jQuery and CSS3: Creating Stunning Visual Effects
In the fast-paced world of web development, creating visually appealing and engaging user interfaces is a top priority. jQuery and CSS3 offer a dynamic duo for achieving stunning visual effects that captivate users and enhance the overall user experience. With the power of animations, transitions, and advanced styling, you can take your web design to the next level. In this blog, we’ll delve into the world of jQuery and CSS3, exploring various techniques and code samples that will help you create truly impressive visuals on your website.
Table of Contents
1. The Power of jQuery:
jQuery, a popular JavaScript library, revolutionized the way developers manipulate the Document Object Model (DOM) and interact with web elements. Its simplicity and cross-browser compatibility make it an excellent choice for creating dynamic visual effects without extensive coding. Let’s explore some key techniques:
1.1. Smooth Scrolling:
Smooth scrolling is a subtle yet impactful effect that can greatly enhance the user experience. With just a few lines of jQuery code, you can create a smooth transition between anchor links, guiding users through your website seamlessly. Here’s a sample code snippet to achieve smooth scrolling:
javascript $('a[href^="#"]').on('click', function(event) { event.preventDefault(); $('html, body').animate({ scrollTop: $($.attr(this, 'href')).offset().top }, 800); });
1.2. Image Sliders:
Image sliders are a fantastic way to showcase multiple images or content in a limited space. jQuery makes it easy to create image sliders with captivating transition effects. The following code demonstrates a simple image slider using the “slick” jQuery plugin:
html <div class="slider"> <div><img src="image1.jpg" alt="Image 1"></div> <div><img src="image2.jpg" alt="Image 2"></div> <div><img src="image3.jpg" alt="Image 3"></div> </div> <script> $('.slider').slick({ autoplay: true, dots: true, infinite: true, speed: 500, fade: true, cssEase: 'linear' }); </script>
2. CSS3 Magic:
CSS3 introduced a plethora of exciting features that allow developers to create stunning visual effects directly within their stylesheets. Let’s delve into some advanced CSS3 techniques that will elevate your web design:
2.1. Transitions:
CSS3 transitions enable smooth and gradual changes between property values. Whether you want to add a hover effect to a button or animate the expansion of a dropdown menu, transitions can be applied to achieve eye-catching effects. Here’s an example of a button with a hover transition:
css .button { background-color: #3498db; color: #ffffff; padding: 10px 20px; border: none; transition: background-color 0.3s ease; } .button:hover { background-color: #e74c3c; }
2.2. Keyframe Animations:
Keyframe animations offer precise control over complex animations. By defining keyframes at specific percentages of the animation duration, you can create intricate visual effects. Consider this example of a pulsating animation:
css @keyframes pulse { 0% { transform: scale(1); } 50% { transform: scale(1.2); } 100% { transform: scale(1); } } .pulsating-element { animation: pulse 2s infinite; }
2.3. Parallax Scrolling:
Parallax scrolling adds depth to your website by moving background elements at different speeds compared to foreground elements. This effect can create a sense of immersion and engagement. Here’s a simple CSS snippet for parallax scrolling:
css .parallax-section { background-image: url('background.jpg'); background-attachment: fixed; background-position: center; background-repeat: no-repeat; background-size: cover; height: 600px; }
3. Combining jQuery and CSS3:
The true magic happens when you combine the capabilities of jQuery and CSS3. This synergy allows you to create intricate visual effects that seamlessly interact with user actions. Let’s explore how you can achieve this synergy:
3.1. Animated Modals:
Modal windows are an elegant way to present additional information or content without navigating away from the current page. By combining jQuery for interaction and CSS3 for animation, you can create visually appealing modals. Here’s a basic example:
html <div class="modal"> <div class="modal-content"> <p>This is the modal content.</p> <button class="close-modal">Close</button> </div> </div> <script> $('.open-modal').click(function() { $('.modal').fadeIn(); }); $('.close-modal').click(function() { $('.modal').fadeOut(); }); </script> <style> .modal { display: none; position: fixed; top: 0; left: 0; width: 100%; height: 100%; background-color: rgba(0, 0, 0, 0.7); } .modal-content { position: absolute; top: 50%; left: 50%; transform: translate(-50%, -50%); background-color: #fff; padding: 20px; box-shadow: 0 2px 5px rgba(0, 0, 0, 0.3); } </style>
3.2. Animated Hover Effects:
By combining jQuery’s event handling with CSS3’s animation capabilities, you can create interactive and visually captivating hover effects. Consider this example of a card flipping effect:
html <div class="flip-card"> <div class="flip-card-inner"> <div class="flip-card-front"> <p>Front Content</p> </div> <div class="flip-card-back"> <p>Back Content</p> </div> </div> </div> <script> $('.flip-card').hover(function() { $(this).find('.flip-card-inner').toggleClass('flipped'); }); </script> <style> .flip-card { perspective: 1000px; width: 200px; height: 300px; } .flip-card-inner { width: 100%; height: 100%; transition: transform 0.5s; transform-style: preserve-3d; } .flip-card.flipped .flip-card-inner { transform: rotateY(180deg); } .flip-card-front, .flip-card-back { width: 100%; height: 100%; position: absolute; backface-visibility: hidden; } .flip-card-front { background-color: #3498db; color: white; display: flex; justify-content: center; align-items: center; } .flip-card-back { background-color: #e74c3c; color: white; transform: rotateY(180deg); display: flex; justify-content: center; align-items: center; } </style>
Conclusion
In the realm of web design, creating stunning visual effects is essential for capturing user attention and delivering an exceptional user experience. By leveraging the power of jQuery and CSS3, you have a rich toolkit at your disposal. From smooth scrolling and image sliders to transitions and keyframe animations, these technologies empower you to bring your creative visions to life. Remember to experiment, iterate, and continuously refine your designs as you explore the boundless possibilities that jQuery and CSS3 offer. Your website’s visual appeal will undoubtedly leave a lasting impression on visitors, setting you apart in the competitive online landscape.
Table of Contents
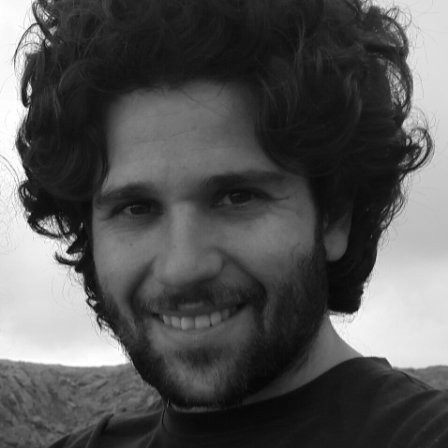
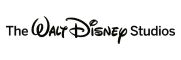