Enhancing User Experience with jQuery UI
In today’s digital landscape, creating an engaging and seamless user experience is essential for any website or web application. Users expect intuitive interfaces, responsive design, and interactive elements that make their journey smooth and enjoyable. One powerful tool that can help achieve this goal is jQuery UI, a curated library of user interface components and widgets built on top of the popular jQuery JavaScript library.
In this blog, we’ll explore the various ways jQuery UI can enhance the user experience on your website. We’ll cover essential aspects such as easy-to-implement widgets, theming options, and interactive features that cater to a wide range of user needs. Let’s dive in and see how jQuery UI can take your web development to the next level.
1. Introduction to jQuery UI
jQuery UI is a free, open-source library that provides a wide range of customizable and ready-to-use user interface elements. It simplifies the process of creating interactive and visually appealing web applications by handling various tasks, such as animation, event handling, and user interactions. The library builds upon jQuery’s core functionality, extending it to include numerous UI components.
2. Quick Setup
To get started with jQuery UI, you need to include both the jQuery library and the jQuery UI library in your project. You can download the libraries and host them on your server or use content delivery networks (CDNs) for quicker access. Here’s a basic HTML template with the necessary script includes:
html <!DOCTYPE html> <html> <head> <title>My jQuery UI Website</title> <link rel="stylesheet" href="https://code.jquery.com/ui/1.12.1/themes/base/jquery-ui.css"> </head> <body> <!-- Your website content here --> <!-- jQuery and jQuery UI script includes --> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> <script src="https://code.jquery.com/ui/1.12.1/jquery-ui.js"></script> </body> </html>
Now that we have the setup in place let’s dive into some of the ways jQuery UI can enhance the user experience on your website.
3. User-Friendly Widgets
One of the primary benefits of jQuery UI is its extensive collection of pre-designed widgets that can be easily integrated into your website. These widgets are designed to be user-friendly, accessible, and customizable according to your project’s needs.
3.1 Accordion
An accordion allows you to organize content into collapsible sections, making it ideal for FAQs, product descriptions, or any content with hierarchical information. Let’s see an example of how to create a simple accordion using jQuery UI:
html <div id="accordion"> <h3>Section 1</h3> <div> <p>Content of section 1 goes here.</p> </div> <h3>Section 2</h3> <div> <p>Content of section 2 goes here.</p> </div> <h3>Section 3</h3> <div> <p>Content of section 3 goes here.</p> </div> </div> <script> $(function() { $("#accordion").accordion(); }); </script>
With this code, the accordion widget will be applied to the accordion div, creating a collapsible section for each h3 element.
3.2 Datepicker
Datepickers are a common element in web forms for selecting dates easily. jQuery UI provides a highly customizable datepicker widget that improves the user experience when choosing dates:
html <input type="text" id="datepicker"> <script> $(function() { $("#datepicker").datepicker(); }); </script>
This code snippet will initialize the datepicker widget for the input field with the id datepicker.
3.3 Autocomplete
Autocomplete is a powerful feature to assist users while filling out forms or searching for content on your website. jQuery UI offers an autocomplete widget that provides suggestions based on user input:
html <input type="text" id="searchInput"> <script> $(function() { const availableTags = ["Apple", "Banana", "Cherry", "Grapes", "Orange", "Pineapple"]; $("#searchInput").autocomplete({ source: availableTags }); }); </script>
In this example, the autocomplete widget will suggest fruits from the availableTags array as the user types in the input field.
4. Custom Theming
A consistent and visually appealing design is crucial for any website. With jQuery UI, you can easily customize the look and feel of the widgets to match your website’s branding or theme. jQuery UI provides theming support through ThemeRoller, a web application that lets you design and download custom themes.
4.1 Using a Custom Theme
After creating or selecting a theme from ThemeRoller, you can include it in your project by modifying the HTML template:
html <!DOCTYPE html> <html> <head> <title>My jQuery UI Website</title> <link rel="stylesheet" href="https://code.jquery.com/ui/1.12.1/themes/custom-theme.css"> </head> <body> <!-- Your website content here --> <!-- jQuery and jQuery UI script includes --> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> <script src="https://code.jquery.com/ui/1.12.1/jquery-ui.js"></script> </body> </html>
Replace custom-theme.css with the path to your downloaded theme CSS file. Now, all the jQuery UI widgets will use your custom theme’s styles.
5. Interactive Effects
Besides providing user-friendly widgets, jQuery UI offers various interactive effects to enhance the user experience. These effects can be easily applied to any element on your website.
5.1 Drag-and-Drop
Drag-and-drop functionality adds an interactive element to your web application, making it easier for users to rearrange elements or upload files.
html <div id="draggableElement">Drag me!</div> <script> $(function() { $("#draggableElement").draggable(); }); </script>
With this code, the draggableElement will be draggable within its container, allowing users to move it around.
5.2 Resizable Elements
Allowing users to resize elements can be handy, especially when dealing with images or layout customization.
html <div id="resizableElement"></div> <script> $(function() { $("#resizableElement").resizable(); }); </script>
In this example, the resizableElement div will become resizable, enabling users to adjust its dimensions.
Conclusion
jQuery UI is a powerful library that enhances user experience through its user-friendly widgets, customizable theming options, and interactive effects. By incorporating these features into your web development projects, you can create intuitive and visually appealing user interfaces that keep visitors engaged and satisfied.
Remember to explore the extensive documentation provided by jQuery UI to unlock its full potential. Embrace the flexibility of this library, and let it guide you in crafting exceptional user experiences that leave a lasting impression on your audience.
So, what are you waiting for? Start using jQuery UI and elevate your web development game to create remarkable user experiences!
Table of Contents
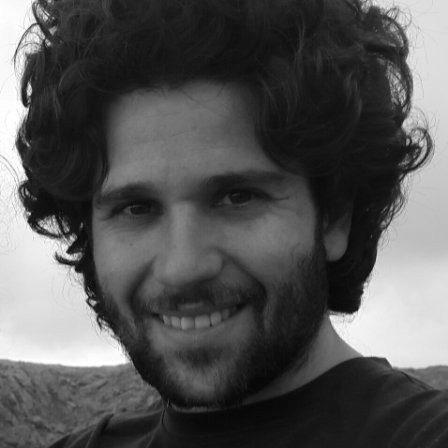
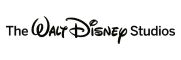