jQuery and Firebase: Building Real-Time Applications
In today’s fast-paced digital world, real-time applications have become the norm. From chat applications to collaborative tools, users expect information to update instantly. This demand for real-time functionality has driven the need for efficient, scalable solutions. jQuery and Firebase, when combined, offer a potent toolkit for building such applications.
Table of Contents
1. Introduction to Real-Time Applications
1.1. Understanding Real-Time Applications
Real-time applications are those that provide instant updates to users as data changes. Examples include live chat applications, collaborative document editors, and online gaming platforms. These applications require constant data synchronization between clients and servers to deliver a seamless user experience.
1.2. The Power of jQuery
jQuery, a fast and feature-rich JavaScript library, simplifies HTML document traversal and manipulation, event handling, and animation. It is renowned for its ease of use and cross-browser compatibility. By leveraging jQuery, developers can build responsive and interactive web applications with less effort.
1.3. Firebase: A Real-Time Database
Firebase, a platform offered by Google, provides a real-time database and a suite of tools for building web and mobile applications. Firebase’s real-time database allows data to be synchronized in milliseconds across all connected clients. It also offers user authentication, cloud functions, and cloud storage, making it a comprehensive solution for building real-time applications.
2. Setting Up Your Development Environment
Before we dive into building real-time applications with jQuery and Firebase, let’s set up our development environment.
2.1. Installing jQuery
You can include jQuery in your project by adding the following script tag to your HTML file:
html <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
Alternatively, you can download the jQuery library and host it on your server.
2.2. Creating a Firebase Project
To get started with Firebase, visit the Firebase Console (https://console.firebase.google.com/) and create a new project. Follow the setup wizard to configure Firebase for your application.
3. Basic jQuery and Firebase Integration
Now that our development environment is set up let’s start integrating jQuery and Firebase into our application.
3.1. Including jQuery in Your Project
In your HTML file, include the jQuery library by adding the script tag mentioned earlier. Make sure it is included before any other JavaScript code that depends on jQuery.
3.2. Initializing Firebase
To use Firebase in your project, you need to initialize it with your Firebase project’s configuration. You can find this configuration in your Firebase project settings under the “General” tab. Add the following code to your JavaScript file:
javascript // Initialize Firebase var config = { apiKey: "YOUR_API_KEY", authDomain: "YOUR_AUTH_DOMAIN", databaseURL: "YOUR_DATABASE_URL", projectId: "YOUR_PROJECT_ID", storageBucket: "YOUR_STORAGE_BUCKET", messagingSenderId: "YOUR_MESSAGING_SENDER_ID" }; firebase.initializeApp(config);
Replace the placeholders with the actual values from your Firebase project.
4. Real-Time Data Synchronization
One of the core features of Firebase is its real-time data synchronization. Let’s explore how to add data to Firebase and display real-time updates using jQuery.
4.1. Adding Data to Firebase
You can store data in Firebase’s real-time database as JSON objects. Here’s an example of adding a simple message to the database:
javascript var database = firebase.database(); var messagesRef = database.ref('messages'); var messageData = { username: 'john_doe', text: 'Hello, Firebase!' }; messagesRef.push(messageData);
This code creates a reference to a “messages” node in the Firebase database and pushes a new message object to it.
4.2. Displaying Real-Time Updates with jQuery
Now, let’s display real-time updates of these messages in our web application. Assuming you have an HTML element with the id “messageList” to display messages, you can use the following code:
javascript var messageList = $('#messageList'); messagesRef.on('child_added', function(snapshot) { var message = snapshot.val(); var messageItem = $('<li>').text(message.username + ': ' + message.text); messageList.append(messageItem); });
This code listens for new child elements (messages) added to the “messages” node in the database and appends them to the “messageList” element in real-time.
5. Authentication and Security
Building secure real-time applications is crucial. Firebase offers robust authentication and security features to help protect your data and control access.
5.1. User Authentication with Firebase
Firebase provides various authentication methods, including email and password authentication, Google sign-in, and more. Here’s how to set up email and password authentication:
- Go to the Firebase Console.
- Navigate to the “Authentication” section.
- Enable the “Email/Password” sign-in method.
- Use Firebase SDK to implement authentication in your app.
javascript // Sign up a new user firebase.auth().createUserWithEmailAndPassword(email, password) .then(function(user) { // User signed up successfully }) .catch(function(error) { // Handle errors }); // Sign in an existing user firebase.auth().signInWithEmailAndPassword(email, password) .then(function(user) { // User signed in successfully }) .catch(function(error) { // Handle errors });
5.2. Securing Your Firebase Database
Firebase allows you to define security rules for your database to control who can read and write data. You can set these rules in the Firebase Console under the “Database” section. For example, you can restrict write access to authenticated users only:
json { "rules": { ".read": "auth != null", ".write": "auth != null" } }
By implementing user authentication and security rules, you can ensure that your real-time application’s data remains safe and accessible only to authorized users.
6. Advanced Features
Now that you’ve mastered the basics, let’s explore some advanced features you can implement in your real-time application.
6.1. Real-Time Collaboration
Firebase’s real-time capabilities make it an excellent choice for collaborative applications. You can create features like collaborative document editing or real-time drawing boards by leveraging Firebase’s real-time database to synchronize data between users in real-time.
6.2. File Storage with Firebase Storage
In addition to the real-time database, Firebase offers Firebase Storage for securely storing and serving user-generated content, such as images, videos, and files. You can easily integrate Firebase Storage into your application to handle file uploads and downloads.
7. Deployment and Best Practices
As your real-time application takes shape, it’s essential to consider deployment and best practices.
7.1. Deploying Your Real-Time Application
When deploying your application, make sure to configure Firebase hosting, which provides fast and secure hosting for your web app. You can deploy your app using the Firebase CLI with a simple command:
bash firebase deploy
Firebase Hosting also provides automatic SSL certificate provisioning and CDN integration for improved performance.
7.2. Best Practices for jQuery and Firebase
Here are some best practices to keep in mind while building real-time applications with jQuery and Firebase:
- Optimize your data structure in the Firebase database to minimize read and write operations.
- Implement client-side data validation to ensure data consistency.
- Handle connection loss gracefully and provide offline support using Firebase’s offline capabilities.
- Regularly monitor and analyze Firebase usage to optimize costs and performance.
Conclusion
Building real-time applications with jQuery and Firebase offers a powerful combination of ease of use, real-time data synchronization, and robust security. Whether you’re creating a chat application, a collaborative tool, or any other real-time application, jQuery and Firebase provide the tools you need to deliver a seamless and responsive user experience. With the knowledge gained from this guide, you’re well-equipped to embark on your real-time development journey. Happy coding!
Table of Contents
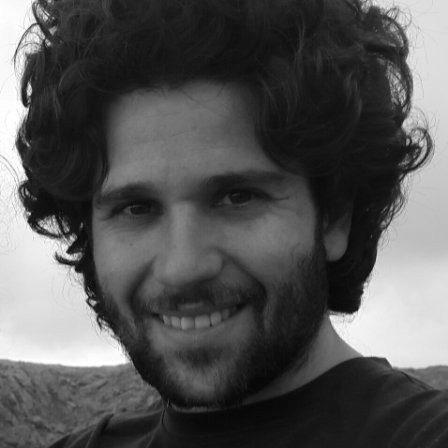
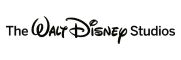