Building Filterable Image Galleries with jQuery Isotope
In the world of web design, aesthetics and functionality go hand in hand. One of the most visually appealing elements you can add to your website is a filterable image gallery. This dynamic feature allows users to sort and view images in various categories or with different attributes, enhancing user engagement and experience. In this comprehensive guide, we’ll explore how to create filterable image galleries using jQuery Isotope, a powerful and flexible JavaScript library. By the end of this tutorial, you’ll be equipped with the knowledge and code samples needed to elevate your web design game.
Table of Contents
1. Why Choose jQuery Isotope?
Before we dive into the nitty-gritty of building filterable image galleries, let’s understand why jQuery Isotope is an excellent choice for this task.
1.1. Lightweight and Efficient
jQuery Isotope is a compact library that doesn’t bloat your website with unnecessary code. It’s designed to be fast and efficient, ensuring your gallery loads quickly, even with a large number of images.
1.2. Seamless Filtering and Sorting
Isotope provides a seamless way to filter and sort your images. Users can easily switch between categories or sort images based on different criteria, enhancing the overall user experience.
1.3. Cross-Browser Compatibility
jQuery Isotope is compatible with all major browsers, ensuring your filterable image gallery works flawlessly for all your website visitors, regardless of their browser preference.
1.4. Responsive Design
In today’s mobile-first world, responsive design is crucial. jQuery Isotope makes it easy to create responsive filterable image galleries that adapt to different screen sizes, from desktop to mobile devices.
Now that we’ve covered the advantages of using jQuery Isotope let’s get down to building our filterable image gallery step by step.
2. Prerequisites
Before we begin, ensure you have the following prerequisites in place:
- Basic HTML/CSS Knowledge: You should have a good understanding of HTML and CSS to structure and style your gallery.
- jQuery and jQuery Isotope: Make sure you’ve included the jQuery and jQuery Isotope libraries in your project. You can download them from the official websites or include them via Content Delivery Networks (CDNs).
- Images: Prepare the images you want to display in your gallery and organize them into categories or groups as needed.
3. Setting Up the HTML Structure
Let’s start by setting up the basic HTML structure for our filterable image gallery. We’ll use a simple unordered list (<ul>) to hold the images. Each list item (<li>) will represent an image, and we’ll use data attributes to categorize them.
html <!DOCTYPE html> <html lang="en"> <head> <!-- Include jQuery and jQuery Isotope here --> <!-- Add your stylesheets and other scripts as needed --> </head> <body> <div class="gallery"> <ul> <li data-category="nature"> <img src="images/nature1.jpg" alt="Nature 1"> </li> <li data-category="city"> <img src="images/city1.jpg" alt="City 1"> </li> <!-- Add more images and categories here --> </ul> </div> </body> </html>
In the code above, we’ve created a simple gallery container with an unordered list. Each list item has a data-category attribute that defines the category to which the image belongs. You can add more images and categories as needed.
4. Styling Your Gallery
Now that we have our HTML structure in place, let’s add some basic CSS to style our gallery. We’ll create a grid layout for the images and apply some styling to make it visually appealing.
css /* Add your CSS styles to style.css */ .gallery { display: grid; grid-template-columns: repeat(auto-fill, minmax(250px, 1fr)); gap: 20px; list-style: none; padding: 0; } .gallery li { position: relative; overflow: hidden; border-radius: 10px; box-shadow: 0px 0px 10px rgba(0, 0, 0, 0.2); } .gallery img { max-width: 100%; height: auto; }
This CSS code sets up a responsive grid layout for our gallery and adds some basic styling to the list items and images. Customize it further to match your website’s design.
5. Initializing Isotope
With our HTML structure and initial styling in place, it’s time to initialize jQuery Isotope to enable filtering and sorting. Include the following JavaScript code at the end of your HTML, just before the closing </body> tag.
html <script> $(document).ready(function () { // Initialize Isotope $('.gallery ul').isotope({ itemSelector: '.gallery li', layoutMode: 'fitRows' }); }); </script>
In this code, we’re using the $(document).ready() function to ensure that the DOM is fully loaded before we initialize Isotope. We’re targeting the <ul> element within our .gallery container and specifying that each <li> is an item. The layoutMode is set to fitRows, which arranges the items in a horizontal row.
6. Adding Filter Buttons
To allow users to filter the images based on categories, we’ll create filter buttons. These buttons will trigger the filtering process when clicked. Add the following HTML code for the filter buttons:
html <div class="filter-buttons"> <button data-filter="*">All</button> <button data-filter=".nature">Nature</button> <button data-filter=".city">City</button> <!-- Add more buttons for other categories --> </div>
Now, let’s add JavaScript to handle the button clicks and initiate the filtering process.
html <script> $(document).ready(function () { // Initialize Isotope var $gallery = $('.gallery ul'); $gallery.isotope({ itemSelector: '.gallery li', layoutMode: 'fitRows' }); // Filter items when the button is clicked $('.filter-buttons button').on('click', function () { var filterValue = $(this).attr('data-filter'); $gallery.isotope({ filter: filterValue }); }); }); </script>
In this JavaScript code, we’re first storing a reference to our gallery’s <ul> element in the $gallery variable. Then, we’re using the on(‘click’) event handler to detect when a filter button is clicked. When a button is clicked, we retrieve its data-filter attribute, which contains the filter value (e.g., .nature, .city). We then use Isotope to filter the gallery based on this value.
7. Adding Animation (Optional)
To make the filtering process smoother and more visually appealing, you can add animations. Isotope provides built-in animation options. Update your Isotope initialization code as follows:
html <script> $(document).ready(function () { // Initialize Isotope var $gallery = $('.gallery ul'); $gallery.isotope({ itemSelector: '.gallery li', layoutMode: 'fitRows', transitionDuration: '0.6s' // Adjust the duration as needed }); // Filter items when the button is clicked $('.filter-buttons button').on('click', function () { var filterValue = $(this).attr('data-filter'); $gallery.isotope({ filter: filterValue }); }); }); </script>
With the transitionDuration property, you can control the animation speed when items are filtered.
Conclusion
Congratulations! You’ve successfully created a filterable image gallery using jQuery Isotope. This dynamic feature not only enhances the visual appeal of your website but also improves user engagement by allowing visitors to explore your content in a more interactive way. You can further customize your gallery by adding more categories, fine-tuning the styles, or integrating it into a larger web project.
Remember that web design is all about creativity, so feel free to experiment and tailor your filterable image gallery to suit your specific needs and preferences. With jQuery Isotope, the possibilities are endless, and your website can truly stand out in the digital landscape.
Now it’s your turn to implement and customize a filterable image gallery using jQuery Isotope. Happy coding!
In this comprehensive guide, we’ve explored how to create stunning filterable image galleries using jQuery Isotope. From setting up the HTML structure to adding filter buttons and animations, you now have the knowledge and code samples to elevate your web design game. Whether you’re a beginner or an experienced developer, this tutorial empowers you to enhance user engagement and create visually appealing websites. So, roll up your sleeves and start building filterable image galleries that captivate your audience.
Table of Contents
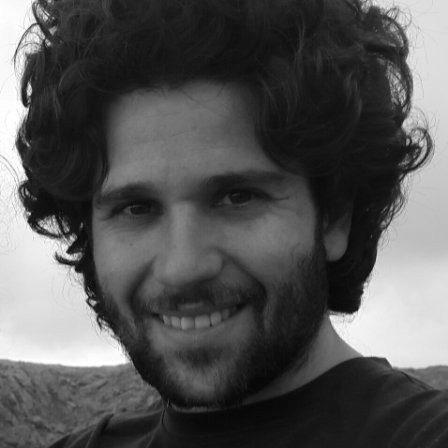
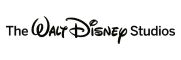