Building Expandable Content Sections with jQuery Expand
In the ever-evolving landscape of web development, creating interactive and user-friendly interfaces is paramount. Expandable content sections, also known as collapsible sections, are a great way to improve user experience on your website. They allow you to present information in a neat and organized manner, making it easy for users to access content while keeping the interface clutter-free. In this blog post, we’ll explore how to build expandable content sections using jQuery Expand, a powerful JavaScript library that simplifies the process.
Table of Contents
1. Why Expandable Content Sections Matter
Before we dive into the implementation details, let’s briefly discuss why expandable content sections are essential for modern web design.
1.1. Enhanced User Experience
Expandable content sections make it easy for users to navigate through your website’s content. By hiding non-essential information initially, you reduce clutter and distractions, allowing users to focus on what’s important. This leads to a smoother and more enjoyable user experience.
1.2. Improved Readability
Long web pages with endless text can overwhelm visitors. Collapsible sections allow you to break content into manageable chunks, making it easier to scan and read. Users can expand only the sections they’re interested in, improving overall readability.
1.3. Space Efficiency
Expanding sections on demand means you can fit more content into a limited space without overwhelming the user. This is especially valuable for mobile and responsive web design, where screen real estate is at a premium.
2. Getting Started with jQuery Expand
Now that we understand the importance of expandable content sections, let’s see how jQuery Expand can help us implement this functionality easily. jQuery Expand is a lightweight and versatile JavaScript library that simplifies creating collapsible sections. Here’s how to get started:
2.1. Include jQuery and jQuery Expand
First, make sure you include jQuery and jQuery Expand in your HTML file. You can either download them and host them locally or include them from a Content Delivery Network (CDN).
html <!-- Include jQuery --> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> <!-- Include jQuery Expand --> <script src="https://cdn.jsdelivr.net/npm/jquery-expand@1.0.0/dist/jquery-expand.min.js"></script>
2.2. HTML Structure
Create the HTML structure for your expandable content section. You’ll typically have a heading or a button to trigger the expansion and a div to hold the content that expands or collapses.
html <div class="expandable-section"> <button class="expand-button">Expand Section</button> <div class="expand-content"> <!-- Content goes here --> </div> </div>
2.3. Initialize jQuery Expand
Next, you’ll need to initialize jQuery Expand. Use the following code to make it work:
javascript $(document).ready(function() { $('.expandable-section').expand(); });
This code waits for the document to be ready and then selects all elements with the class expandable-section to apply the expand() method, which enables the expandable functionality.
2.4. Styling
To make your expandable sections visually appealing, you can apply CSS styles to control the appearance of the sections and the expand/collapse animations.
css .expandable-section { margin: 10px; border: 1px solid #ccc; border-radius: 5px; padding: 10px; } .expand-button { background-color: #007BFF; color: #fff; border: none; padding: 5px 10px; cursor: pointer; } .expand-content { display: none; padding: 10px; } .expanded { display: block; }
In this example, we’ve defined a basic style for the expandable section, the button, and the content. We’ve set the initial display of .expand-content to none, which hides it. The .expanded class is used to display the content when the section is expanded.
2.5. Adding Functionality
Now, you’ll want to add functionality to your expandable sections. To toggle the visibility of the content when the button is clicked, use the following JavaScript code:
javascript $(document).ready(function() { $('.expandable-section').expand(); $('.expand-button').click(function() { $(this).siblings('.expand-content').toggleClass('expanded'); }); });
In this code, when a button with the class .expand-button is clicked, it finds its sibling with the class .expand-content and toggles the .expanded class, which controls the visibility of the content.
3. Customizing Expandable Sections
While we’ve covered the basics of creating expandable content sections with jQuery Expand, you can customize this functionality to suit your specific needs. Here are a few customization options:
3.1. Multiple Expandable Sections
You can have multiple expandable sections on a single page by repeating the HTML structure and initialization code for each section with unique classes or identifiers.
html <div class="expandable-section"> <button class="expand-button">Expand Section 1</button> <div class="expand-content"> <!-- Content for Section 1 --> </div> </div> <div class="expandable-section"> <button class="expand-button">Expand Section 2</button> <div class="expand-content"> <!-- Content for Section 2 --> </div> </div>
3.2. Animation Effects
jQuery Expand provides options to customize the animation effects when expanding and collapsing sections. You can specify the duration and easing function for smooth transitions.
javascript $('.expandable-section').expand({ duration: 500, // Duration in milliseconds easing: 'swing' // Easing function (e.g., 'linear', 'swing', 'easeOutBounce') });
3.3. Callback Functions
You can execute custom functions before and after expanding or collapsing sections using callback functions. For example, you might want to update other elements on the page when a section is expanded or collapsed.
javascript $('.expandable-section').expand({ beforeExpand: function() { // Code to execute before expanding }, afterExpand: function() { // Code to execute after expanding }, beforeCollapse: function() { // Code to execute before collapsing }, afterCollapse: function() { // Code to execute after collapsing } });
Conclusion
Incorporating expandable content sections into your website can greatly enhance the user experience by providing organized and easily accessible information. With jQuery Expand, you can implement this functionality effortlessly and customize it to fit your design requirements.
Remember to keep user experience at the forefront of your web development efforts. Providing a clean and intuitive interface, such as expandable sections, can make a significant difference in how users interact with your website. So, go ahead, experiment with jQuery Expand, and create expandable content sections that elevate your web design to the next level. Your users will thank you for it.
Table of Contents
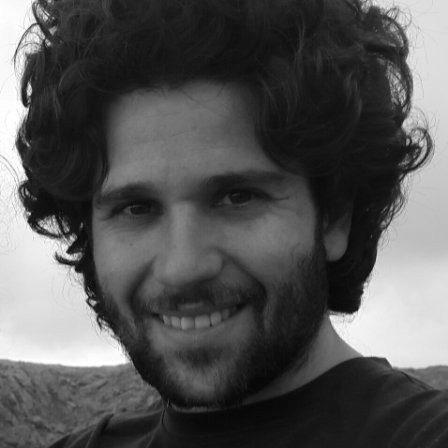
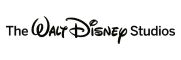