jQuery and D3.js: Integrating Data Visualization Libraries
In today’s data-driven world, effective data visualization is key to understanding complex information. jQuery and D3.js are two powerful libraries that, when combined, can take your data visualizations to the next level. In this blog, we’ll delve into the art of integrating jQuery and D3.js to create interactive and captivating data visualizations.
Table of Contents
1. Why jQuery and D3.js?
1.1. jQuery: The Versatile Helper
jQuery is a fast, small, and feature-rich JavaScript library that simplifies HTML document traversal and manipulation, event handling, and animation. It provides a concise and efficient way to interact with HTML documents and offers a wide range of plugins that extend its capabilities.
jQuery’s simplicity and versatility make it an excellent choice for handling DOM manipulation, user interactions, and AJAX requests. When it comes to integrating data visualization libraries, jQuery serves as the glue that binds everything together, making your applications more interactive and responsive.
1.2. D3.js: The Data-Driven Wizard
D3.js (Data-Driven Documents) is a JavaScript library that allows you to bring data to life using HTML, SVG, and CSS. It is renowned for its ability to create stunning, data-driven visualizations that are not only informative but also visually appealing.
D3.js provides a powerful set of tools for binding data to the Document Object Model (DOM) and applying data-driven transformations to the document. With D3.js, you can create bar charts, line graphs, scatter plots, maps, and more. It’s a perfect choice for crafting custom data visualizations that suit your specific needs.
2. Setting Up the Environment
Before we dive into integrating jQuery and D3.js, let’s ensure we have the necessary tools in place. Here’s how to set up your development environment:
Step 1: HTML Structure
Start by creating the basic structure of your HTML file. Here’s a minimal example:
html <!DOCTYPE html> <html> <head> <title>Data Visualization with jQuery and D3.js</title> <link rel="stylesheet" type="text/css" href="styles.css"> </head> <body> <div id="visualization"></div> <script src="jquery.min.js"></script> <script src="d3.min.js"></script> <script src="app.js"></script> </body> </html>
In this example, we link to external CSS and JavaScript files. Ensure you download the latest versions of jQuery and D3.js and place them in your project folder.
Step 2: Project Structure
Create a project folder with the following structure:
arduino project-folder/ ??? index.html ??? styles.css ??? jquery.min.js ??? d3.min.js ??? app.js
This structure separates your HTML, CSS, and JavaScript files, keeping your project organized and easy to maintain.
Step 3: Installing jQuery and D3.js
To include jQuery and D3.js in your project, you can either download the libraries and include them locally as shown in the HTML structure above, or you can use a Content Delivery Network (CDN) for faster loading in production.
Now that your environment is set up, let’s proceed to integrate jQuery and D3.js for data visualization.
3. Integrating jQuery and D3.js
In this section, we’ll explore various ways to integrate jQuery and D3.js to create interactive data visualizations.
3.1. Loading Data with jQuery
jQuery’s AJAX capabilities make it easy to load data from external sources, such as JSON files or APIs. Here’s a simple example of how to load data using jQuery:
javascript $(document).ready(function () { $.getJSON("data.json", function (data) { // Process the data here }); });
In this code snippet, we use $.getJSON to fetch data from a JSON file named “data.json.” Once the data is loaded, you can process it as needed.
3.2. Binding Data with D3.js
D3.js excels at binding data to DOM elements and applying data-driven transformations. Let’s say we have loaded some data about monthly sales, and we want to create a bar chart to visualize it:
javascript // Assuming data is an array of objects with 'month' and 'sales' properties // Select the container element var svg = d3.select("#visualization") .append("svg") .attr("width", 500) .attr("height", 300); // Create scales for mapping data to visual properties var xScale = d3.scaleBand() .domain(data.map(function (d) { return d.month; })) .range([0, 400]) .padding(0.1); var yScale = d3.scaleLinear() .domain([0, d3.max(data, function (d) { return d.sales; })]) .range([0, 250]); // Create and append rectangles for each data point svg.selectAll("rect") .data(data) .enter() .append("rect") .attr("x", function (d) { return xScale(d.month); }) .attr("y", function (d) { return 300 - yScale(d.sales); }) .attr("width", xScale.bandwidth()) .attr("height", function (d) { return yScale(d.sales); }) .attr("fill", "blue");
In this example, we select the #visualization element and create an SVG canvas within it. We define scales to map data values to visual properties like x and y positions and heights. Then, we bind the data to SVG rectangles and set their attributes accordingly.
3.3. Adding Interactivity
Integrating jQuery and D3.js also allows you to add interactivity to your visualizations. For instance, you can use jQuery to respond to user interactions and update the D3.js visualization accordingly.
javascript // Assuming we have a button with the id "updateButton" $("#updateButton").click(function () { // Generate new data or modify existing data var newData = generateData(); // Update the D3.js visualization with the new data // ... // You can also add animations and transitions with D3.js d3.selectAll("rect") .transition() .duration(1000) .attr("height", function (d) { return yScale(newData[d.month]); }); });
In this code, we use jQuery to listen for a click event on the “updateButton.” When clicked, we generate new data and use D3.js to update the visualization, adding a smooth transition effect.
4. Case Study: Creating a Dynamic Bar Chart
To illustrate the power of integrating jQuery and D3.js, let’s walk through the process of creating a dynamic bar chart that allows users to toggle between different data sets. We’ll start by setting up the HTML structure, loading data with jQuery, and then building the chart with D3.js.
Step 1: HTML Structure
Here’s the HTML structure for our dynamic bar chart:
html <!DOCTYPE html> <html> <head> <title>Dynamic Bar Chart</title> <link rel="stylesheet" type="text/css" href="styles.css"> </head> <body> <div id="visualization"></div> <button id="toggleData">Toggle Data</button> <script src="jquery.min.js"></script> <script src="d3.min.js"></script> <script src="app.js"></script> </body> </html>
We’ve added a “Toggle Data” button to allow users to switch between different data sets.
Step 2: Loading Data with jQuery
In our “app.js” file, we’ll load two sets of data using jQuery. We assume that we have two JSON files, “data1.json” and “data2.json,” containing different datasets.
javascript $(document).ready(function () { var currentData = "data1.json"; // Start with the first dataset // Function to load and update data function loadAndUpdateData() { $.getJSON(currentData, function (data) { // Call a function to create/update the chart using D3.js createOrUpdateChart(data); }); } // Load and update data when the page loads loadAndUpdateData(); // Toggle data when the button is clicked $("#toggleData").click(function () { // Toggle between the two datasets currentData = currentData === "data1.json" ? "data2.json" : "data1.json"; loadAndUpdateData(); }); });
In this code, we use jQuery to load the initial data when the page loads and to toggle between datasets when the “Toggle Data” button is clicked.
Step 3: Creating the Dynamic Bar Chart with D3.js
Now, let’s create the “createOrUpdateChart” function to build and update our dynamic bar chart using D3.js:
javascript var svg = d3.select("#visualization") .append("svg") .attr("width", 500) .attr("height", 300); var xScale, yScale; function createOrUpdateChart(data) { // Clear the previous chart svg.selectAll("*").remove(); // Create scales and axes xScale = d3.scaleBand() .domain(data.map(function (d) { return d.month; })) .range([0, 400]) .padding(0.1); yScale = d3.scaleLinear() .domain([0, d3.max(data, function (d) { return d.sales; })]) .range([0, 250]); var xAxis = d3.axisBottom(xScale); var yAxis = d3.axisLeft(yScale); // Append axes to the SVG svg.append("g") .attr("class", "x-axis") .attr("transform", "translate(0, 250)") .call(xAxis); svg.append("g") .attr("class", "y-axis") .call(yAxis); // Create and append rectangles for each data point svg.selectAll("rect") .data(data) .enter() .append("rect") .attr("x", function (d) { return xScale(d.month); }) .attr("y", function (d) { return 300 - yScale(d.sales); }) .attr("width", xScale.bandwidth()) .attr("height", function (d) { return yScale(d.sales); }) .attr("fill", "blue"); }
In this function, we first clear the previous chart to ensure a clean update. We then create scales, axes, and append them to the SVG. Finally, we create and append rectangles for the data points, making sure to update the scales and axes as needed.
With this setup, users can toggle between two datasets, and the bar chart will update accordingly. This dynamic chart showcases the seamless integration of jQuery and D3.js to create interactive data visualizations.
Conclusion
Integrating jQuery and D3.js opens up a world of possibilities for creating captivating and interactive data visualizations. jQuery simplifies data loading and user interaction handling, while D3.js empowers you to create stunning visualizations that bring data to life.
By combining these two libraries, you can build dynamic and responsive data-driven applications that engage your audience and deliver valuable insights. Whether you’re creating charts, graphs, or custom data visualizations, jQuery and D3.js make the process more accessible and enjoyable.
So, roll up your sleeves, experiment with different types of visualizations, and harness the synergy of jQuery and D3.js to craft data-driven masterpieces that make your data shine. Happy coding!
Table of Contents
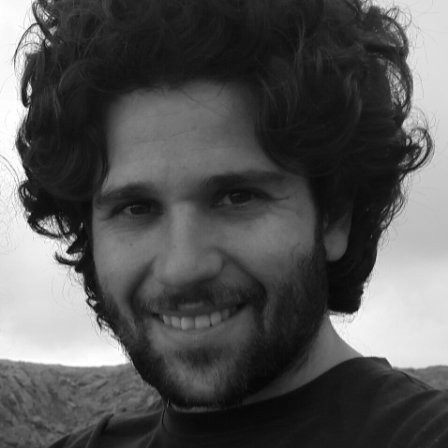
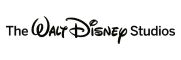