Creating Accordion Menus with jQuery UI Accordion
Accordion menus are a popular choice in web design for organizing and presenting content in a space-saving, user-friendly manner. They allow users to expand and collapse sections of content, making navigation more intuitive and efficient. One of the easiest ways to implement accordion menus in your web projects is by using jQuery UI Accordion. In this comprehensive guide, we’ll walk you through the process of creating accordion menus with jQuery UI Accordion, complete with code samples and examples.
Table of Contents
1. What is jQuery UI Accordion?
jQuery UI is a widely used library that extends jQuery’s capabilities by providing a set of user interface widgets and interactions. Among these widgets, the Accordion is a versatile component for creating collapsible and expandable content sections with ease. jQuery UI Accordion simplifies the process of adding interactive accordion menus to your website or web application.
2. Why Use jQuery UI Accordion?
Before we dive into the implementation, let’s explore why you should consider using jQuery UI Accordion for your accordion menus:
- Ease of Use: jQuery UI Accordion abstracts complex JavaScript and CSS interactions, making it accessible even to developers with limited frontend experience.
- Customization: It allows you to customize the appearance and behavior of your accordion menus with options for animations, icons, and themes.
- Accessibility: jQuery UI Accordion is designed with accessibility in mind, ensuring that your menus are usable by all visitors, including those with disabilities.
- Cross-Browser Compatibility: It offers cross-browser compatibility, so your accordion menus will work consistently across various web browsers.
Now, let’s walk through the steps to create accordion menus using jQuery UI Accordion.
3. Getting Started
3.1. Include jQuery and jQuery UI
To get started, you’ll need to include jQuery and jQuery UI in your project. You can download the libraries and host them on your server or include them from content delivery networks (CDNs). Here’s how you can include them in your HTML file:
html <!DOCTYPE html> <html> <head> <title>Accordion Menu Example</title> <link rel="stylesheet" href="https://code.jquery.com/ui/1.12.1/themes/base/jquery-ui.css"> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> <script src="https://code.jquery.com/ui/1.12.1/jquery-ui.js"></script> </head> <body> <!-- Your content here --> </body> </html>
3.2. Create HTML Structure
Next, you’ll need to create the HTML structure for your accordion menu. Typically, an accordion menu consists of a series of sections (panels) that can be expanded or collapsed. Here’s an example:
html <div id="accordion"> <h3>Section 1</h3> <div> <p>Content for Section 1 goes here.</p> </div> <h3>Section 2</h3> <div> <p>Content for Section 2 goes here.</p> </div> <!-- Add more sections as needed --> </div>
In this example, each <h3> element represents a section header, and the associated <div> contains the content for that section. You can add as many sections as you need.
3.3. Initialize the Accordion
Now that you have the HTML structure in place, it’s time to initialize the accordion using jQuery. Add the following script to your HTML file:
html <script> $(function() { $("#accordion").accordion(); }); </script>
This script initializes the accordion on the #accordion element, which is the container for your accordion menu.
3.4. Customize Your Accordion
While the basic accordion is functional, you can customize it further to suit your design preferences. jQuery UI Accordion provides several options to control its behavior and appearance. Here are some common customizations:
3.4.1. Animation
You can specify an animation effect when expanding or collapsing accordion sections. The available options include ‘slide’, ‘fade’, and ‘scale’. For example, to use the ‘slide’ animation, modify the initialization script like this:
html <script> $(function() { $("#accordion").accordion({ animate: "slide" }); }); </script>
3.4.2. Icons
You can add icons to the section headers to indicate the open and closed states. jQuery UI provides a set of built-in icons, and you can also use custom icons. To use the default icons, modify the initialization script like this:
html <script> $(function() { $("#accordion").accordion({ icons: { header: "ui-icon-plus", activeHeader: "ui-icon-minus" } }); }); </script>
3.4.3. Theme
You can apply a theme to your accordion to match your website’s style. jQuery UI offers several pre-built themes, or you can create a custom theme using the ThemeRoller tool. To apply a theme, add the appropriate CSS class to your accordion container. For example:
html <div id="accordion" class="ui-widget ui-helper-reset"> <!-- Sections go here --> </div>
Make sure to include the theme’s CSS file in your HTML, as mentioned in the “Include jQuery and jQuery UI” step.
3.5. Add Content
With the accordion initialized and customized, you can now add content to your sections. Simply populate the <div> elements within each section with your desired content. You can include text, images, forms, or any HTML elements you need.
Here’s an example with more detailed content:
html <div id="accordion"> <h3>Section 1</h3> <div> <p>Lorem ipsum dolor sit amet, consectetur adipiscing elit.</p> <img src="image1.jpg" alt="Image 1"> </div> <h3>Section 2</h3> <div> <form> <label for="name">Name:</label> <input type="text" id="name" name="name"> <br> <label for="email">Email:</label> <input type="email" id="email" name="email"> </form> </div> </div>
3.6. Final Result
After completing these steps, your accordion menu should be fully functional and styled according to your customizations. Users can click on section headers to expand or collapse the content, providing an efficient way to navigate your website’s information.
4. Advanced Usage
While we’ve covered the basics of creating accordion menus with jQuery UI Accordion, there’s much more you can do with this powerful library. Here are some advanced usage tips:
4.1. Nested Accordions
You can nest accordions within accordions to create complex hierarchical menus. To do this, simply create another accordion inside a section of the parent accordion. Initialize the nested accordion in the appropriate section.
html <div id="accordion"> <h3>Section 1</h3> <div> <!-- Nested accordion --> <div id="nested-accordion"> <h3>Nested Section 1</h3> <div> <p>Content for Nested Section 1.</p> </div> <h3>Nested Section 2</h3> <div> <p>Content for Nested Section 2.</p> </div> </div> </div> <!-- Add more sections as needed --> </div> <script> $(function() { $("#accordion").accordion(); $("#nested-accordion").accordion(); }); </script>
4.2. Programmatic Control
You can control the accordion programmatically by triggering events. For example, to programmatically open the second section, you can use:
javascript $("#accordion").accordion("option", "active", 1);
4.3. Event Handling
jQuery UI Accordion also provides event handling, allowing you to respond to accordion-related events such as section opening and closing. You can use event listeners like this:
javascript $("#accordion").on("accordionactivate", function(event, ui) { // Handle accordion activation here console.log("Accordion section activated:", ui.newHeader.text()); });
Conclusion
jQuery UI Accordion simplifies the process of creating interactive accordion menus for your web projects. With its ease of use, customization options, accessibility features, and cross-browser compatibility, it’s a valuable tool for enhancing user experience. Whether you’re building a personal blog, an e-commerce site, or a web application, accordion menus can help you organize and present content in a user-friendly way. Start incorporating jQuery UI Accordion into your projects and provide users with intuitive navigation and organized content.
In this guide, we’ve covered the basics of creating accordion menus with jQuery UI Accordion, including initialization, customization, and advanced usage. Armed with this knowledge, you can create engaging and interactive accordion menus that enhance the usability of your website. Experiment with different options, styles, and content to tailor your accordion menus to your specific needs and design preferences. Happy coding!
Table of Contents
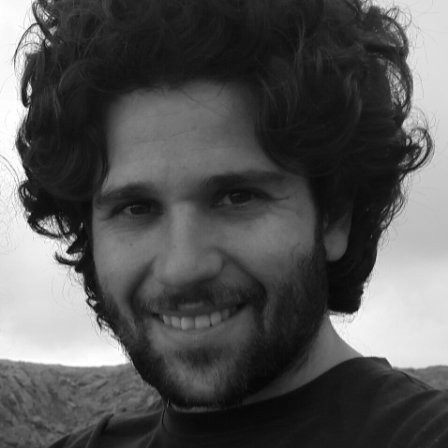
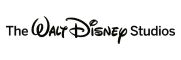