Creating Dynamic Content Filters with jQuery
In today’s fast-paced digital world, websites often contain a plethora of content that users need to navigate through. Whether it’s an online portfolio showcasing various projects or an e-commerce platform with numerous products, providing users with efficient ways to filter and sort content is crucial. Enter dynamic content filters – a powerful tool that enhances user experience by allowing them to quickly access the specific content they’re interested in. In this tutorial, we’ll delve into the process of creating dynamic content filters using jQuery, a popular JavaScript library.
Table of Contents
1. Understanding the Importance of Dynamic Content Filters
Before we dive into the technical aspects, let’s explore why dynamic content filters are essential for modern websites. Imagine you’re browsing an online clothing store with hundreds of products. Without content filters, finding a specific item in your preferred size, color, or style would be like searching for a needle in a haystack. Dynamic content filters simplify this process by enabling users to narrow down their search criteria, making their experience on your website smoother and more enjoyable.
2. Prerequisites: What You Need to Get Started
Before we jump into the coding, make sure you have the following prerequisites in place:
- HTML Structure: You should have a structured HTML layout that includes the content you want to filter. This could be a grid of products, a gallery of images, or any other content you want users to filter through.
- jQuery Library: Make sure you have the jQuery library included in your project. You can either download it and host it locally or use a Content Delivery Network (CDN) to include it in your HTML.
- Basic CSS: A little bit of CSS knowledge will come in handy to style your content filters and make them visually appealing.
3. Step-by-Step Guide to Creating Dynamic Content Filters
Now that we’ve covered the basics, let’s get our hands dirty and start creating dynamic content filters using jQuery.
3.1. Setting Up the HTML Structure
Begin by structuring your HTML to include the content you want to filter. This could be a series of div elements containing images, product descriptions, or any other relevant information. Assign appropriate classes and data attributes to these elements to help with filtering later on.
html <div class="content-filter"> <button data-filter="all">All</button> <button data-filter="category1">Category 1</button> <button data-filter="category2">Category 2</button> <!-- Add more buttons for different categories --> </div> <div class="content-items"> <div class="item" data-category="category1"> <!-- Content for category 1 --> </div> <div class="item" data-category="category2"> <!-- Content for category 2 --> </div> <!-- Add more items with respective categories --> </div>
3.2. Adding CSS Styles
Style your content filter buttons and content items to ensure they’re visually appealing and user-friendly. You can use CSS to give the buttons a distinct appearance and hide/show content items based on their categories.
css /* Style for content filter buttons */ .content-filter button { background-color: #3498db; color: white; border: none; padding: 8px 16px; margin: 5px; cursor: pointer; border-radius: 4px; } /* Style for active button */ .content-filter button.active { background-color: #e74c3c; } /* Hide content items by default */ .item { display: none; } /* Show only the selected category's items */ .item[data-category="category1"], .item[data-category="category2"], /* Add more categories here */ { display: block; }
3.3. Implementing jQuery for Dynamic Filtering
Now comes the heart of our dynamic content filtering – jQuery! We’ll write JavaScript code to handle the button clicks and display the relevant content items.
javascript $(document).ready(function() { // Initially show all items $(".item").show(); // Handle button click events $(".content-filter button").click(function() { // Remove 'active' class from all buttons $(".content-filter button").removeClass("active"); // Add 'active' class to the clicked button $(this).addClass("active"); // Get the filter category from the button's data attribute var filterCategory = $(this).attr("data-filter"); // Show or hide items based on the selected category if (filterCategory === "all") { $(".item").show(); } else { $(".item").hide(); $(".item[data-category='" + filterCategory + "']").show(); } }); });
3.4. Testing and Fine-Tuning
With the HTML structure, CSS, and jQuery code in place, you can now open your HTML file in a web browser and test the dynamic content filtering functionality. Click on different buttons to see how the content items are filtered based on their categories. If you encounter any issues, remember to check your console for error messages and double-check your code for typos.
Conclusion
In this tutorial, we’ve explored the significance of dynamic content filters in enhancing user experience on websites with substantial amounts of content. By providing users with the ability to filter and sort content based on their preferences, you can significantly improve their browsing experience. We walked through the step-by-step process of creating dynamic content filters using jQuery, from setting up the HTML structure to writing the necessary CSS and JavaScript code.
With dynamic content filters, you can empower your users to find the content that matters most to them quickly and efficiently. Whether you’re building an e-commerce site, a portfolio showcase, or a blog with various categories, implementing dynamic content filters will undoubtedly make your website more user-friendly and engaging. So go ahead, experiment with the concepts discussed in this tutorial, and take your website’s user experience to the next level!
Table of Contents
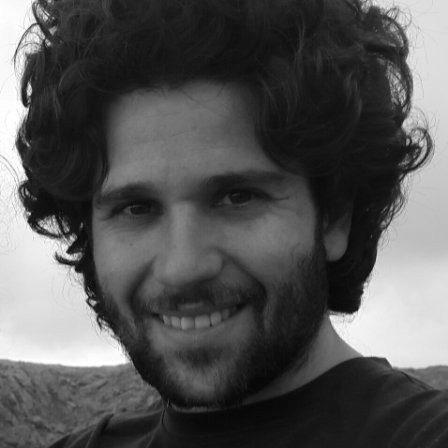
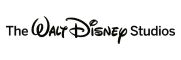