jQuery Mobile: Developing Cross-Platform Mobile Apps
In the ever-evolving world of mobile application development, cross-platform compatibility has become a top priority for developers. Creating separate apps for various platforms can be time-consuming and resource-intensive. Fortunately, jQuery Mobile comes to the rescue as a versatile framework that enables developers to build feature-rich and responsive mobile applications with ease.
1. What is jQuery Mobile?
jQuery Mobile is a touch-optimized web framework that facilitates the creation of cross-platform mobile applications. It’s an extension of the jQuery library, designed to simplify the process of building consistent and responsive apps that work seamlessly across various devices and platforms. Whether you’re targeting iOS, Android, Windows Phone, or other platforms, jQuery Mobile ensures your app looks and feels native on each one.
2. Advantages of jQuery Mobile:
- Cross-Platform Compatibility: One of the biggest advantages of jQuery Mobile is its ability to provide a uniform experience across multiple platforms, reducing development time and costs significantly.
- Responsive Design: jQuery Mobile incorporates responsive design principles, automatically adjusting the layout and components to fit various screen sizes and resolutions.
- Touch-Optimized UI: With built-in touch events, gestures, and smooth transitions, jQuery Mobile makes it easy to create mobile-friendly user interfaces that are intuitive and user-friendly.
- Ease of Use: Developers familiar with jQuery will find it straightforward to work with jQuery Mobile, as it follows a similar syntax and approach.
- Robust Theming: The framework comes with an extensive theming system, allowing developers to customize the app’s appearance and adapt it to their brand easily.
- Lightweight: jQuery Mobile is a lightweight framework that doesn’t put a heavy burden on the app’s performance, ensuring smooth and swift user experiences.
3. Getting Started:
Before diving into the development process, ensure you have the following set up:
3.1 HTML Structure:
The foundation of any jQuery Mobile application is a well-structured HTML document. Begin by setting up the basic structure, including the necessary CSS and JavaScript libraries.
html <!DOCTYPE html> <html> <head> <title>My jQuery Mobile App</title> <link rel="stylesheet" href="https://code.jquery.com/mobile/1.5.0/jquery.mobile-1.5.0.min.css" /> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> <script src="https://code.jquery.com/mobile/1.5.0/jquery.mobile-1.5.0.min.js"></script> </head> <body> <!-- Your app content goes here --> </body> </html>
3.2 Page Structure:
jQuery Mobile operates using a page-based model, where each page represents a distinct section of your application. Create a basic page structure by using the data-role=”page” attribute.
html <div data-role="page" id="home-page"> <div data-role="header"> <h1>Welcome to My App</h1> </div> <div data-role="main" class="ui-content"> <!-- Page content goes here --> </div> <div data-role="footer"> <h4>© 2023 My App</h4> </div> </div>
3.3 Creating Navigation:
Navigation is a crucial aspect of any mobile application. jQuery Mobile provides multiple ways to implement seamless navigation between pages.
3.4 Basic Linking:
Using anchor tags with the href attribute pointing to the target page’s ID will automatically handle the navigation.
html <!-- Inside the main content of a page --> <a href="#second-page">Go to Second Page</a>
3.5 Programmatic Navigation:
You can use JavaScript to navigate between pages programmatically.
html <script> $(document).on("click", "#my-button", function() { $.mobile.changePage("#second-page", { transition: "slide" }); }); </script>
3.6 Buttons and Toolbars:
Mobile applications often use buttons and toolbars to enhance user interactions. jQuery Mobile provides various styles for buttons and toolbars.
1. Standard Button:
Create a basic button using the data-role=”button” attribute.
html <a href="#" data-role="button">Click Me</a>
2. Inline Button:
To create an inline button, add the data-inline=”true” attribute.
html <a href="#" data-role="button" data-inline="true">Inline Button</a>
3. Toolbars:
Implement header and footer toolbars using data-role=”header” and data-role=”footer” attributes.
html <div data-role="header"> <h1>My App</h1> </div> <div data-role="footer"> <h4>© 2023 My App</h4> </div>
3.7 Theming:
jQuery Mobile offers an extensive theming system to customize the app’s appearance. You can either choose from a range of pre-defined themes or create your own.
1. Pre-defined Themes:
Include the desired theme’s CSS file in your HTML.
html <link rel="stylesheet" href="https://code.jquery.com/mobile/1.5.0/jquery.mobile-1.5.0.min.css" />
2. Custom Theme:
Create a custom theme using the ThemeRoller tool provided by jQuery Mobile.
html <link rel="stylesheet" href="path/to/your/custom-theme.css" />
3.8 Handling Events:
Interactivity is crucial for mobile apps. jQuery Mobile simplifies event handling for touch devices.
1. Tap Event:
The tap event is similar to the click event but is better suited for mobile devices.
html <script> $(document).on("tap", "#my-button", function() { alert("Button tapped!"); }); </script>
2. Swipe Event:
The swipe event allows you to detect left, right, up, or down swipes.
html <script> $(document).on("swipeleft swiperight", "#my-element", function(event) { if (event.type === "swipeleft") { // Handle left swipe } else { // Handle right swipe } }); </script>
3.9 Responsive Layouts:
jQuery Mobile excels at creating responsive layouts that adapt to various screen sizes.
1. Grids:
Use grids to create flexible layouts with multiple columns.
html <div class="ui-grid"> <div class="ui-block-a">Block A</div> <div class="ui-block-b">Block B</div> </div>
2. Panel:
The panel widget allows you to create collapsible sidebars for navigation.
html <div data-role="panel" id="my-panel"> <!-- Panel content here --> </div> <a href="#my-panel" data-role="button" data-icon="bars" data-iconpos="notext">Open Panel</a>
Conclusion
jQuery Mobile is an excellent framework for developing cross-platform mobile applications. Its simplicity, cross-platform compatibility, and rich feature set make it a top choice for developers aiming to create stunning mobile experiences. By leveraging jQuery Mobile’s power and flexibility, you can streamline the development process and deliver seamless mobile apps that delight users on any device or platform. So, get started with jQuery Mobile and embark on a journey to build impressive cross-platform mobile apps!
Table of Contents
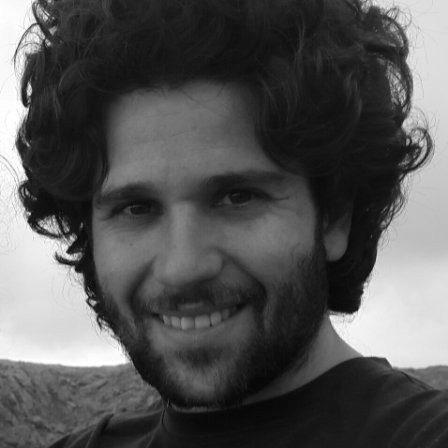
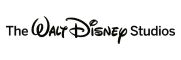