Exploring jQuery Deferred Objects: Handling Asynchronous Operations
In the world of web development, asynchronous operations play a crucial role in creating responsive and efficient applications. However, managing these operations can become quite challenging, leading to callback hell and tangled code. This is where jQuery Deferred Objects come to the rescue, offering an elegant solution for handling asynchronous tasks with ease and maintaining code readability.
Table of Contents
1. Understanding Asynchronous Operations
Before we delve into the realm of jQuery Deferred Objects, let’s briefly understand what asynchronous operations are. In JavaScript, many tasks, such as fetching data from a server or handling user interactions, are non-blocking and can be executed independently of the main program flow. As a result, they don’t hinder the overall performance of the application.
However, managing the execution order and dependencies of these asynchronous tasks can become complex, leading to callback functions nested within callback functions. This is commonly referred to as “callback hell,” and it can quickly make your code hard to read and maintain.
2. Introducing jQuery Deferred Objects
jQuery Deferred Objects provide a powerful mechanism to manage asynchronous operations in a more organized and readable manner. They allow you to execute tasks asynchronously and define the order in which they should be executed, without resorting to deeply nested callbacks.
3. Creating a Deferred Object
To create a Deferred object in jQuery, you can use the $.Deferred() constructor. This object represents a computation that might complete in the future. You can then attach callbacks to this object to be executed when the computation is done.
javascript // Creating a Deferred object var deferred = $.Deferred(); // Attaching success and failure callbacks deferred.done(function(result) { console.log("Success:", result); }).fail(function(error) { console.log("Error:", error); });
4. Resolving and Rejecting the Deferred Object
You can manually resolve a Deferred object using the resolve() method and pass a value that will be sent to the success callbacks. Similarly, the reject() method can be used to signal failure and trigger the execution of failure callbacks.
javascript // Manually resolving a Deferred object deferred.resolve("Operation successful!"); // Manually rejecting a Deferred object deferred.reject("An error occurred.");
5. Chaining Deferred Operations with Promises
jQuery Deferred Objects often work in conjunction with promises. A promise represents the eventual completion (or failure) of an asynchronous operation and allows you to attach callbacks to be executed when the operation is complete.
javascript // Creating a promise from a Deferred object var promise = deferred.promise(); // Attaching callback to the promise promise.then(function(result) { console.log("Promise resolved:", result); }).catch(function(error) { console.log("Promise rejected:", error); });
6. Simplifying Asynchronous Workflows
One of the significant advantages of using jQuery Deferred Objects is that they enable you to simplify complex asynchronous workflows. Let’s take an example where you need to fetch data from an API, perform some processing, and then update the UI.
6.1. Traditional Approach
javascript // Traditional callback hell fetchData(function(data) { processData(data, function(processedData) { updateUI(processedData, function() { console.log("All operations completed!"); }); }); });
6.2. Using jQuery Deferred Objects
javascript // Using jQuery Deferred Objects fetchData() .then(processData) .then(updateUI) .done(function() { console.log("All operations completed!"); });
As you can see, the code using jQuery Deferred Objects is much cleaner and easier to follow. It represents a linear sequence of operations, making the code more maintainable and less error-prone.
7. Handling Multiple Asynchronous Tasks
In real-world applications, you often encounter scenarios where multiple asynchronous tasks need to be executed in parallel or in a specific order. jQuery Deferred Objects provide a concise way to handle these situations.
7.1. Running Tasks in Parallel
javascript // Running tasks in parallel var task1 = $.ajax({ url: "api/task1", method: "GET" }); var task2 = $.ajax({ url: "api/task2", method: "GET" }); $.when(task1, task2).done(function(result1, result2) { console.log("Task 1 result:", result1); console.log("Task 2 result:", result2); });
7.2. Executing Tasks Sequentially
javascript // Executing tasks sequentially var taskQueue = []; taskQueue.push(function() { return $.ajax({ url: "api/task1", method: "GET" }); }); taskQueue.push(function(result1) { return $.ajax({ url: "api/task2", method: "GET", data: result1 }); }); taskQueue.reduce(function(prevTask, nextTask) { return prevTask.then(nextTask); }, $.when()).done(function(finalResult) { console.log("Final result:", finalResult); });
8. Handling Errors Gracefully
Error handling is a crucial aspect of managing asynchronous operations. jQuery Deferred Objects offer mechanisms to handle errors gracefully and prevent them from disrupting the entire application.
8.1. Error Handling with Deferred Objects
javascript var deferred = $.Deferred(); deferred.then(function() { // Successful operation console.log("Success"); }).catch(function(error) { // Handle errors console.log("Error:", error); }); // Simulating an error deferred.reject("An error occurred.");
8.2. Error Handling in Chained Operations
javascript fetchData() .then(processData) .then(updateUI) .done(function() { console.log("All operations completed!"); }) .catch(function(error) { console.log("An error occurred:", error); });
Conclusion
jQuery Deferred Objects provide a valuable tool for managing asynchronous operations in a structured and efficient manner. By using Deferreds, you can escape the tangled web of nested callbacks and create more readable, maintainable, and error-resistant code. Whether you’re dealing with simple asynchronous tasks or complex workflows, jQuery Deferred Objects empower you to handle these challenges with confidence.
Incorporating jQuery Deferred Objects into your development toolkit can significantly enhance your ability to create responsive and effective web applications. By understanding the concepts and examples discussed in this blog, you’re well on your way to mastering the art of handling asynchronous operations in JavaScript. So go ahead, explore the power of Deferred Objects, and elevate your coding skills to the next level!
Table of Contents
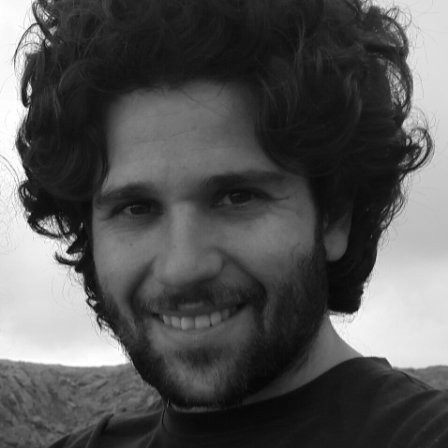
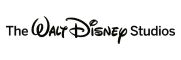