The Art of DOM Manipulation: jQuery’s Key Functionality
In the world of web development, manipulating the Document Object Model (DOM) is a crucial task. It allows developers to dynamically change the content, structure, and style of web pages. While there are various JavaScript libraries and frameworks available, jQuery stands out as a popular choice due to its simplicity and extensive support for DOM manipulation.
This blog post will explore the key functionalities of jQuery for DOM manipulation. We’ll cover the fundamental concepts, selecting elements, manipulating properties, handling events, and more. Get ready to unlock the art of DOM manipulation with jQuery!
1. What is DOM Manipulation?
DOM manipulation refers to the process of modifying elements within an HTML document dynamically. It involves accessing and altering elements, their content, attributes, and other properties using JavaScript or libraries like jQuery. By manipulating the DOM, developers can create interactive and responsive web applications.
2. Why Choose jQuery?
jQuery is a lightweight JavaScript library that simplifies DOM manipulation, making it accessible to developers of all skill levels. It provides a wide range of functions and utilities, reducing the amount of code needed for common tasks. Additionally, jQuery offers cross-browser compatibility, which eliminates the need for writing browser-specific code.
Now, let’s dive into the key functionalities of jQuery for DOM manipulation.
3. Selecting Elements
3.1 Basic Selectors
jQuery makes selecting elements from the DOM a breeze. It provides a simple and concise syntax for targeting elements based on their tag name, class, ID, or even custom attributes. Here’s an example:
javascript // Selecting elements by tag name $("p").addClass("highlight"); // Selecting elements by class $(".box").fadeOut(); // Selecting elements by ID $("#title").html("New Title"); // Selecting elements by custom attribute $("[data-toggle='tooltip']").tooltip();
3.2 Attribute Selectors
With jQuery, you can also select elements based on their attribute values. This allows you to target specific elements that match certain criteria. Here’s an example:
javascript // Selecting elements with a specific attribute $("input[type='text']").val("Hello!"); // Selecting elements with an attribute starting with a specific value $("img[src^='images/']").hide(); // Selecting elements with an attribute ending with a specific value $("a[href$='.pdf']").addClass("pdf-link"); // Selecting elements with an attribute containing a specific value $("div[class*='error']").slideDown();
3.3 Traversing the DOM
jQuery provides a range of methods to navigate and traverse the DOM tree efficiently. This allows you to select elements based on their relationship with other elements. Here are a few examples:
javascript // Selecting the parent element $("span").parent().addClass("highlight"); // Selecting the first child element $("ul").find("li:first").addClass("active"); // Selecting the next sibling element $(".box").next().addClass("selected"); // Selecting all the previous sibling elements $(".box").prevAll().addClass("previous");
4. Manipulating Properties
4.1 Changing Text and HTML Content
jQuery offers simple methods to modify the text and HTML content of elements. You can easily update or retrieve the content within an element. Take a look at these examples:
javascript // Changing the text content $("#message").text("Hello, World!"); // Changing the HTML content $("#container").html("<p>Welcome to my website!</p>"); // Retrieving the text content var text = $("#title").text(); // Retrieving the HTML content var html = $("#content").html();
4.2 Modifying CSS Styles
Modifying CSS styles is another common requirement in DOM manipulation. With jQuery, you can add, remove, or toggle CSS classes, as well as directly modify individual style properties. Here’s an example:
javascript // Adding a CSS class $("button").addClass("btn-primary"); // Removing a CSS class $("input").removeClass("error"); // Toggling a CSS class $("div").toggleClass("visible"); // Modifying a style property $("#box").css("background-color", "blue");
4.3 Adjusting Element Attributes
jQuery allows you to manipulate element attributes easily. You can modify attributes like src, href, disabled, and more. Here are a few examples:
javascript // Modifying the source attribute of an image $("img").attr("src", "newimage.jpg"); // Disabling an input element $("input[type='text']").attr("disabled", true); // Removing an attribute $("a").removeAttr("target");
5. Handling Events
5.1 Binding and Unbinding Events
Event handling is a crucial aspect of web development. jQuery provides a convenient way to bind and unbind events to elements. Here’s how you can attach and detach event handlers:
javascript // Binding a click event handler $("button").click(function() { // Handle the click event }); // Unbinding a click event handler $("button").off("click");
5.2 Event Delegation
Event delegation allows you to handle events for elements that may not exist when the page initially loads. Instead of binding event handlers to individual elements, you can attach a handler to a parent element and capture events that bubble up. This is especially useful for dynamically generated content. Here’s an example:
javascript // Event delegation for dynamically generated elements $("#container").on("click", "button", function() { // Handle the click event for all buttons inside the container });
5.3 Common Event Handlers
jQuery simplifies the usage of common event handlers, such as click, mouseover, keydown, and more. These handlers help you respond to user interactions effectively. Here’s an example:
javascript // Handling the click event $("button").click(function() { // Handle the click event }); // Handling the mouseover event $("#image").mouseover(function() { // Handle the mouseover event }); // Handling the keydown event $("input").keydown(function(event) { // Handle the keydown event });
Conclusion
jQuery’s key functionality for DOM manipulation provides developers with a powerful toolset to create dynamic and interactive web applications. By mastering the art of DOM manipulation with jQuery, you can enhance user experiences and streamline development workflows. So, dive in, experiment with the code samples provided, and unlock the full potential of jQuery for DOM manipulation. Happy coding!
Table of Contents
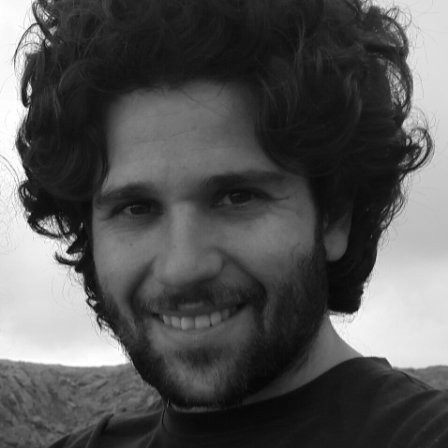
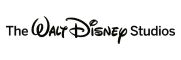