoWindow({ content: `<h3>${markerData.title}</h3> <p>Latitude: ${markerData.lat}, Longitude: ${markerData.lng}</p>` }); marker.addListener('click', () => { infoWindow.open(map, marker); }); }); } </script>
Now, when a user clicks on a marker, an info window will pop up, displaying the location’s name, latitude, and longitude. You can customize the content of the info window to include any relevant information.
6. Adding Interactivity to the Map
Apart from markers and info windows, we can make our map more interactive by adding additional features. Let’s explore some possibilities:
6.1. Adding Custom Controls
Custom controls allow you to add buttons or elements to the map to trigger specific actions. For example, let’s add a control that centers the map on the user’s current location:
html <script> function initMap() { // Same initialization code as before const map = new google.maps.Map(document.getElementById('map'), mapOptions); // Same marker code as before // Same info window code as before // Add custom control to center the map on the user's current location const centerControlDiv = document.createElement('div'); const centerControl = new CenterControl(centerControlDiv, map); centerControlDiv.index = 1; map.controls[google.maps.ControlPosition.TOP_RIGHT].push(centerControlDiv); function CenterControl(controlDiv, map) { const controlUI = document.createElement('div'); controlUI.style.backgroundColor = '#fff'; controlUI.style.border = '2px solid #fff'; controlUI.style.borderRadius = '3px'; controlUI.style.boxShadow = '0 2px 6px rgba(0,0,0,.3)'; controlUI.style.cursor = 'pointer'; controlUI.style.marginBottom = '10px'; controlUI.style.textAlign = 'center'; controlUI.title = 'Click to center the map on your current location'; controlDiv.appendChild(controlUI); const controlText = document.createElement('div'); controlText.style.color = 'rgb(25,25,25)'; controlText.style.fontFamily = 'Roboto,Arial,sans-serif'; controlText.style.fontSize = '16px'; controlText.style.lineHeight = '38px'; controlText.style.paddingLeft = '5px'; controlText.style.paddingRight = '5px'; controlText.innerHTML = 'Center Map'; controlUI.appendChild(controlText); controlUI.addEventListener('click', () => { if (navigator.geolocation) { navigator.geolocation.getCurrentPosition(position => { const userLatLng = { lat: position.coords.latitude, lng: position.coords.longitude }; map.setCenter(userLatLng); }); } }); } } </script>
This code will add a custom control to the top-right corner of the map. When the “Center Map” button is clicked, the map will center on the user’s current location if geolocation is enabled in the user’s browser.
6.2. Drawing Shapes on the Map
The Google Maps API allows us to draw shapes like polygons, circles, and polylines on the map. Let’s add a polygon to highlight a specific area:
html <script> function initMap() { // Same initialization code as before const map = new google.maps.Map(document.getElementById('map'), mapOptions); // Same marker code as before // Same info window code as before // Same custom control code as before // Draw a polygon to highlight an area const polygonCoords = [ { lat: 40.748817, lng: -73.985428 }, { lat: 40.7057, lng: -74.0140 }, { lat: 40.7176, lng: -74.0129 }, // Add more coordinates to complete the polygon ]; const highlightArea = new google.maps.Polygon({ paths: polygonCoords, strokeColor: '#FF0000', strokeOpacity: 0.8, strokeWeight: 2, fillColor: '#FF0000', fillOpacity: 0.35 }); highlightArea.setMap(map); } </script>
Here, we draw a polygon to highlight a specific area in New York City. The polygon’s coordinates are specified in the polygonCoords array. You can add more coordinates to customize the shape and size of the polygon.
Conclusion
Interactive maps play a vital role in modern web design, providing valuable information and enhancing the user experience. By harnessing the power of jQuery and the Google Maps API, you can create dynamic and visually appealing maps for your website. From adding markers and info windows to incorporating custom controls and drawing shapes, the possibilities are limitless. Start experimenting with different features, and watch your website come to life with interactive maps!
In this blog, we’ve covered the basic steps to create an interactive map with jQuery and the Google Maps API. However, this is just the tip of the iceberg. The Google Maps API offers a wide range of features like geocoding, directions, Street View, and much more. So, feel free to explore the official documentation and take your interactive maps to the next level! Happy mapping!
Table of Contents
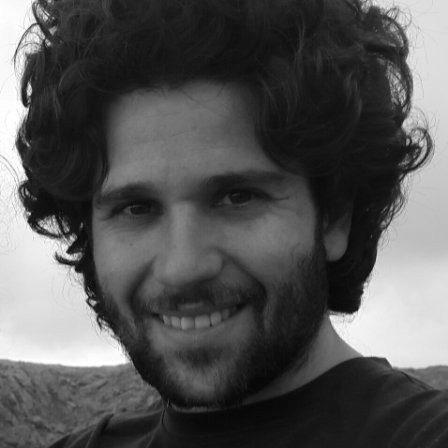
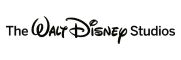