jQuery Effects: Fading, Sliding, and Animating Content
In the dynamic realm of web development, enhancing user experience and engagement is paramount. One powerful way to achieve this is through captivating visual effects that grab attention and create a seamless browsing experience. jQuery, a popular JavaScript library, offers a plethora of tools to achieve these effects effortlessly. In this blog post, we’ll delve into three core jQuery effects – fading, sliding, and animating content – and provide you with valuable insights and practical code examples to implement these effects on your own website.
Table of Contents
1. Understanding jQuery Effects
1.1. What are jQuery Effects?
jQuery effects are pre-defined animations and transitions that can be easily applied to HTML elements using the jQuery library. These effects add a touch of interactivity and dynamism to your web pages, making them more visually appealing and engaging. The beauty of jQuery effects lies in their simplicity – they require minimal coding effort yet yield impressive results.
1.2. Advantages of Using jQuery Effects
- User Engagement: jQuery effects can captivate users’ attention, keeping them engaged and encouraging them to explore your website further.
- Visual Appeal: By incorporating fading, sliding, and animating effects, you can make your website visually appealing and give it a modern touch.
- Smooth Transitions: These effects provide smooth transitions between different states of your website, creating a polished and professional user experience.
- Interactivity: Effects like sliding and animating content can provide interactive elements that respond to user actions, making the website feel more interactive.
2. Fading Content
2.1. The .fadeIn() Method
The .fadeIn() method gradually increases the opacity of selected elements, making them appear to fade in smoothly. This effect is particularly useful when you want to reveal elements gradually, instead of having them abruptly appear on the screen.
javascript $(document).ready(function() { $("#fadeInButton").click(function() { $("#fadeInTarget").fadeIn(); }); });
2.2. The .fadeOut() Method
Conversely, the .fadeOut() method decreases the opacity of selected elements, creating a fading-out effect. This can be useful when you want to gently remove elements from the screen.
javascript $(document).ready(function() { $("#fadeOutButton").click(function() { $("#fadeOutTarget").fadeOut(); }); });
2.3. Customizing Fading Effects
Both .fadeIn() and .fadeOut() methods allow you to specify a duration for the fading effect. For instance:
javascript $("#fadeInTarget").fadeIn(1000); // Fades in over 1000 milliseconds (1 second) $("#fadeOutTarget").fadeOut(500); // Fades out over 500 milliseconds (0.5 seconds)
3. Sliding Content
3.1. The .slideUp() Method
The .slideUp() method smoothly hides elements by sliding them up, reducing their height to zero. This is handy for creating collapsible sections and toggling content visibility.
javascript $(document).ready(function() { $("#slideUpButton").click(function() { $("#slideUpTarget").slideUp(); }); });
3.2. The .slideDown() Method
Conversely, the .slideDown() method expands hidden elements by sliding them down, revealing their content. This is often used to show hidden content on demand.
javascript $(document).ready(function() { $("#slideDownButton").click(function() { $("#slideDownTarget").slideDown(); }); });
3.3. Combining Slide and Fade
You can also combine sliding and fading effects to create a more sophisticated animation. For instance, you might want an element to slide down while simultaneously fading in.
javascript $(document).ready(function() { $("#slideFadeButton").click(function() { $("#slideFadeTarget").slideDown(); $("#slideFadeTarget").fadeIn(); }); });
4. Animating Content
4.1. The .animate() Method
The .animate() method is a versatile tool that allows you to create custom animations by specifying the properties and values you want to change. This method can animate a wide range of properties, including width, height, opacity, and more.
javascript $(document).ready(function() { $("#animateButton").click(function() { $("#animateTarget").animate({ width: "300px", opacity: 0.5, marginLeft: "50px" }); }); });
4.2. Properties and Values
You can animate multiple properties simultaneously, and even provide values with relative changes:
javascript $("#animateTarget").animate({ width: "+=100px", // Increases width by 100px fontSize: "20px" // Changes font size to 20px });
4.3. Easing Functions for Smooth Animation
The .animate() method also allows you to apply easing functions, which control the acceleration and deceleration of the animation. Some common easing options include “swing” and “linear”:
javascript $("#animateTarget").animate({ opacity: 0.2 }, 1000, "swing"); // Fades out with a smooth swinging motion over 1000ms
5. Bringing It All Together
5.1. Creating Interactive Banners
Imagine you want to create an interactive banner that fades in when the page loads and slides up when the user clicks a close button:
html <div id="interactiveBanner" style="display: none;"> <p>Welcome to our website!</p> <button id="closeButton">Close</button> </div> javascript $(document).ready(function() { $("#interactiveBanner").fadeIn(); $("#closeButton").click(function() { $("#interactiveBanner").slideUp(); }); });
5.2. Building Smooth Image Galleries
You can build smooth image galleries by using the .animate() method to create appealing transitions between images:
html <div id="imageGallery"> <img src="image1.jpg" class="galleryImage"> <img src="image2.jpg" class="galleryImage"> <img src="image3.jpg" class="galleryImage"> </div> javascript Copy code $(document).ready(function() { $(".galleryImage").click(function() { $(this).animate({ width: "200px", height: "200px" }); }); });
6. Best Practices for Using jQuery Effects
6.1. Optimize for Performance
While jQuery effects can enhance user experience, overusing them can lead to performance issues. Use effects judiciously and consider optimizing animations for smoothness and speed.
6.2. Ensure Cross-Browser Compatibility
Test your effects on various browsers to ensure consistent behavior. Different browsers might handle animations slightly differently, so be prepared to tweak your code if necessary.
6.3. Use Effects Sparingly and Purposefully
Not every element on your website needs an animation. Use effects to highlight important content or interactions, avoiding unnecessary distractions for users.
Conclusion
In conclusion, jQuery effects offer a fantastic way to make your website stand out with minimal effort. By leveraging fading, sliding, and animating effects, you can create a more immersive and engaging user experience. Incorporating these effects strategically can elevate your website’s aesthetics and usability, leaving a lasting impression on your visitors. So go ahead, experiment with these effects, and watch your web pages come to life!
Table of Contents
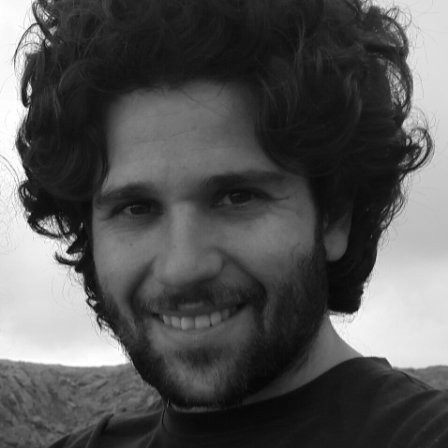
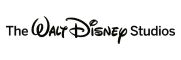