Cross-Browser Compatibility with jQuery: Writing Code That Works Everywhere
In today’s fast-paced digital world, building a website that works seamlessly across different web browsers is essential. One of the challenges developers face is ensuring cross-browser compatibility, as different browsers may interpret HTML, CSS, and JavaScript code differently. jQuery, a popular JavaScript library, can be a powerful tool in achieving cross-browser compatibility and providing a consistent user experience. In this article, we’ll explore the importance of cross-browser compatibility, how jQuery can help, and provide practical code samples to ensure your web code works everywhere.
Table of Contents
1. Why Cross-Browser Compatibility Matters
When you build a website or web application, your target audience may be using various web browsers such as Chrome, Firefox, Safari, Edge, and more. Each browser has its own rendering engine and may interpret your code differently. This can lead to inconsistent layouts, broken functionality, and frustrated users.
Cross-browser compatibility ensures that your website looks and functions as intended across different browsers and devices. It helps you reach a wider audience and provides a better user experience. Without cross-browser compatibility, you risk losing potential customers and damaging your brand’s reputation.
2. The Role of jQuery in Achieving Compatibility
jQuery is a widely used JavaScript library that simplifies HTML document traversal, event handling, animation, and more. It was designed with cross-browser compatibility in mind, making it an excellent choice for developers aiming to create consistent and reliable web experiences.
3. Handling DOM Manipulation
One of the areas where jQuery shines is DOM manipulation. Different browsers may have slight variations in how they handle DOM manipulation tasks. With jQuery, you can write code that targets DOM elements in a consistent and browser-agnostic way. Here’s an example of how jQuery can be used to toggle the visibility of an element:
javascript // Vanilla JavaScript document.getElementById('myButton').addEventListener('click', function() { var element = document.getElementById('myElement'); if (element.style.display === 'none') { element.style.display = 'block'; } else { element.style.display = 'none'; } }); // Using jQuery $('#myButton').click(function() { $('#myElement').toggle(); });
4. Event Handling
Handling events across different browsers can be a headache due to varying event models and behaviors. jQuery abstracts away these differences, allowing you to attach event handlers consistently. Here’s an example of binding a click event handler using jQuery:
javascript // Vanilla JavaScript document.getElementById('myButton').addEventListener('click', function() { alert('Button clicked!'); }); // Using jQuery $('#myButton').click(function() { alert('Button clicked!'); });
5. AJAX and Cross-Browser Requests
Cross-browser compatibility is particularly crucial when making AJAX requests to fetch data from a server. jQuery’s AJAX methods provide a standardized way to perform asynchronous requests, ensuring that your code behaves consistently across different browsers. Here’s a simple example of an AJAX request using jQuery:
javascript $.ajax({ url: 'https://api.example.com/data', method: 'GET', success: function(response) { // Handle the response }, error: function(xhr, status, error) { // Handle errors } });
6. Best Practices for Cross-Browser Compatibility
While jQuery helps simplify cross-browser compatibility, it’s essential to follow best practices to ensure your code works reliably across different environments. Here are some tips to keep in mind:
6.1. Keep jQuery Updated
jQuery’s team actively maintains the library and releases updates to address browser inconsistencies and security issues. Make sure to use the latest version of jQuery to take advantage of these improvements.
6.2. Test Extensively
Thorough testing is key to identifying compatibility issues early in the development process. Test your website on various browsers and devices to catch and address any discrepancies.
6.3. Use Feature Detection
Instead of relying on browser detection, which can be error-prone, use feature detection to check if a specific browser supports a certain feature. Libraries like Modernizr can assist in this process.
6.4. Optimize Performance
Cross-browser compatibility doesn’t just refer to visual consistency; it also involves performance. Optimize your code and minimize unnecessary actions to ensure smooth performance across different browsers.
6.5. Leverage Browser DevTools
Modern browsers offer developer tools that allow you to inspect elements, debug JavaScript, and analyze network requests. Use these tools to identify and fix compatibility issues efficiently.
7. Code Samples for Cross-Browser Compatibility
Here are a few more code samples demonstrating how jQuery can help achieve cross-browser compatibility:
7.1. Smooth Scrolling
Smooth scrolling can enhance user experience, but it’s implemented differently in various browsers. jQuery simplifies the process:
javascript $('a[href^="#"]').click(function(event) { event.preventDefault(); $('html, body').animate({ scrollTop: $($.attr(this, 'href')).offset().top }, 1000); });
7.2. Placeholder Text for Older Browsers
Older browsers don’t support the HTML5 placeholder attribute. jQuery can provide a workaround:
javascript $('[placeholder]').focus(function() { var input = $(this); if (input.val() === input.attr('placeholder')) { input.val(''); input.removeClass('placeholder'); } }).blur(function() { var input = $(this); if (input.val() === '' || input.val() === input.attr('placeholder')) { input.addClass('placeholder'); input.val(input.attr('placeholder')); } }).blur();
7.3. Cross-Browser AJAX
Ensure consistent AJAX behavior with jQuery:
javascript $.ajax({ url: 'https://api.example.com/data', method: 'GET', dataType: 'json', success: function(response) { // Handle the response }, error: function(xhr, status, error) { // Handle errors } });
Conclusion
Cross-browser compatibility is a critical aspect of web development. jQuery, with its focus on simplifying JavaScript tasks and abstracting browser differences, can significantly contribute to a consistent and seamless user experience. By following best practices and utilizing jQuery’s capabilities, you can create web applications that work smoothly across different browsers and devices. Remember to stay updated with jQuery’s releases and embrace testing to ensure your code functions as intended everywhere. With these techniques in your toolkit, you’ll be well-equipped to conquer the challenges of cross-browser compatibility and provide top-notch web experiences for your users.
Table of Contents
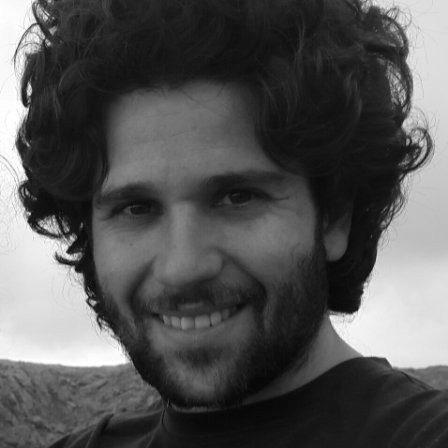
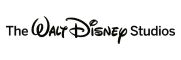