Drag and Drop Functionality with jQuery: Building Intuitive Interfaces
In today’s fast-paced digital world, intuitive user interfaces are crucial for creating a positive user experience. One of the most popular and effective ways to achieve this is through drag and drop functionality. By allowing users to interact with elements by dragging and dropping them, you can enhance the usability and interactivity of your web applications.
Table of Contents
In this blog post, we will explore how to implement drag and drop functionality using jQuery, a powerful JavaScript library that simplifies HTML document traversal and manipulation. We’ll cover the basic concepts, essential techniques, and provide code samples to help you get started with building intuitive interfaces that engage your users.
1. Understanding Drag and Drop
Before diving into the implementation, let’s briefly understand the concept of drag and drop. Drag and drop is a user interface interaction pattern that allows users to click and “grab” an element, move it to a different location, and “drop” it there. This pattern is commonly used for tasks like reordering items, file uploads, and organizing content.
When implementing drag and drop, there are typically three main events involved:
- Drag Start: This event occurs when the user starts dragging an element. It is the initial point of the interaction, where you can capture data about the dragged element.
- Drag: The drag event is triggered as the user moves the element around. During this phase, you can update the visual representation of the dragged element and perform any necessary calculations.
- Drag End: When the user releases the mouse button, the dragend event is triggered. At this point, you can perform any final actions, like dropping the element into a specific target or resetting the interface.
Now that we have a basic understanding of drag and drop, let’s see how to implement it using jQuery.
2. Setting Up the Environment
First, make sure you have jQuery included in your project. You can either download the library and host it locally or use a Content Delivery Network (CDN) to access it. Here’s an example of including jQuery using a CDN:
html <head> <!-- Other meta tags and links --> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> </head>
With jQuery set up, we can move on to the next step.
3. Creating the HTML Structure
For this demonstration, let’s create a simple to-do list application with drag and drop functionality. We’ll have two columns: “Pending Tasks” and “Completed Tasks.” Each task will be represented as a list item (<li>). Here’s the HTML structure:
html <div class="todo-list"> <h2>Pending Tasks</h2> <ul id="pending-tasks" class="task-list"> <li draggable="true">Task 1</li> <li draggable="true">Task 2</li> <li draggable="true">Task 3</li> </ul> <h2>Completed Tasks</h2> <ul id="completed-tasks" class="task-list"> <li draggable="true">Task 4</li> <li draggable="true">Task 5</li> </ul> </div>
In this example, we have two lists (<ul>) with draggable list items (<li>). The draggable=”true” attribute is essential for enabling the drag and drop functionality on these elements.
4. Styling the Interface
Now, let’s add some basic CSS to style our to-do list application. We want to make the items look like cards and add some spacing between the lists. Here’s a simple CSS snippet:
css .todo-list { display: flex; justify-content: space-around; } .task-list { list-style-type: none; padding: 10px; border: 1px solid #ccc; border-radius: 5px; width: 200px; min-height: 100px; } li { background-color: #f9f9f9; padding: 8px; margin-bottom: 5px; border: 1px solid #ddd; border-radius: 3px; cursor: grab; }
With the HTML and CSS in place, we can now focus on adding the jQuery code to implement the drag and drop functionality.
5. Implementing Drag and Drop with jQuery
We’ll use jQuery to handle the drag and drop events and update the task’s status between the “Pending Tasks” and “Completed Tasks” lists.
First, let’s create a function to handle the dragstart event. This event will be triggered when the user starts dragging a task. We’ll store the data of the dragged task using jQuery’s data() method:
js $(document).on("dragstart", "li", function (event) { // Store the ID of the dragged task const taskId = event.target.id; event.originalEvent.dataTransfer.setData("text", taskId); });
Next, we need to handle the dragover event to allow the drop. By default, elements cannot be dropped into other elements. We need to prevent the default behavior in this case:
js $(document).on("dragover", "ul", function (event) { event.preventDefault(); });
Now, we can handle the drop event to move the task from the “Pending Tasks” list to the “Completed Tasks” list or vice versa:
js $(document).on("drop", "ul", function (event) { event.preventDefault(); // Get the ID of the dragged task from the data transfer object const taskId = event.originalEvent.dataTransfer.getData("text"); const draggedTask = $("#" + taskId); // Check if the drop target is the "Pending Tasks" or "Completed Tasks" list const targetListId = event.currentTarget.id; if (targetListId === "pending-tasks") { $("#pending-tasks").append(draggedTask); } else if (targetListId === "completed-tasks") { $("#completed-tasks").append(draggedTask); } });
Conclusion
Congratulations! You’ve learned how to implement drag and drop functionality using jQuery. By applying this knowledge, you can build intuitive interfaces that allow users to interact with your web applications seamlessly.
In this blog post, we covered the basic concepts of drag and drop, set up the development environment, created the HTML structure, styled the interface, and implemented the drag and drop functionality with jQuery. Remember, the possibilities are endless with drag and drop, and you can tailor it to fit various use cases.
Now it’s time to put your skills into practice and experiment with drag and drop in your own projects. As you delve deeper into this topic, you’ll discover more ways to enhance user experiences and create engaging interfaces that leave a lasting impression.
Happy coding!
Table of Contents
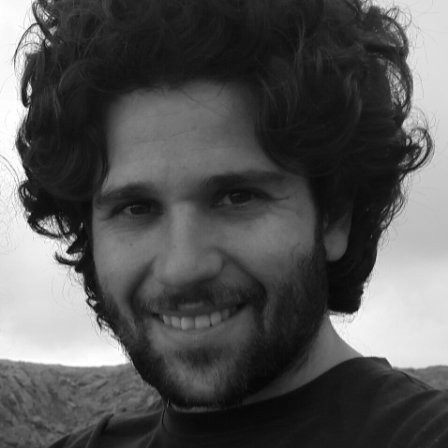
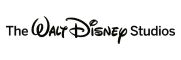