jQuery Data Binding: Keeping Your UI in Sync with Backend Data
In the world of web development, creating dynamic and responsive user interfaces is essential to provide a seamless user experience. As applications grow in complexity, managing the synchronization of frontend and backend data becomes increasingly challenging. This is where jQuery Data Binding comes to the rescue. By enabling automatic updates of UI elements in response to changes in backend data, jQuery Data Binding streamlines development and enhances user interaction. In this article, we’ll delve into the concept of jQuery Data Binding, its advantages, and how you can implement it effectively in your projects.
Table of Contents
1. Understanding Data Binding
Data binding is a fundamental concept that simplifies the process of keeping UI elements in sync with backend data. Traditionally, developers had to manually manipulate the DOM (Document Object Model) to reflect changes in data. However, this approach often led to complex and error-prone code, making maintenance and scalability a challenge. Data binding automates this process by establishing a connection between UI components and data sources, ensuring that any changes to the data are automatically reflected in the UI, and vice versa.
2. The Advantages of Data Binding
Data binding offers several advantages that significantly enhance the efficiency and maintainability of your web applications:
- Reduced Code Complexity: Data binding eliminates the need for manual DOM manipulation, resulting in cleaner and more readable code. Developers can focus on the logic of their applications rather than worrying about synchronizing UI updates.
- Real-time Updates: With data binding, UI elements update in real-time as the underlying data changes. This instant feedback provides users with a smoother and more engaging experience.
- Enhanced User Experience: By seamlessly reflecting changes in data, data binding creates a more interactive and responsive user interface. Users can immediately see the impact of their actions, fostering a sense of control and engagement.
- Simplified Maintenance: As your application evolves, maintaining data binding is easier than managing manual updates. When data structures change, the binding mechanisms can be adjusted without the need to rewrite extensive portions of the codebase.
3. Introducing jQuery Data Binding
jQuery, a popular JavaScript library, offers a robust solution for implementing data binding in your web applications. jQuery simplifies many aspects of JavaScript programming, and data binding is no exception.
3.1. Setting Up jQuery Data Binding
To get started with jQuery data binding, ensure that you’ve included the jQuery library in your project. You can either download it and host it locally or include it from a content delivery network (CDN). Here’s an example of including jQuery via a CDN:
html <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
3.2. Basic Data Binding Example
Let’s consider a simple scenario where you want to bind a user’s name to an HTML element and have it automatically update as the user’s name changes. Here’s how you can achieve this using jQuery data binding:
3.2.1. HTML:
html <div id="user-profile"> <p>Welcome, <span id="user-name"></span>!</p> </div>
3.2.2. JavaScript:
javascript $(document).ready(function() { const user = { name: "John Doe" }; const $userName = $("#user-name"); // Initial binding $userName.text(user.name); // Simulate a data change setTimeout(function() { user.name = "Jane Smith"; // Update the UI automatically due to data binding $userName.text(user.name); }, 3000); });
In this example, we first define the initial user object with a name property. We select the user-name span element using jQuery and bind its content to the user.name property. After a simulated delay of 3 seconds, we update the user’s name and observe how the UI automatically reflects the change.
3.3. Two-way Data Binding
jQuery data binding also supports two-way data binding, where changes in both the UI and the data source are synchronized. This is especially useful for forms and interactive elements.
javascript $(document).ready(function() { const user = { name: "John Doe" }; const $userNameInput = $("#user-name-input"); const $userNameDisplay = $("#user-name-display"); // Initial binding $userNameInput.val(user.name); $userNameDisplay.text(user.name); // Input change triggers data update $userNameInput.on("input", function() { user.name = $userNameInput.val(); // Update the UI automatically due to data binding $userNameDisplay.text(user.name); }); });
In this example, we have an input field where the user’s name can be edited. As the user types, the input event triggers a change in the user.name property, which in turn updates the UI display.
4. Best Practices for jQuery Data Binding
To make the most of jQuery data binding and ensure efficient development, consider the following best practices:
4.1. Use a Clear Data Structure:
Organize your data in a structured format that aligns with your UI components. This simplifies the binding process and makes your code more intuitive.
4.2. Delegate Event Handling:
Delegate event handling to parent elements whenever possible. This ensures that dynamically added or removed elements also adhere to the binding rules.
4.3. Minimize Direct DOM Manipulation:
While jQuery provides tools for directly manipulating the DOM, prefer using data binding mechanisms to maintain a clear separation between your data and UI.
4.4. Avoid Overbinding:
Avoid binding every single data property to a UI element. Overbinding can lead to unnecessary performance overhead. Instead, focus on the most critical data elements.
4.5. Consider Using a MVVM Library:
For larger and more complex applications, consider using a Model-View-ViewModel (MVVM) library like Knockout.js or Vue.js. These libraries provide advanced data binding capabilities and help manage complex UI interactions.
Conclusion
jQuery data binding offers a powerful way to streamline your UI development process and create responsive web applications with minimal effort. By automating the synchronization of frontend and backend data, data binding enhances user experience and simplifies maintenance. As you delve deeper into the world of web development, mastering jQuery data binding will prove to be an invaluable skill, empowering you to create dynamic, engaging, and user-friendly interfaces. So, embrace the power of data binding, and elevate your web development projects to new heights of efficiency and interactivity.
Table of Contents
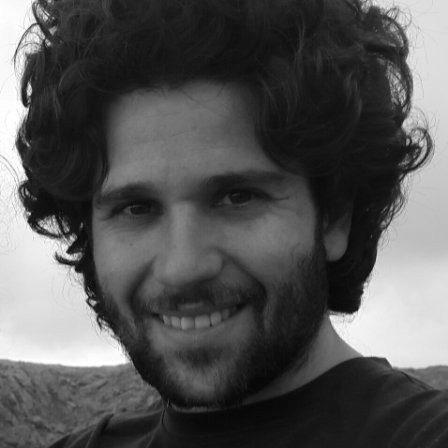
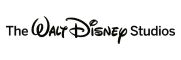