Customizing jQuery UI Widgets: Extending Functionality
In the realm of web development, creating rich and interactive user interfaces is paramount. jQuery UI, a widely used library, offers a collection of pre-built widgets that enable developers to quickly implement features like accordions, tabs, datepickers, and more. While these widgets come with a range of styling and behavior options out of the box, there might be instances when you need to take them a step further to perfectly match your project’s unique requirements. This is where customizing and extending jQuery UI widgets becomes a valuable skill.
Table of Contents
1. Understanding the Power of jQuery UI Widgets
Before delving into the world of customization, it’s crucial to understand the core concepts of jQuery UI widgets. A widget, in this context, is essentially a self-contained component that provides a specific functionality. These widgets are designed to be easily reusable, making it possible to achieve consistent and polished interfaces across different parts of your web application.
jQuery UI widgets typically follow a structured pattern. They consist of HTML, CSS, and JavaScript components. The HTML defines the structure of the widget, the CSS styles it, and the JavaScript adds interactivity and behavior. This separation of concerns not only enhances maintainability but also allows for seamless customization without disrupting the core functionality.
2. Extending jQuery UI Widgets
The true magic of jQuery UI lies in its extensibility. The library provides developers with the ability to extend existing widgets or even create entirely new ones. This means that you can build upon the provided widgets to add features or behaviors that cater specifically to your project’s requirements. Let’s explore how you can extend jQuery UI widgets to enhance their functionality.
2.1. Extending Options and Behaviors
A common way to customize jQuery UI widgets is by extending their available options and behaviors. Most widgets offer a range of options that control aspects like animations, styling, and event handling. To customize a widget’s behavior, you can modify these options according to your needs.
For example, let’s consider the Autocomplete widget. By default, this widget displays a dropdown list of suggestions as users type into an input field. You can extend this behavior by adjusting options like the delay before suggestions appear, the minimum characters required to trigger suggestions, and the source of suggestion data.
javascript $("#searchInput").autocomplete({ delay: 300, minLength: 2, source: customSuggestionSource });
In this code snippet, the delay option sets a slight delay before suggestions appear, the minLength option ensures that suggestions only appear after typing at least two characters, and the source option is customized to fetch suggestions from a custom data source.
2.2. Creating Custom Widgets
While extending existing widgets is powerful, there might be scenarios where your project requires a unique functionality that isn’t available in the standard jQuery UI library. In such cases, you can create your own custom widgets.
Let’s say you’re building an e-commerce website and need a widget to showcase products with a sliding carousel effect. You can create a custom widget that encapsulates this behavior. Here’s a simplified example:
javascript $.widget("custom.productCarousel", { options: { speed: 500, autoPlay: true }, _create: function() { this._initializeCarousel(); }, _initializeCarousel: function() { // Initialization logic here, e.g., setting up event listeners }, // Additional methods for controlling the carousel go here });
In this snippet, you’re defining a new widget named productCarousel with default options like speed and autoPlay. The _create method is where the widget’s initialization logic resides, and _initializeCarousel is a custom method you’ll define to set up the carousel behavior. With this custom widget, you can now easily apply the product carousel functionality to any element on your website.
2.3. Styling and Theming
Beyond functionality, the visual aspect of widgets is also vital for a seamless user experience. jQuery UI widgets come with default themes, but you might need to tailor the appearance to match your project’s design language.
Styling jQuery UI widgets involves overriding the default CSS styles with your custom ones. To do this, you can leverage the widget’s classes and IDs to target specific elements. For instance, if you’re customizing the Tabs widget, you can target the tabs themselves, the active tab, and the tab content to apply your own styles.
css /* Custom styling for Tabs widget */ .ui-tabs { background-color: #f4f4f4; border: 1px solid #ddd; border-radius: 5px; } .ui-tabs .ui-tabs-nav li { background-color: #fff; border: 1px solid #ccc; } .ui-tabs .ui-tabs-nav .ui-state-active { background-color: #fff; border-bottom-color: #fff; } .ui-tabs .ui-tabs-panel { padding: 20px; }
In this example, you’re customizing the background color, borders, and spacing of the Tabs widget to create a more visually appealing and cohesive design.
3. Best Practices for Customizing jQuery UI Widgets
As you embark on your journey of extending and customizing jQuery UI widgets, here are some best practices to keep in mind:
- Know the Widget API: Familiarize yourself with the documentation for the specific widget you’re working with. Understand the available options, methods, and events to make informed customization decisions.
- Keep Compatibility in Mind: While customization is empowering, it’s essential to ensure that your customizations don’t lead to compatibility issues across different browsers and devices.
- Modularize Your Code: If you’re creating custom widgets, aim to keep your code modular and organized. Break down functionality into smaller methods to enhance reusability and maintainability.
- Separate Structure, Style, and Behavior: Adhere to the separation of concerns principle by keeping HTML, CSS, and JavaScript in their respective places. This will help prevent conflicts and make future updates smoother.
- Test Thoroughly: Whenever you make customizations, thoroughly test your widgets across various scenarios to ensure that they function as expected and provide a consistent user experience.
Conclusion
jQuery UI widgets serve as powerful tools for quickly implementing interactive elements in web applications. However, their true potential is unlocked when you dive into the realm of customization and extension. By understanding the widget architecture, extending options and behaviors, creating custom widgets, and applying tailored styling, you can elevate the user experience and tailor your web application to your exact specifications. With the right balance of creativity and technical proficiency, you can transform the standard widgets into dynamic components that truly make your application stand out. So, embrace the art of customization and let your creativity shine in the world of web development.
Table of Contents
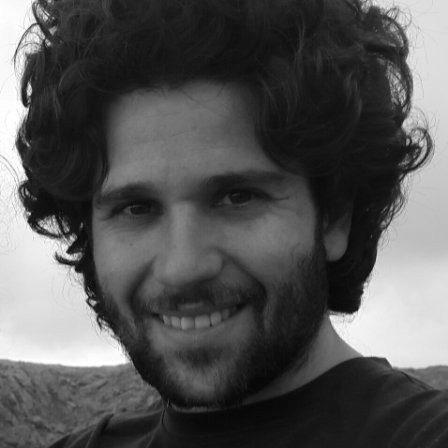
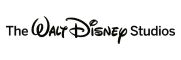