Implementing Drag and Drop File Uploads with jQuery and HTML5
In the ever-evolving landscape of web development, user experience continues to be a critical factor in designing successful websites and applications. One area where this principle holds true is file uploads. Traditional file input fields often feel clunky and outdated, detracting from the overall user experience. Enter drag and drop file uploads – a seamless and intuitive way to enhance your users’ interaction with your website. In this tutorial, we’ll explore how to implement drag and drop file uploads using the power of jQuery and the native HTML5 drag-and-drop API.
Table of Contents
1. Elevating user experience with drag and drop file uploads
1.1. Introduction
The need for improved file upload experiences
File uploads are an essential part of many websites and applications. Whether you’re allowing users to share images, documents, or any other type of file, providing a smooth and intuitive file upload experience can significantly impact user satisfaction. Traditional file input fields lack the interactivity and user-friendliness that modern web users expect.
1.2. Benefits of drag and drop file uploads
Drag and drop file uploads offer several advantages over conventional file input methods. They allow users to easily select and upload files by dragging them from their local file system and dropping them onto a designated area on the webpage. This method eliminates the need to navigate through file directories, making the process faster and more engaging.
Furthermore, drag and drop interfaces provide visual feedback as the file is dragged, creating a sense of direct manipulation. This tactile interaction mimics real-world actions, enhancing the user experience and making the file upload process more intuitive.
2. Getting Started
2.1. Setting up your HTML structure
To begin implementing drag and drop file uploads, ensure you have a basic HTML structure in place. Create a container where users can drag and drop files, and include placeholders for displaying file previews and upload progress. Here’s a simple example:
html <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Drag and Drop File Uploads</title> </head> <body> <div id="drag-drop-area"> <p>Drag and drop files here</p> <div id="file-previews"></div> <div id="upload-progress"></div> </div> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> <script src="script.js"></script> </body> </html>
2.2. Including jQuery and necessary libraries
Before you proceed, make sure to include the jQuery library in your HTML file. You can either download it and host it on your server or use a content delivery network (CDN) link, as shown in the example above. Additionally, we’ll be using jQuery UI for some visual enhancements, so include that as well.
In the next section, we’ll dive into the actual implementation of drag and drop functionality.
3. Implementing Drag and Drop
Understanding the HTML5 drag-and-drop API
The HTML5 drag-and-drop API provides the foundation for creating drag and drop interactions on your webpage. This API consists of several events that are fired during different stages of the drag-and-drop process:
- dragstart: Fired when a draggable element is first dragged.
- drag: Fired continuously as the element is being dragged.
- dragenter: Fired when a draggable element enters a drop target.
- dragover: Fired when a draggable element is over a drop target.
- dragleave: Fired when a draggable element leaves a drop target.
- drop: Fired when a draggable element is dropped onto a drop target.
- dragend: Fired when the drag operation is completed (whether successful or not).
- Creating the drag-and-drop area
Let’s implement a basic drag-and-drop area using jQuery. In your script.js file, add the following code:
javascript $(document).ready(function () { const dragDropArea = document.getElementById('drag-drop-area'); dragDropArea.addEventListener('dragover', (e) => { e.preventDefault(); dragDropArea.classList.add('drag-over'); }); dragDropArea.addEventListener('dragleave', () => { dragDropArea.classList.remove('drag-over'); }); dragDropArea.addEventListener('drop', (e) => { e.preventDefault(); dragDropArea.classList.remove('drag-over'); const files = e.dataTransfer.files; handleDroppedFiles(files); }); function handleDroppedFiles(files) { // Handle dropped files here } });
In this code, we’re adding event listeners for the dragover, dragleave, and drop events to the drag-drop-area element. When a file is dragged over the area, we prevent the default behavior to allow dropping and add a CSS class to indicate that the area is a valid drop target. When the file is dropped, we remove the CSS class and call the handleDroppedFiles function, passing in the dropped files.
4. Handling File Uploads
4.1. Capturing dropped files with jQuery
Now that we’re capturing dropped files, let’s proceed to handle these files. In the handleDroppedFiles function, we can create file previews and prepare for uploading. Modify your script.js file as follows:
javascript // Inside the handleDroppedFiles function function handleDroppedFiles(files) { const filePreviews = document.getElementById('file-previews'); for (const file of files) { const preview = document.createElement('div'); preview.className = 'file-preview'; preview.textContent = file.name; filePreviews.appendChild(preview); } }
In this code snippet, we’re creating a div element for each dropped file and adding a class and the file name as content. This will give users a visual representation of the files they’ve selected for upload.
4.2. Displaying previews of uploaded files
To enhance the user experience further, let’s add the ability to display actual previews of image files. Update the handleDroppedFiles function as follows:
javascript // Inside the handleDroppedFiles function function handleDroppedFiles(files) { const filePreviews = document.getElementById('file-previews'); for (const file of files) { const preview = document.createElement('div'); preview.className = 'file-preview'; preview.textContent = file.name; if (file.type.startsWith('image/')) { const image = document.createElement('img'); image.src = URL.createObjectURL(file); preview.appendChild(image); } filePreviews.appendChild(preview); } }
Now, if the dropped file is an image, we create an img element and set its src attribute to a URL created using URL.createObjectURL(file). This URL generates a temporary URL for the file, allowing us to display the image as a preview.
5. Uploading Files
5.1. Preparing for server-side upload
Before we proceed with uploading the files, ensure you have a server-side script or endpoint ready to handle file uploads. For this example, let’s assume you have an endpoint at upload.php that can handle the uploaded files.
5.2. Sending files using AJAX and FormData
To upload the selected files to the server, we’ll use AJAX and the FormData API. Update your script.js file to include the following code:
javascript // Inside the handleDroppedFiles function function handleDroppedFiles(files) { // ... (previous code) const uploadProgress = document.getElementById('upload-progress'); const formData = new FormData(); for (const file of files) { formData.append('files[]', file); } $.ajax({ url: 'upload.php', type: 'POST', data: formData, processData: false, contentType: false, xhr: function () { const xhr = new XMLHttpRequest(); xhr.upload.addEventListener('progress', (e) => { const percent = (e.loaded / e.total) * 100; uploadProgress.innerHTML = `Uploading: ${percent.toFixed(2)}%`; }); return xhr; }, success: function () { uploadProgress.innerHTML = 'Upload complete!'; }, error: function () { uploadProgress.innerHTML = 'Upload failed'; } }); }
In this code, we’re using the FormData API to create a formData object and appending each file to it with the key ‘files[]’. We then send the formData object using an AJAX POST request to the upload.php endpoint.
The xhr function is used to customize the XMLHttpRequest object. We’ve added an event listener for the progress event to track the upload progress. The success and error callbacks provide feedback to the user based on the upload result.
6. Adding Feedback and Enhancements
6.1. Providing user feedback during upload
To provide real-time feedback to the user during file upload, we’ve already implemented a progress bar in the previous step. However, you can enhance this feedback further by including additional UI elements or animations to make the upload process more engaging.
6.2. Handling errors gracefully
Error handling is a crucial aspect of any user-centric design. In the example code above, we’ve added an error callback to display a message in case the upload fails. Depending on your application’s requirements, you can expand error handling to provide more context about the failure and possible solutions.
Conclusion
Implementing drag and drop file uploads using jQuery and HTML5 offers a significant improvement to the user experience of file uploads on your website or application. By leveraging the HTML5 drag-and-drop API and jQuery’s capabilities, you can create a seamless and intuitive way for users to upload files with ease. This approach not only enhances user engagement but also showcases your commitment to providing a modern and user-friendly interface.
In this tutorial, we’ve covered the fundamentals of implementing drag and drop file uploads, from setting up the HTML structure to handling file previews and server-side uploads. As you continue to refine your web development skills, remember that user experience should remain a top priority, and drag and drop functionality is just one example of how thoughtful design can elevate your projects to new heights. So go ahead, experiment with drag and drop file uploads, and watch as your users appreciate the improved upload experience.
Table of Contents
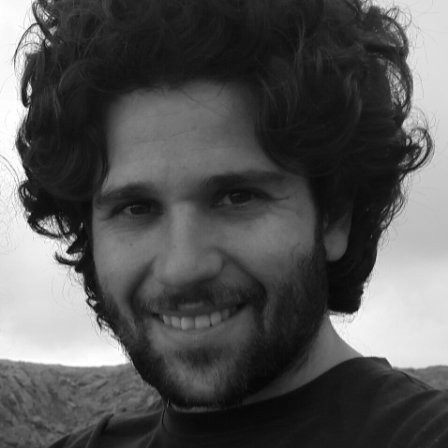
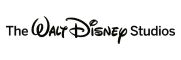