Creating Interactive Maps with jQuery Maps
Interactive maps have become a ubiquitous feature in modern web applications. Whether you’re building a location-based service, a travel website, or a data visualization tool, interactive maps can enhance user engagement and provide valuable information. One popular library for achieving this is jQuery Maps. In this comprehensive guide, we’ll dive into the world of interactive maps with jQuery Maps, exploring its features, implementation, and customization options.
Table of Contents
1. Why jQuery Maps?
Before we delve into the technical details, let’s briefly discuss why you might choose jQuery Maps for your interactive mapping needs.
1.1. Accessibility
jQuery Maps prioritizes accessibility, making it easier for all users, including those with disabilities, to interact with your maps. This ensures a wider audience can benefit from your maps.
1.2. Cross-Browser Compatibility
One of jQuery Maps’ strengths is its cross-browser compatibility. It works seamlessly on various browsers, eliminating the headaches of dealing with compatibility issues.
1.3. Extensive Plugin Ecosystem
jQuery Maps boasts a vast collection of plugins that can add a myriad of features to your maps, from geolocation to custom markers, heatmaps, and more. These plugins can save you significant development time.
2. Getting Started
Now, let’s dive into creating interactive maps with jQuery Maps. Follow these steps to get started:
2.1. Setting Up Your Environment
First, ensure you have the necessary tools and libraries. You’ll need jQuery and jQuery Maps. Download and include them in your project:
html <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> <script src="https://cdn.jsdelivr.net/npm/jquery-maps@1.2.3/dist/jquery.maps.min.js"></script>
2.2. HTML Structure
Set up your HTML structure for the map container:
html <div id="map"></div>
2.3. CSS Styling
Apply CSS to style your map container:
css #map { width: 100%; height: 500px; }
2.4. JavaScript Initialization
Initialize the map in your JavaScript file:
javascript $(document).ready(function() { $('#map').maps(); });
Congratulations! You now have a basic interactive map set up on your website.
3. Adding Markers
Interactive maps become more useful when you can add markers to specific locations. Let’s learn how to do this using jQuery Maps.
3.1. Marker Data
Define marker data as an array of objects, each containing latitude and longitude coordinates:
javascript var markers = [ { lat: 40.7128, lng: -74.0060, title: 'New York City' }, { lat: 34.0522, lng: -118.2437, title: 'Los Angeles' }, { lat: 41.8781, lng: -87.6298, title: 'Chicago' } ];
3.2. Adding Markers to the Map
Use the addMarkers method to add markers to your map:
javascript $('#map').maps('addMarkers', markers);
You can customize the markers further by specifying icons, titles, and even click events.
4. Interactivity and Event Handling
jQuery Maps provides extensive support for handling user interactions and events on your map.
4.1. Click Events
Attach a click event to a marker:
javascript $('#map').on('markerClick', function(event, marker) { alert('You clicked on ' + marker.title); });
4.2. Info Windows
Display information when a marker is clicked:
javascript $('#map').on('markerClick', function(event, marker) { var infoWindow = new google.maps.InfoWindow({ content: 'You clicked on ' + marker.title }); infoWindow.open(marker.map, marker); });
4.3. Custom Controls
Add custom controls to your map for enhanced interactivity:
javascript $('#map').maps('addControl', { position: 'top-right', content: '<button id="custom-button">Custom Button</button>' }); $('#custom-button').click(function() { // Custom button click logic here });
5. Geolocation and User Interaction
Enabling geolocation on your map allows users to find their own location. Here’s how to do it:
javascript $('#map').maps('getUserLocation', function(location) { if (location) { alert('Your location is: ' + location.latitude + ', ' + location.longitude); } else { alert('Geolocation not supported or denied by the user.'); } });
6. Customizing Your Map
jQuery Maps offers extensive customization options to make your maps match your website’s aesthetics.
6.1. Styling
Apply custom styles to your map using JSON:
javascript var mapStyles = [ { featureType: 'water', stylers: [ { color: '#19a0d8' } ] }, { featureType: 'road.highway', stylers: [ { saturation: -100 }, { lightness: 50 } ] } ]; $('#map').maps('setStyles', mapStyles);
6.2. Custom Icons
Use custom icons for your markers:
javascript var customIcon = { url: 'path/to/custom-icon.png', size: new google.maps.Size(50, 50), origin: new google.maps.Point(0, 0), anchor: new google.maps.Point(25, 50) }; $('#map').maps('setMarkerIcon', customIcon);
7. Integrating Data and Visualizations
Interactive maps are often used to visualize data. jQuery Maps makes it easy to integrate data and create stunning visualizations.
7.1. Heatmaps
Display data as a heatmap:
javascript var heatmapData = [ new google.maps.LatLng(40.7128, -74.0060), new google.maps.LatLng(34.0522, -118.2437), // Add more data points here ]; $('#map').maps('addHeatmap', heatmapData);
7.2. GeoJSON Integration
Load GeoJSON data onto your map:
javascript $.getJSON('path/to/data.json', function(data) { $('#map').maps('addGeoJSON', data); });
Conclusion
jQuery Maps is a powerful tool for creating interactive maps that can greatly enhance your web applications. Whether you need to display locations, visualize data, or provide geolocation services, jQuery Maps offers a wide range of features and customization options to meet your needs. Start exploring and experimenting with this library to create engaging and informative maps that captivate your audience.
In this guide, we’ve covered the basics of setting up a map, adding markers, handling events, enabling geolocation, customizing the map’s appearance, and integrating data. Armed with this knowledge, you’re well on your way to creating stunning interactive maps that will elevate your web projects to the next level. So, get started with jQuery Maps and unlock the world of interactive mapping possibilities for your web applications. Happy mapping!
Table of Contents
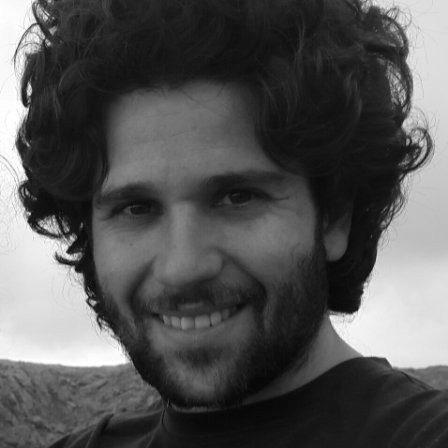
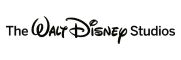