Advanced DOM Traversal Techniques with jQuery
When it comes to manipulating and interacting with the Document Object Model (DOM) of a web page, jQuery has long been a popular choice among frontend developers. Its intuitive syntax and powerful capabilities make it an excellent tool for enhancing user interfaces and creating dynamic web experiences. In this article, we’ll dive into advanced DOM traversal techniques using jQuery, exploring methods that allow you to efficiently navigate and manipulate the DOM structure. Let’s uncover the hidden potential of jQuery’s traversal functions.
Table of Contents
1. Understanding DOM Traversal
1.1. What is DOM Traversal?
DOM traversal refers to the process of navigating through the hierarchical structure of a web page’s DOM to access and manipulate specific elements. It involves moving from one element to another, whether they are parents, children, siblings, or ancestors, using various methods and techniques.
1.2. Why Choose jQuery for DOM Traversal?
While modern JavaScript provides native methods for DOM manipulation, jQuery offers a more concise and often more intuitive syntax. It abstracts away browser inconsistencies, simplifying complex traversal tasks. With jQuery, you can write efficient and readable code that targets specific elements without needing to worry about varying browser implementations.
2. Common DOM Traversal Methods
2.1. find(): Locating Elements Within Another Element
The find() method in jQuery is incredibly useful when you want to search for specific elements within a given container. It performs a deep search through the descendants of the selected element and returns all elements that match the provided selector.
javascript // Example: Finding all <span> elements within a <div> container const spanElements = $('div.container').find('span'); closest(): Finding Ancestors
The closest() method helps you locate the nearest ancestor that matches a given selector. It traverses up the DOM tree from the selected element and stops as soon as it finds a matching ancestor.
javascript // Example: Finding the closest <div> ancestor with class 'highlight' const closestDiv = $('span.target').closest('div.highlight'); siblings(): Navigating Through Siblings
When you need to navigate through siblings of an element, the siblings() method comes in handy. It returns all sibling elements that share the same parent as the selected element.
javascript // Example: Selecting all sibling <li> elements of a clicked <li> $('li').on('click', function() { const siblings = $(this).siblings(); // Manipulate the siblings as needed });
3. Filtering and Chaining
3.1. Refining Selections with filter()
The filter() method allows you to refine a selection by applying a filter based on a specified criteria. It reduces the set of matched elements to those that meet the condition.
javascript // Example: Filtering <li> elements to only include those with 'completed' class const completedItems = $('li').filter('.completed');
3.2. Chaining Multiple Traversal Methods
One of the strengths of jQuery is its ability to chain multiple methods together. This allows you to perform complex traversals in a concise manner.
javascript // Example: Chaining methods to find the next <input> element within the next <div> const nextInput = $('div.container').find('span.target').closest('div').next('div').find('input');
4. Traversing the DOM Tree
4.1. parent() and parents(): Ascending the DOM Hierarchy
The parent() method selects the immediate parent element of the current element. Meanwhile, the parents() method lets you select all ancestor elements that match a given selector.
javascript // Example: Getting the parent <div> of a clicked button $('button').on('click', function() { const parentDiv = $(this).parent('div'); });
4.2. children(): Descending to Child Elements
The children() method returns all direct child elements of the selected element. This is useful for targeting specific child elements within a container.
javascript // Example: Selecting all direct child <li> elements of a <ul> const childItems = $('ul.list').children('li');
5. Advanced Traversal Techniques
5.1. next() and prev(): Moving Among Siblings
The next() method selects the immediately following sibling of the current element, while prev() selects the immediately preceding sibling.
javascript // Example: Selecting the next <div> after a clicked <button> $('button').on('click', function() { const nextDiv = $(this).next('div'); });
5.2. not(): Excluding Elements from Selection
The not() method helps you exclude elements from a selection based on a provided selector.
javascript // Example: Selecting all <li> elements except those with class 'completed' const incompleteItems = $('li').not('.completed');
5.3. eq(): Selecting Elements by Index
The eq() method lets you select elements by their index within the matched set.
javascript // Example: Selecting the second <li> element within a <ul> const secondItem = $('ul.list').find('li').eq(1);
6. Handling Dynamic Content
6.1. Event Delegation: Binding Events to Dynamic Elements
When working with dynamically added elements, event delegation ensures that events are properly handled, even for elements that were not present when the page loaded.
javascript // Example: Using event delegation to handle clicks on dynamically added <button> elements $('ul.list').on('click', 'button', function() { // Handle the click event });
6.2. Utilizing on() for Dynamic Content
The on() method provides a way to bind event handlers to both existing and dynamically added elements. It offers flexibility and efficiency when dealing with changing DOM structures.
javascript // Example: Binding a click event to all present and future <a> elements $('body').on('click', 'a', function() { // Handle the click event });
Conclusion
In this exploration of advanced DOM traversal techniques using jQuery, we’ve covered a range of powerful methods and concepts that can greatly enhance your frontend development skills. Understanding how to efficiently navigate the DOM tree, manipulate elements, and work with dynamic content is essential for creating interactive and user-friendly web applications. By leveraging the capabilities of jQuery, you can streamline your code and build more engaging user experiences. Whether you’re a seasoned developer or just starting out, mastering these advanced DOM traversal techniques will undoubtedly be a valuable asset in your toolkit.
Table of Contents
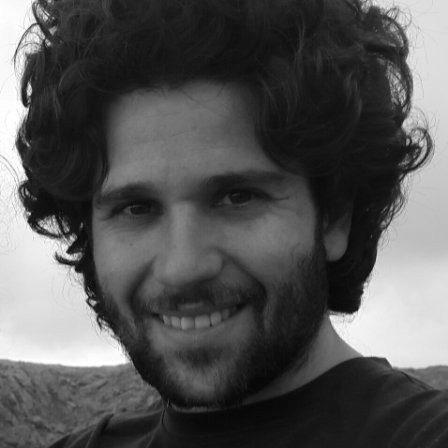
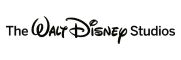