jQuery Validation: Ensuring Data Integrity in Forms
Web forms are an integral part of modern websites, allowing users to interact with online services, provide feedback, and input data. However, without proper validation mechanisms in place, the data submitted through these forms can be inaccurate, incomplete, or even malicious. This is where jQuery Validation comes into play. In this comprehensive guide, we’ll explore how jQuery Validation can be used to ensure data integrity in forms, enhance user experience, and streamline the data collection process.
Table of Contents
1. Understanding the Importance of Form Validation
1.1. Preventing Data-Related Issues
When users interact with web forms, the data they provide is essential for various purposes, such as user registration, contact forms, and online purchases. However, inaccurate or incomplete data can lead to serious issues, including failed transactions, lost leads, and frustrated users. Proper form validation ensures that the data submitted is valid, consistent, and meets specific criteria, reducing the likelihood of data-related problems.
1.2. Enhancing User Experience
Form validation not only prevents errors but also contributes to a better user experience. When users receive immediate feedback about their input, they can quickly correct mistakes, reducing frustration and enhancing engagement. By guiding users through the correct input format and validating their data in real-time, you create a smoother and more enjoyable experience on your website.
2. Introduction to jQuery Validation
2.1. What is jQuery Validation?
jQuery Validation is a popular JavaScript library that simplifies the process of validating user input in web forms. It offers a set of pre-defined validation rules and methods that can be easily integrated into your HTML forms. With jQuery Validation, you can perform validation checks on the client side before the form data is even submitted to the server, ensuring that only valid data is sent.
2.2. Advantages of Client-Side Validation
Client-side validation, as offered by jQuery Validation, offers several advantages over server-side validation alone. Firstly, it provides instantaneous feedback to users, allowing them to correct errors on the spot. This saves time and reduces the number of round-trips between the user and the server. Additionally, it offloads some of the validation work from the server, potentially improving server performance and reducing unnecessary traffic.
3. Getting Started with jQuery Validation
3.1. Including jQuery and jQuery Validation Libraries
To begin using jQuery Validation, you need to include the jQuery library and the jQuery Validation plugin in your HTML file. You can do this by adding the following lines of code to the <head> section of your HTML document:
html <!-- Include jQuery library --> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> <!-- Include jQuery Validation plugin --> <script src="https://cdn.jsdelivr.net/jquery.validation/1.16.0/jquery.validate.min.js"></script>
Make sure to adjust the version numbers in the URLs as needed.
3.2. Basic Setup and Implementation
Once you’ve included the required libraries, you can start using jQuery Validation in your forms. Let’s say you have a simple registration form with fields for the user’s name, email address, and password. Here’s how you can set up validation for this form:
html <form id="registrationForm"> <label for="name">Name:</label> <input type="text" id="name" name="name" required> <label for="email">Email:</label> <input type="email" id="email" name="email" required> <label for="password">Password:</label> <input type="password" id="password" name="password" required> <button type="submit">Register</button> </form> <script> // Initialize validation for the registration form $("#registrationForm").validate(); </script>
In this example, we’ve included the jQuery Validation plugin and initialized it on the registrationForm using the .validate() method. The required attribute on each input field indicates that the field must be filled out before the form can be submitted.
4. Common Validation Techniques
4.1. Required Field Validation
The required rule, as seen in the previous example, ensures that a field is not empty before the form can be submitted. This is the most basic form of validation and can be applied to various input types, such as text, email, and password fields.
html <label for="username">Username:</label> <input type="text" id="username" name="username" required>
4.2 Email and Phone Number Validation
jQuery Validation provides built-in validation rules for specific input types, such as email and phone number fields. These rules ensure that the input follows the correct format.
html <label for="email">Email:</label> <input type="email" id="email" name="email" required> <label for="phone">Phone:</label> <input type="tel" id="phone" name="phone" required>
4.3. Password Strength Validation
You can also implement custom validation rules to check for password strength. For instance, you can require passwords to have a minimum length and include both uppercase and lowercase letters, as well as numbers and special characters.
html <label for="password">Password:</label> <input type="password" id="password" name="password" required> javascript Copy code $("#registrationForm").validate({ rules: { password: { required: true, minlength: 8, pattern: /^(?=.*[a-z])(?=.*[A-Z])(?=.*\d)(?=.*[@$!%*?&])[A-Za-z\d@$!%*?&]{8,}$/ // Example pattern } } });
5. Customizing Validation Rules and Messages
5.1. Adding Custom Rules
jQuery Validation allows you to define custom validation rules tailored to your specific requirements. For instance, you might want to validate a field to ensure that the user’s age is within a certain range.
javascript $("#registrationForm").validate({ rules: { age: { required: true, range: [18, 99] // Custom rule for age range } } });
5.2. Modifying Error Messages
You can also customize the error messages displayed to users when their input doesn’t meet validation criteria. This helps provide clear guidance on what went wrong.
javascript $("#registrationForm").validate({ messages: { age: { required: "Please provide your age", range: "Your age must be between 18 and 99" } } });
6. Handling Form Submission
6.1. Validating Form on Submit
By default, jQuery Validation validates the form when the user tries to submit it. If any validation errors are present, the form submission is prevented, and the errors are displayed to the user.
javascript $("#registrationForm").validate();
6.2. Displaying Errors
jQuery Validation automatically displays error messages next to the respective input fields when validation fails. You can further customize the placement and appearance of these error messages to match your design.
javascript $("#registrationForm").validate({ errorPlacement: function (error, element) { error.insertAfter(element); // Display error messages after input fields } });
7. Advanced Validation Scenarios
7.1. Conditional Validation
In some cases, you might need to perform validation based on certain conditions. For example, you may have optional fields that need validation only if the user chooses to provide input.
javascript $("#registrationForm").validate({ rules: { optionalField: { required: "#checkbox:checked" // Validate only if checkbox is checked } } });
7.2. Cross-Field Validation
Cross-field validation involves validating one field based on the value of another field. For instance, you might want to ensure that two password fields match.
javascript $("#registrationForm").validate({ rules: { confirmPassword: { required: true, equalTo: "#password" // Validate against the password field } } });
8. Integrating jQuery Validation with AJAX
8.1. Dynamic Form Validation
In modern web applications, forms often involve dynamic interactions. jQuery Validation can seamlessly integrate with AJAX requests to validate data in real-time as users interact with the form.
javascript $("#registrationForm").validate({ rules: { email: { required: true, email: true, remote: { url: "check-email-availability.php", type: "post" } } } });
8.2. Real-Time Feedback
By using AJAX-based validation, you can provide users with immediate feedback on the validity of their input without needing to submit the form. This creates a smoother and more interactive experience.
9. Performance Considerations
9.1. Minifying JavaScript
To improve the loading time of your web pages, it’s advisable to minify your JavaScript code, including the jQuery Validation library. Minification reduces the file size by removing unnecessary characters, making your pages load faster.
9.2. Asynchronous Loading
You can also consider asynchronously loading JavaScript libraries to prevent them from blocking the rendering of your page. This can further enhance the overall performance and user experience.
10. Best Practices for Form Design
10.1. Providing Clear Instructions
Effective form design includes clear instructions on how to fill out the form. Use labels, placeholders, and hints to guide users through the process.
10.2. Using HTML5 Input Types
Leverage HTML5 input types to provide a better experience for users on different devices. For example, use the email input type for email fields to display appropriate keyboards on mobile devices.
10.3. Graceful Degradation
While client-side validation improves user experience, always ensure that your server-side validation is robust. Client-side validation can be bypassed, so server-side validation is a critical security measure.
11. Future of Form Validation
11.1. HTML Living Standard
The HTML specification continues to evolve, and modern browsers are incorporating more built-in form validation features. However, client-side libraries like jQuery Validation still play a crucial role in enhancing and customizing validation processes.
11.2. Modern JavaScript Frameworks
As web development trends shift towards more sophisticated JavaScript frameworks, you might find that form validation is integrated into these frameworks. Keep an eye on evolving technologies to adapt your validation strategies accordingly.
Conclusion
jQuery Validation is a powerful tool for ensuring data integrity in web forms. By implementing client-side validation, you can prevent data-related issues, enhance user experience, and streamline the data collection process. From basic validation techniques to advanced scenarios and integration with AJAX, this guide has covered essential concepts to help you create robust and user-friendly forms. As web development continues to evolve, staying informed about the latest techniques and best practices will ensure that your forms remain effective and efficient in ensuring data integrity.
Table of Contents
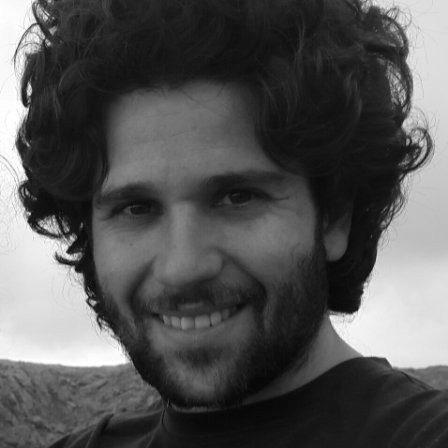
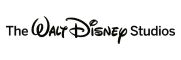