Building Dynamic Sliders with jQuery UI Slider
In the world of web development, interactivity and user engagement play a crucial role in creating a successful user experience. One popular way to achieve this is by incorporating dynamic sliders into your website. These sliders allow users to select values within a range by dragging a handle along a track, making them perfect for inputting numeric values, filtering results, or adjusting settings. In this tutorial, we’ll delve into the world of building dynamic sliders using the jQuery UI Slider library. We’ll explore its features, learn how to customize its appearance, and understand how to integrate it into your web projects seamlessly.
Table of Contents
1. Understanding the jQuery UI Slider
The jQuery UI Slider is a versatile and user-friendly component that provides an interactive way for users to input a value or select a range. It offers a smooth sliding animation, a range of configuration options, and the ability to handle events and updates dynamically. With its flexibility and ease of integration, the jQuery UI Slider has become a staple in web development when it comes to implementing dynamic user interfaces.
2. Getting Started: Setting Up the Environment
Before we dive into building dynamic sliders, it’s important to set up our development environment. Make sure you have a basic understanding of HTML, CSS, and jQuery before proceeding. Here’s a simple example of how you can structure your HTML file:
html <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link rel="stylesheet" href="https://code.jquery.com/ui/1.12.1/themes/base/jquery-ui.css"> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> <script src="https://code.jquery.com/ui/1.12.1/jquery-ui.js"></script> <title>Dynamic Sliders with jQuery UI</title> </head> <body> <div id="slider"></div> </body> </html>
3. Creating a Basic Slider
Let’s start by creating a simple dynamic slider using the jQuery UI Slider. In the provided HTML file, we have a div element with the ID “slider.” We will initialize the slider using JavaScript:
javascript $(document).ready(function() { $("#slider").slider(); });
With these few lines of code, you have a functional slider on your webpage. However, it’s currently not interactive, and you cannot retrieve the selected value. Let’s enhance the slider’s functionality.
4. Adding Interactivity and Displaying Selected Values
To make the slider interactive and display the selected value, we need to utilize the slider’s configuration options and event handling capabilities. Modify the JavaScript code as follows:
javascript $(document).ready(function() { $("#slider").slider({ value: 50, // Initial value min: 0, // Minimum value max: 100, // Maximum value step: 5, // Increment step slide: function(event, ui) { $("#selected-value").text(ui.value); // Display selected value } }); });
In this code snippet, we’ve added configuration options like min, max, and step to define the slider’s behavior. The slide event is used to update a designated element with the currently selected value. Make sure you have an HTML element with the ID “selected-value” to display the value:
html <p>Selected Value: <span id="selected-value">50</span></p>
5. Customizing Slider Appearance
While the default appearance of the jQuery UI Slider is functional, you might want to customize it to match your website’s design. The library provides options for customizing various aspects, such as the slider’s range, handle, and orientation.
5.1. Changing Handle Appearance
You can change the appearance of the slider handle using the classes option. Add the following code to your JavaScript to create a custom handle style:
javascript $(document).ready(function() { $("#slider").slider({ // ... classes: { "ui-slider": "custom-slider", "ui-slider-handle": "custom-handle" }, // ... }); });
Then, define the CSS styles for the custom classes:
css .custom-slider .custom-handle { background-color: #3498db; border: 2px solid #2980b9; width: 20px; height: 20px; }
5.2. Vertical Slider
By default, the slider is horizontal. If you want to create a vertical slider, simply set the orientation option to “vertical”:
javascript $("#slider").slider({ // ... orientation: "vertical", // ... });
5.3. Range Slider
A range slider allows users to select a range of values. To create a range slider, set the range option to “true”:
javascript $("#slider").slider({ // ... range: true, // ... });
6. Handling Slider Events
The jQuery UI Slider library provides a range of events that you can utilize to enhance interactivity and provide real-time updates. Here’s an example of how you can use the change event to perform an action whenever the slider value changes:
javascript $("#slider").slider({ // ... change: function(event, ui) { console.log("Slider value changed to: " + ui.value); } });
You can also use events like start, slide, and stop to control actions during different phases of slider interaction.
7. Dynamic Slider with Real-time Updates
In many scenarios, you might want to dynamically update other elements on the page based on the slider’s value. For instance, consider a use case where you want to display the corresponding price as the user adjusts a discount slider. Let’s see how this can be achieved:
javascript $(document).ready(function() { $("#discount-slider").slider({ value: 10, min: 0, max: 50, step: 5, slide: function(event, ui) { $("#discount-value").text(ui.value + "%"); updatePrice(ui.value); } }); function updatePrice(discount) { var originalPrice = 100; // Example original price var discountedPrice = originalPrice * (1 - discount / 100); $("#discounted-price").text("$" + discountedPrice.toFixed(2)); } });
In this example, as the user adjusts the discount slider, the corresponding percentage and discounted price are updated in real-time.
Conclusion
Dynamic sliders are powerful tools that enhance user experience and interactivity on websites. With the jQuery UI Slider library, creating these sliders becomes a breeze. We’ve covered the basics of creating a slider, customizing its appearance, handling events, and implementing dynamic real-time updates. Armed with this knowledge, you can take your web development projects to the next level by incorporating engaging and interactive sliders that provide users with seamless and intuitive ways to interact with your content. So go ahead, experiment with the code samples provided, and start building dynamic sliders that captivate your users and add value to your web applications.
Table of Contents
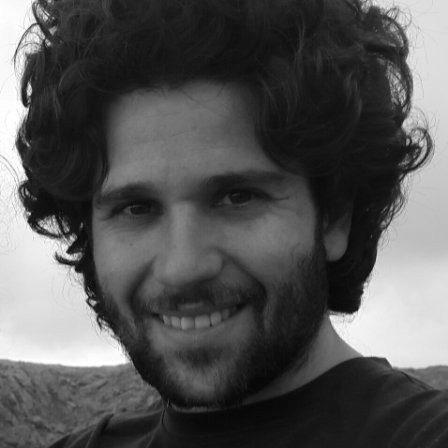
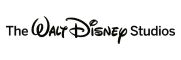