A Practical Guide to jQuery Event Delegation
In the world of web development, interactivity is a key factor in creating engaging and dynamic user experiences. Events, such as clicks, hovers, and keyboard interactions, play a crucial role in this interactivity. jQuery, a popular JavaScript library, has long been favored by developers for its simplicity and powerful features. One such feature, event delegation, offers an elegant solution for managing events efficiently, especially on dynamically generated content.
Table of Contents
1. Understanding Event Delegation
When developing web applications, it’s common to have dynamically generated elements that need to respond to user interactions. Traditionally, developers would attach event handlers directly to these elements. However, this approach becomes inefficient when dealing with a large number of elements, as it requires attaching a separate event handler to each element. This not only increases memory consumption but also affects performance.
Event delegation provides a more efficient alternative. Instead of attaching an event handler to each individual element, you attach a single event handler to a higher-level, static parent element. This parent element then listens for events triggered by its child elements. This technique is particularly useful when you have elements that are added or removed dynamically, as the event handling remains consistent regardless of changes in the DOM.
2. The Advantages of Event Delegation
Event delegation offers several advantages that make it a valuable technique in web development:
- Efficiency: By attaching a single event handler to a parent element, you reduce memory usage and improve performance, especially when dealing with a large number of elements.
- Dynamic Content: When new elements are added to the DOM dynamically, you don’t need to manually attach event handlers to them. The parent element takes care of capturing events from all its children, present and future.
- Simplicity: Event delegation simplifies your codebase by centralizing event handling logic. This leads to cleaner and more maintainable code.
3. Implementing Event Delegation
Let’s dive into the implementation details of event delegation using jQuery. We’ll go through the process step by step and provide code samples to illustrate each concept.
Step 1: Selecting the Parent Element
The first step in event delegation is to select the parent element to which you want to attach the event handler. This parent element should be a static ancestor of the dynamically generated child elements. You can use standard jQuery selectors to target this element. For instance, if you have a list of items with class “item-list,” you can select it as follows:
javascript const parentElement = $(".item-list");
Step 2: Defining the Event and Target
Next, you need to specify the event you want to capture and the target child element(s) that should trigger the event handler. For example, if you want to capture clicks on individual list items with class “item,” you would define the event and target as follows:
javascript parentElement.on("click", ".item", function(event) { // Event handling logic here });
In this code, the event handler is attached to the parent element and instructed to listen for click events that originate from elements with the class “item.”
Step 3: Writing the Event Handling Logic
Inside the event handler function, you can write the specific logic you want to execute when the event is triggered. The event parameter provides information about the event, such as the target element, the event type, and any associated data. For example, you can change the background color of the clicked item:
javascript parentElement.on("click", ".item", function(event) { $(event.target).css("background-color", "lightblue"); });
Step 4: Enjoying the Benefits
With your event delegation set up, you can now enjoy the benefits of efficient event handling on dynamically generated content. Whether you add new items to the list or remove existing ones, the event delegation mechanism remains unchanged.
4. Handling Different Types of Events
Event delegation is not limited to handling click events; it can be used for various types of interactions. Here are a few examples:
4.1. Mouseover Event
javascript parentElement.on("mouseover", ".item", function(event) { $(event.target).addClass("highlight"); });
In this example, when the mouse pointer hovers over an item, it gains a “highlight” class.
4.2. Form Submission
javascript const formElement = $(".my-form"); formElement.on("submit", function(event) { event.preventDefault(); // Form submission handling here });
Here, the event delegation approach prevents the need to attach the submit event handler directly to each form.
5. Caveats and Considerations
While event delegation offers many advantages, it’s essential to keep a few things in mind:
- Performance Implications: Although event delegation is efficient for large numbers of elements, excessive use of event delegation across multiple levels of the DOM can still impact performance.
- Propagation and Bubbling: Events triggered on child elements can propagate up the DOM tree. Use methods like stopPropagation() to control event bubbling if needed.
- Dynamic Changes: If the parent element itself is dynamically added or removed, you’ll need to reattach the event handler.
Conclusion
jQuery event delegation is a powerful technique that simplifies event handling for dynamically generated content. By attaching a single event handler to a static parent element, you can efficiently manage interactions on numerous child elements. This approach improves performance, maintains consistency, and leads to cleaner code. Remember the steps: select the parent element, define the event and target, write your event handling logic, and enjoy the benefits of streamlined interaction in your web applications. While jQuery itself is becoming less prevalent in modern web development, the concept of event delegation remains relevant and can be applied using native JavaScript methods as well. So, whether you’re a jQuery enthusiast or an advocate of plain JavaScript, event delegation is a tool worth mastering for creating exceptional user experiences.
Table of Contents
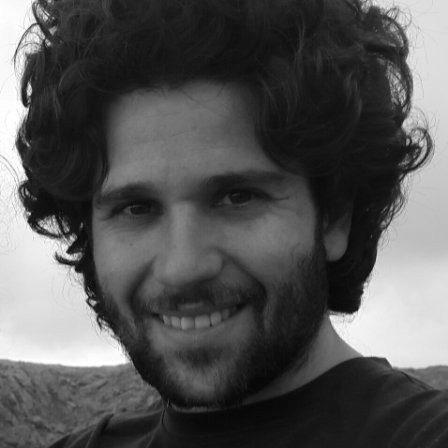
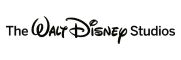