jQuery Data Tables: Sorting, Filtering, and Pagination Made Simple
In the world of web development, presenting tabular data is a common task. Whether you’re displaying product listings, financial data, or user information, having a well-organized and user-friendly table can greatly enhance the user experience. jQuery Data Tables is a powerful plugin that simplifies the process of adding sorting, filtering, and pagination functionality to your HTML tables. In this blog, we’ll explore how to use jQuery Data Tables to transform your tables into interactive and easily navigable elements.
Table of Contents
1. What are jQuery Data Tables?
jQuery Data Tables is a popular jQuery plugin that allows you to enhance standard HTML tables by adding features like sorting, filtering, and pagination. It’s built to be flexible, customizable, and easy to implement. With Data Tables, you can turn a static HTML table into an interactive and dynamic component that users can interact with effortlessly.
2. Getting Started
To get started with jQuery Data Tables, you’ll need to include the necessary files in your HTML document. You can either download the plugin files and host them on your server or use a Content Delivery Network (CDN) for quicker setup:
html <!-- jQuery library (required) --> <script src="https://code.jquery.com/jquery-x.x.x.min.js"></script> <!-- jQuery Data Tables --> <link rel="stylesheet" href="https://cdn.datatables.net/1.11.1/css/jquery.dataTables.min.css"> <script src="https://cdn.datatables.net/1.11.1/js/jquery.dataTables.min.js"></script>
Replace x.x.x in the script tag with the version you want to use. It’s always recommended to use the latest stable version to benefit from bug fixes and improvements.
3. Basic Implementation
Let’s start with a basic implementation of jQuery Data Tables on a simple HTML table:
html <table id="myTable"> <thead> <tr> <th>Name</th> <th>Age</th> <th>City</th> </tr> </thead> <tbody> <tr> <td>John Doe</td> <td>30</td> <td>New York</td> </tr> <tr> <td>Jane Smith</td> <td>25</td> <td>Los Angeles</td> </tr> <!-- Add more rows as needed --> </tbody> </table>
Now, let’s initialize the table with jQuery Data Tables:
javascript $(document).ready(function() { $('#myTable').DataTable(); });
With just these few lines of code, your table will now have sorting, filtering, and pagination functionality.
4. Sorting Data
Sorting allows users to rearrange the table rows based on the values in a specific column. By default, Data Tables will enable sorting for all columns. To disable sorting for a specific column, you can add the “sorting_disabled” class to the respective <th> element.
html <thead> <tr> <th class="sorting_disabled">Name</th> <th>Age</th> <th>City</th> </tr> </thead>
You can also set the default sorting column and order during initialization:
javascript $(document).ready(function() { $('#myTable').DataTable({ "order": [[1, "asc"]] // Sort by the second column (Age) in ascending order }); });
5. Filtering Data
Filtering allows users to search for specific data within the table. By default, Data Tables will create a search input box above the table. Users can type in their query, and the table will automatically update to display only the rows that match the search term.
You can customize the default behavior by using various options:
javascript $(document).ready(function() { $('#myTable').DataTable({ "searching": false, // Disable searching "searchDelay": 500, // Add a delay before triggering search (in milliseconds) // More options can be added here }); });
6. Pagination
Pagination divides the table data into multiple pages, making it easier for users to navigate through a large dataset. By default, Data Tables will display ten rows per page. If you have fewer rows than the specified limit, no pagination will be applied.
You can customize the number of rows per page and other pagination settings:
javascript $(document).ready(function() { $('#myTable').DataTable({ "paging": true, // Enable pagination "pageLength": 5, // Show five rows per page // More options can be added here }); });
7. Customization
One of the strengths of jQuery Data Tables is its customization options. You can modify various aspects of the table’s appearance, behavior, and functionality to match the style of your website and the specific requirements of your data presentation.
8. Styling
To apply custom CSS styling to the Data Table, you can add your CSS rules or override the default styles provided by the plugin. For example:
css /* Change font size of the table header */ #myTable th { font-size: 16px; } /* Add background color to even rows */ #myTable tbody tr:nth-child(even) { background-color: #f2f2f2; } /* Customize the pagination buttons */ .dataTables_paginate .paginate_button { background-color: #007BFF; color: #fff; }
9. Callback Functions
jQuery Data Tables provides various callback functions that allow you to intercept and modify the default behavior of the plugin. For instance, you can use the drawCallback to perform actions after the table is redrawn:
javascript $(document).ready(function() { $('#myTable').DataTable({ // other options... "drawCallback": function(settings) { console.log('The table has been redrawn.'); } }); });
10. Extensions
Data Tables also offers extensions that can further extend its capabilities, such as responsive design support, additional sorting options, and custom data source loading.
javascript $(document).ready(function() { $('#myTable').DataTable({ // other options... "responsive": true, // Enable responsive design support // Add more extensions here }); });
Conclusion
jQuery Data Tables simplifies the process of adding essential features like sorting, filtering, and pagination to your HTML tables. With its ease of use and extensive customization options, you can create interactive and user-friendly tables that enhance the overall user experience on your website. By following the guidelines and code examples provided in this blog, you can quickly integrate Data Tables into your projects and take your tabular data presentation to the next level. Happy coding!
Remember to keep the plugin updated and refer to the official documentation for more advanced usage and to stay up-to-date with the latest features and improvements. Happy coding!
Table of Contents
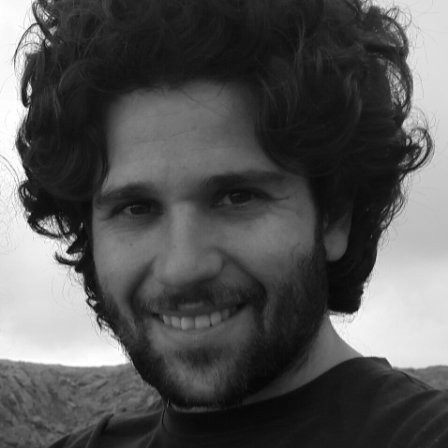
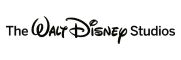