Dynamic Form Validation with jQuery
In today’s digital era, web forms play a crucial role in capturing user data and enabling various online interactions. Whether it’s a sign-up form, a contact form, or a search box, effective form validation is essential to ensure the accuracy and integrity of the data submitted by users. Dynamic form validation with jQuery offers a powerful and flexible way to validate form inputs in real-time, providing instant feedback to users and enhancing their overall experience. In this blog post, we’ll explore how to implement dynamic form validation using jQuery, along with code samples and best practices.
1. Why Dynamic Form Validation?
Traditional form validation typically occurs after a user submits a form. The server then processes the data and returns validation errors if any. While this approach can work, it often leads to a suboptimal user experience. Users may have to fill out an entire form, only to find out they made a mistake, leading to frustration and abandonment of the form.
Dynamic form validation, on the other hand, validates user inputs as they fill out the form. It provides instant feedback, highlighting errors in real-time, thus allowing users to correct their mistakes immediately. This way, users can submit error-free data, reducing the chances of form submission errors and improving overall data quality.
2. Getting Started with jQuery
Before we dive into dynamic form validation, let’s ensure we have jQuery set up in our project. If you haven’t included jQuery yet, you can do so by adding the following script tag to your HTML file, preferably before the closing </body> tag:
html <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
With jQuery in place, we’re ready to start implementing dynamic form validation.
3. Basic Form Structure
For demonstration purposes, let’s consider a simple registration form with the following fields:
- Name (text input)
- Email (email input)
- Password (password input)
- Confirm Password (password input)
- Age (number input)
- Terms and Conditions (checkbox)
The goal is to validate each input in real-time, giving users instant feedback about their input errors.
4. Implementing Dynamic Form Validation
4.1. Real-time Input Event Binding
To perform real-time validation, we need to bind input event handlers to each form field. These handlers will be triggered whenever a user interacts with the corresponding input element. Let’s take a look at the following jQuery code that accomplishes this:
js $(document).ready(function() { // Select all the input fields that need validation const $nameInput = $('#name'); const $emailInput = $('#email'); const $passwordInput = $('#password'); const $confirmPasswordInput = $('#confirm-password'); const $ageInput = $('#age'); const $termsCheckbox = $('#terms'); // Bind input event handlers $nameInput.on('input', validateName); $emailInput.on('input', validateEmail); $passwordInput.on('input', validatePassword); $confirmPasswordInput.on('input', validateConfirmPassword); $ageInput.on('input', validateAge); $termsCheckbox.on('change', validateTerms); });
4.2. Implementing Validation Functions
Now that we have our input event handlers set up, let’s implement the validation functions for each input field. These functions will be responsible for checking the input values and providing feedback to the user if there are any errors. Here are the functions for each input field:
1. Name Validation
js function validateName() { const $nameInput = $('#name'); const name = $nameInput.val().trim(); if (name === '') { showError($nameInput, 'Name is required.'); } else { hideError($nameInput); } }
2. Email Validation
js function validateEmail() { const $emailInput = $('#email'); const email = $emailInput.val().trim(); const emailRegex = /^[^\s@]+@[^\s@]+\.[^\s@]+$/; if (email === '') { showError($emailInput, 'Email is required.'); } else if (!emailRegex.test(email)) { showError($emailInput, 'Invalid email format.'); } else { hideError($emailInput); } }
3. Password Validation
js function validatePassword() { const $passwordInput = $('#password'); const password = $passwordInput.val(); if (password === '') { showError($passwordInput, 'Password is required.'); } else if (password.length < 8) { showError($passwordInput, 'Password must be at least 8 characters long.'); } else { hideError($passwordInput); } }
4. Confirm Password Validation
js function validateConfirmPassword() { const $confirmPasswordInput = $('#confirm-password'); const confirmPassword = $confirmPasswordInput.val(); const password = $('#password').val(); if (confirmPassword === '') { showError($confirmPasswordInput, 'Please confirm your password.'); } else if (confirmPassword !== password) { showError($confirmPasswordInput, 'Passwords do not match.'); } else { hideError($confirmPasswordInput); } }
5. Age Validation
js function validateAge() { const $ageInput = $('#age'); const age = $ageInput.val(); if (age === '') { showError($ageInput, 'Age is required.'); } else if (isNaN(age) || age <= 0) { showError($ageInput, 'Please enter a valid age.'); } else { hideError($ageInput); } }
6. Terms and Conditions Validation
js function validateTerms() { const $termsCheckbox = $('#terms'); if (!$termsCheckbox.prop('checked')) { showError($termsCheckbox, 'You must accept the terms and conditions.'); } else { hideError($termsCheckbox); } }
4.3. Displaying Error Messages
To provide feedback to users, we need to create functions to show and hide error messages for each input field. Let’s define these functions as follows:
js function showError($input, errorMessage) { hideError($input); // Hide any existing error message $input.addClass('error'); const $errorSpan = $('<span>').addClass('error-message').text(errorMessage); $input.after($errorSpan); } function hideError($input) { $input.removeClass('error'); $input.next('.error-message').remove(); }
In the code above, the showError function adds a red border around the input field and appends an error message below it. The hideError function, as the name suggests, removes the error styling and message from the input field.
4.4. Applying CSS Styling
To make the error messages visually appealing, let’s apply some basic CSS styling to our form:
css /* CSS Styles for error messages */ .error { border: 2px solid #f00; } .error-message { color: #f00; font-size: 12px; margin-top: 5px; }
With this CSS, whenever an input field fails validation, a red border will be added around the field, and a small error message will be displayed below it.
5. Putting It All Together
Now that we have all the pieces in place, let’s see our dynamic form validation in action. Below is the complete HTML code for the registration form:
html <!DOCTYPE html> <html> <head> <title>Dynamic Form Validation with jQuery</title> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> <style> /* CSS Styles for error messages */ .error { border: 2px solid #f00; } .error-message { color: #f00; font-size: 12px; margin-top: 5px; } </style> </head> <body> <h1>Registration Form</h1> <form id="registration-form"> <div> <label for="name">Name:</label> <input type="text" id="name" name="name"> </div> <div> <label for="email">Email:</label> <input type="email" id="email" name="email"> </div> <div> <label for="password">Password:</label> <input type="password" id="password" name="password"> </div> <div> <label for="confirm-password">Confirm Password:</label> <input type="password" id="confirm-password" name="confirm-password"> </div> <div> <label for="age">Age:</label> <input type="number" id="age" name="age"> </div> <div> <input type="checkbox" id="terms" name="terms"> <label for="terms">I agree to the terms and conditions</label> </div> <button type="submit">Submit</button> </form> <script> // jQuery code for dynamic form validation // ... // The code snippets provided earlier for real-time input event binding, // validation functions, and displaying error messages should be placed here. // ... </script> </body> </html>
Once you’ve added the above code to an HTML file, open it in a web browser. As you interact with the form, you’ll notice that validation errors are displayed in real-time, helping you correct any mistakes before submitting the form.
Conclusion
Dynamic form validation with jQuery is a powerful technique that can significantly enhance user experience and ensure the accuracy of data submitted through web forms. By providing real-time feedback, users can easily correct errors, reducing frustration and improving overall data quality.
In this blog post, we’ve explored how to implement dynamic form validation using jQuery. We learned how to bind input event handlers, create validation functions, and display error messages to users. By combining these techniques, you can build robust and user-friendly forms that deliver a seamless experience to your website visitors.
Remember that form validation is just one part of creating great user experiences. Always strive to implement accessibility best practices, and consider using server-side validation to ensure data integrity. With these practices in mind, you’ll create forms that users love to fill out, leading to increased engagement and satisfaction with your website. Happy coding!
Table of Contents
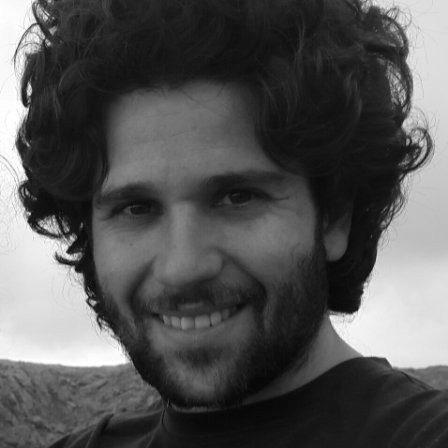
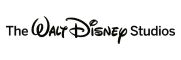