Creating Interactive Timelines with jQuery Timeline
In today’s digital age, presenting information in an engaging and interactive way is crucial for capturing your audience’s attention. Timelines have been a tried-and-true method of displaying chronological data, and with the help of jQuery Timeline, you can take your timelines to the next level. In this blog, we will explore the ins and outs of creating interactive timelines using jQuery Timeline, complete with code samples and step-by-step instructions.
Table of Contents
1. What is jQuery Timeline?
jQuery Timeline is a versatile JavaScript library that simplifies the process of creating interactive timelines on your website. Whether you want to showcase historical events, project milestones, or any chronological data, jQuery Timeline provides you with the tools to do so efficiently and beautifully. It’s a valuable asset for web developers and designers looking to enhance user engagement through compelling visual storytelling.
2. Getting Started with jQuery Timeline
Step 1: Installation
Before we dive into creating interactive timelines, let’s set up jQuery Timeline. You can include it in your project by downloading the library from the official website or linking it via a content delivery network (CDN).
Here’s how you can include jQuery Timeline via CDN in your HTML file:
html <!DOCTYPE html> <html> <head> <!-- Include jQuery --> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> <!-- Include jQuery Timeline CSS --> <link rel="stylesheet" type="text/css" href="path-to-your-css/jquery.timeline.css"> <!-- Include jQuery Timeline JS --> <script src="path-to-your-js/jquery.timeline.js"></script> </head> <body> <!-- Your timeline content goes here --> </body> </html>
Step 2: HTML Structure
To create an interactive timeline, you need to define the structure in your HTML. You’ll typically have a container element for the timeline and individual items for each event. Here’s a basic example:
html <div class="timeline"> <div class="timeline-item"> <div class="timeline-content"> <h2>Event 1</h2> <p>Description of Event 1.</p> </div> <div class="timeline-badge"> <!-- Badge content, e.g., date or icon --> </div> </div> <!-- Add more timeline items as needed --> </div>
Step 3: Initialize jQuery Timeline
After defining the HTML structure, it’s time to initialize jQuery Timeline. Use the following JavaScript code to make your timeline interactive:
javascript $(document).ready(function() { $('.timeline').timeline(); });
Now, you have a basic interactive timeline set up on your website.
3. Customizing Your Timeline
jQuery Timeline offers a wide range of customization options to match your website’s style and the content you want to present. Here are some key customization features:
3.1. Styling
You can easily modify the appearance of your timeline using CSS. jQuery Timeline provides classes and IDs for different elements, making it straightforward to apply your own styles. Here’s an example of how you can change the background color of timeline items:
css .timeline-item { background-color: #f2f2f2; }
3.2. Timeline Orientation
By default, jQuery Timeline displays events in a vertical orientation. However, you can switch to a horizontal layout if it better suits your design. Use the horizontal option when initializing the timeline:
javascript $('.timeline').timeline({ orientation: 'horizontal' });
3.3. Date Format
You can customize the date format displayed in timeline badges. For example, if you want to display dates in the “Month Year” format, use the dateFormat option:
javascript $('.timeline').timeline({ dateFormat: 'MM yyyy' });
3.4. Icons and Badges
Enhance the visual appeal of your timeline by adding icons or custom badges to timeline items. This can help users quickly identify the type or significance of each event.
Here’s an example of how to include Font Awesome icons in your timeline badges:
html <div class="timeline-badge"> <i class="fas fa-calendar"></i> </div>
3.5. Event Interaction
jQuery Timeline allows you to incorporate user interaction with your timeline events. You can add click handlers to each timeline item to display additional information or links related to the event:
javascript $('.timeline-item').on('click', function() { // Handle click event for the timeline item // Display more information, open a modal, etc. });
4. Advanced Features
Beyond the basics, jQuery Timeline offers advanced features that can take your interactive timelines to the next level:
4.1. Timeline Filtering
If your timeline contains a large number of events, you can implement filtering to allow users to focus on specific categories or time periods. This is especially useful for complex timelines with diverse content.
Here’s how you can add filtering options to your timeline:
javascript $('.timeline').timeline({ filters: { 'Category 1': '.category-1', 'Category 2': '.category-2', // Add more categories and corresponding CSS classes } });
4.2. Timeline Animation
You can add animations to your timeline to make it even more engaging. jQuery Timeline supports various animation effects, such as fading in timeline items as the user scrolls down the page.
To enable animations, simply include the jquery.timeline.animations.js plugin and customize the animation settings:
javascript $('.timeline').timeline({ animation: { effect: 'fadeIn', // Choose from 'fadeIn', 'slideDown', 'slideLeft', and more duration: 400, // Animation duration in milliseconds trigger: 'inview' // Specify when the animation triggers ('inview' or 'scroll') } });
4.3. Responsive Design
In today’s mobile-first world, ensuring your timeline looks and functions well on different screen sizes is essential. jQuery Timeline is responsive by default, but you can fine-tune its behavior using CSS media queries and options like responsive and responsiveFallback.
javascript $('.timeline').timeline({ responsive: true, responsiveFallback: 600 // Adjust the breakpoint as needed });
5. Real-World Examples
Let’s explore a couple of real-world examples where jQuery Timeline can be a valuable addition:
5.1. Company History Timeline
Many businesses use timelines to showcase their company’s growth and milestones. With jQuery Timeline, you can create an engaging company history page that highlights key achievements, product launches, and important events. Add images and descriptions to make it visually appealing and informative.
html <div class="timeline"> <div class="timeline-item"> <div class="timeline-content"> <h2>Founded</h2> <p>Our company was founded with a vision to revolutionize...</p> </div> <div class="timeline-badge"> <i class="fas fa-calendar"></i> 2000 </div> </div> <!-- Add more company history events --> </div>
5.2. Educational Course Schedule
Educational websites can utilize jQuery Timeline to display course schedules and important dates. Students can easily navigate through semesters or terms, accessing details about each course and its prerequisites.
html <div class="timeline"> <div class="timeline-item"> <div class="timeline-content"> <h2>Fall Semester</h2> <p>Explore our exciting courses for the fall semester...</p> </div> <div class="timeline-badge"> <i class="fas fa-calendar"></i> Sep 2023 </div> </div> <!-- Add more course schedule events --> </div>
Conclusion
Creating interactive timelines with jQuery Timeline can significantly enhance your website’s user experience and engagement. Whether you’re sharing historical events, project milestones, or any chronological data, jQuery Timeline provides the tools and flexibility needed to craft stunning and informative timelines. By following the steps and customization options outlined in this guide, you’ll be well on your way to impressing your website visitors with captivating timelines.
So, why wait? Start implementing jQuery Timeline today and bring your chronological data to life in a visually compelling way.
Remember, a well-crafted timeline not only informs but also captivates and engages your audience, making it an invaluable addition to any website or web application.
Table of Contents
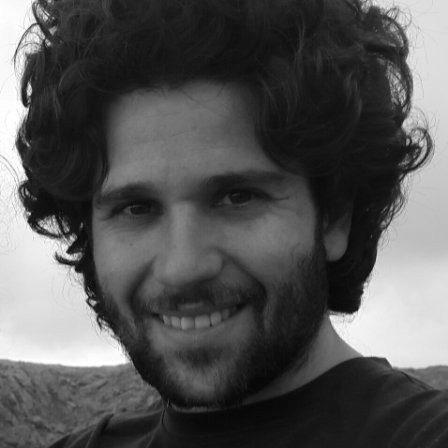
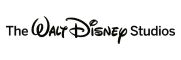