Creating Interactive Menus with jQuery
In today’s fast-paced digital world, user experience plays a pivotal role in the success of any website. One of the key aspects of enhancing user experience is the navigation menu. Traditional static menus have now evolved into dynamic, interactive menus that not only look aesthetically pleasing but also provide a seamless browsing experience. With jQuery, a popular JavaScript library, you can easily create interactive menus that engage users and make navigation a breeze.
Table of Contents
In this tutorial, we’ll explore the process of creating interactive menus using jQuery. We’ll cover everything from the basics to more advanced techniques, along with code samples to help you implement these features on your own website. Let’s dive in!
1. Introduction to Interactive Menus
1.1 Why Interactive Menus Matter
Interactive menus play a significant role in improving user engagement and navigation on your website. They allow users to explore different sections of your site effortlessly, leading to a more satisfying browsing experience. With interactive menus, users can find what they’re looking for quickly, boosting your website’s usability and ultimately keeping visitors on your site for longer.
1.2 Understanding jQuery
jQuery is a powerful JavaScript library that simplifies the process of creating interactive web elements. It provides a wide range of features and functions that enable developers to manipulate HTML documents, handle events, create animations, and much more, with less code than traditional JavaScript.
2. Getting Started
2.1 Setting Up Your Project
Before you begin, create a new directory for your project files. Organizing your files neatly from the start will save you time and effort later on.
2.2 Linking jQuery to Your Project
To start using jQuery, you need to include it in your HTML document. You can either download jQuery and host it locally or include it from a content delivery network (CDN). Place the following code within the <head> section of your HTML file:
html <head> <!-- Other meta tags and stylesheets --> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> <script src="your-script.js"></script> </head>
Replace “your-script.js” with the actual path to your JavaScript file where you’ll be writing the jQuery code.
3. Building the Basic Menu
3.1 Creating the HTML Structure
Start by creating the basic structure of your menu using HTML. For this example, let’s create a simple horizontal menu:
html <nav class="menu"> <ul> <li><a href="#">Home</a></li> <li><a href="#">About</a></li> <li><a href="#">Services</a></li> <li><a href="#">Contact</a></li> </ul> </nav>
3.2 Styling the Menu with CSS
Enhance the visual appeal of your menu using CSS styles. You can customize the styling according to your website’s design:
css .menu { background-color: #333; padding: 10px; } .menu ul { list-style: none; margin: 0; padding: 0; display: flex; } .menu li { margin-right: 20px; } .menu a { text-decoration: none; color: #fff; font-weight: bold; transition: color 0.3s; } .menu a:hover { color: #ff6600; }
3.3 Adding jQuery for Interactivity
Now comes the exciting part—adding interactivity to your menu using jQuery. Open your JavaScript file (e.g., “your-script.js”) and write the following code:
javascript $(document).ready(function() { $(".menu li").click(function() { // Remove the 'active' class from all menu items $(".menu li").removeClass("active"); // Add the 'active' class to the clicked menu item $(this).addClass("active"); }); });
In this code, we use the .click() function to handle clicks on menu items. When a menu item is clicked, we remove the active class from all other items and add it to the clicked item. This will visually highlight the active menu item.
Congratulations! You’ve now created a basic interactive menu using jQuery. Users will see the active menu item change color when they click on it.
4. Implementing Dropdown Menus
4.1 Structuring Dropdown Elements
To create dropdown menus within your interactive menu, you’ll need to modify the HTML structure. Let’s add a dropdown under the “Services” menu item:
html <nav class="menu"> <ul> <li><a href="#">Home</a></li> <li><a href="#">About</a></li> <li class="dropdown"> <a href="#">Services</a> <ul class="dropdown-content"> <li><a href="#">Web Design</a></li> <li><a href="#">Development</a></li> <li><a href="#">Digital Marketing</a></li> </ul> </li> <li><a href="#">Contact</a></li> </ul> </nav>
4.2 Writing jQuery for Dropdown Functionality
Update your JavaScript code to make the dropdowns functional:
javascript $(document).ready(function() { // ... Previous code ... $(".dropdown").hover(function() { // Show the dropdown content on hover $(this).find(".dropdown-content").slideDown(); }, function() { // Hide the dropdown content when not hovering $(this).find(".dropdown-content").slideUp(); }); });
With this code, the dropdown content will slide down when a user hovers over a menu item and slide up when the mouse leaves the area.
4.3 Styling Dropdown Menus
To style the dropdown menus, you can use CSS similar to the following:
css .dropdown-content { display: none; position: absolute; background-color: #fff; box-shadow: 0px 8px 16px 0px rgba(0,0,0,0.2); } .dropdown-content li { padding: 10px; } .dropdown-content a { color: #333; text-decoration: none; transition: background-color 0.3s; } .dropdown-content a:hover { background-color: #f4f4f4; }
5. Adding Smooth Animations
5.1 Enhancing User Experience with Animations
Animations can greatly enhance the user experience by making your interactive menus feel responsive and visually appealing. Let’s add some smooth animations to our menu interactions.
5.2 Implementing Fade and Slide Effects
Update your JavaScript code to include animations:
javascript $(document).ready(function() { // ... Previous code ... $(".menu li").click(function() { // ... Previous code ... // Scroll smoothly to the clicked menu item $("html, body").animate({ scrollTop: $($(this).find("a").attr("href")).offset().top }, 800); }); $(".dropdown").hover(function() { // ... Previous code ... // Add a smooth fade-in effect to the dropdown content $(this).find(".dropdown-content").fadeIn(); }, function() { // ... Previous code ... // Add a smooth fade-out effect to the dropdown content $(this).find(".dropdown-content").fadeOut(); }); });
By adding .fadeIn() and .fadeOut() functions, you provide a visually appealing transition when showing and hiding the dropdown content. Similarly, the .animate() function smoothly scrolls the page to the target section when a menu item is clicked.
5.3 Fine-tuning Animation Speeds
Feel free to adjust the animation speeds to your preference by changing the values passed to the animation functions. Experimenting with different durations can help you find the right balance between a smooth experience and a responsive feel.
6. Active Menu Highlighting
6.1 Indicating the Current Page
When users navigate through your website, it’s essential to highlight the active menu item to provide them with a clear sense of their location. Let’s implement this feature using jQuery.
6.2 Writing jQuery for Active Menu Highlighting
Update your JavaScript code to automatically highlight the menu item corresponding to the current page:
javascript $(document).ready(function() { // ... Previous code ... // Highlight the appropriate menu item based on the current page var currentPage = window.location.pathname.split("/").pop(); $(".menu a[href='" + currentPage + "']").parent().addClass("active"); });
In this code, we extract the current page’s filename from the URL and then add the active class to the menu item with a corresponding link.
6.3 Styling the Active Menu Item
Finally, apply some CSS to style the active menu item:
css .menu li.active a { color: #ff6600; border-bottom: 2px solid #ff6600; }
This CSS rule ensures that the active menu item is visually distinct, making it easy for users to identify their current location on the website.
7. Mobile-Friendly Navigation
7.1 Importance of Responsive Menus
With the increasing use of mobile devices, having a responsive menu is crucial for providing a seamless experience across various screen sizes. Let’s adapt our interactive menu for mobile devices by implementing a hamburger menu.
7.2 Creating a Hamburger Menu
Modify your HTML structure to accommodate the mobile-friendly navigation:
html <nav class="menu"> <div class="mobile-menu-toggle">☰</div> <ul> <!-- ... Menu items ... --> </ul> </nav>
7.3 Adapting Dropdowns for Mobile
To ensure a smooth experience on mobile devices, adjust your JavaScript code to toggle the menu on mobile:
javascript $(document).ready(function() { // ... Previous code ... $(".mobile-menu-toggle").click(function() { $(".menu ul").toggleClass("show"); }); });
Then, add the necessary CSS to make the menu items visible when the mobile menu is toggled:
css .menu ul.show { display: block; }
8. Advanced Menu Effects
8.1 Creating Mega Menus
Mega menus offer an even more comprehensive way of displaying content and options to users. By combining multiple dropdowns and visual elements, you can create engaging mega menus using jQuery and CSS.
8.2 Adding Search Functionality
Enhance user experience by including a search bar within your interactive menu. You can use jQuery to toggle the search bar’s visibility and smoothly animate its appearance.
8.3 Implementing Keyboard Navigation
For users who prefer keyboard navigation, consider implementing keyboard shortcuts to enhance accessibility. Using jQuery, you can capture keyboard events and navigate through the menu items seamlessly.
Conclusion
By incorporating jQuery into your web development toolkit, you can take your website’s navigation menus to the next level. Interactive menus not only improve user experience but also showcase your commitment to providing a modern and engaging platform for your visitors. From basic interactivity to advanced effects, jQuery empowers you to create menus that captivate and guide users through your website effortlessly. Experiment with the techniques discussed in this tutorial, and tailor them to suit your website’s unique design and functionality. With these skills under your belt, you’re well on your way to creating dynamic and user-friendly menus that leave a lasting impression.
Table of Contents
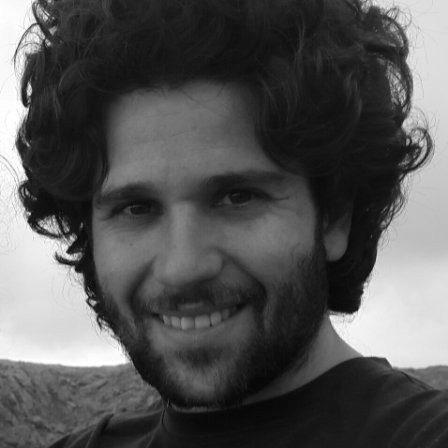
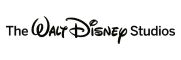