Building Dynamic Forms with jQuery Form Plugin
Forms are an essential part of web development, enabling user interaction and data submission. As web applications grow more complex, the need for dynamic and interactive forms becomes increasingly important. jQuery Form Plugin is a powerful tool that simplifies the process of building dynamic forms with enhanced features such as AJAX form submissions, form validation, and more. In this blog, we’ll explore the jQuery Form Plugin and demonstrate how to create dynamic forms with various examples and code snippets.
Table of Contents
1. What is jQuery Form Plugin?
jQuery Form Plugin is a popular JavaScript library that extends the capabilities of standard HTML forms. It provides a convenient way to handle various form-related functionalities, including AJAX form submissions, form validation, file uploads, and form element enhancements. By utilizing the jQuery Form Plugin, developers can streamline the process of building dynamic forms without the need for extensive JavaScript code.
2. Getting Started
To begin using the jQuery Form Plugin, you need to include jQuery and the plugin script in your HTML file. You can either download the plugin files or use a Content Delivery Network (CDN) to access the latest version. Below is an example of how to include the required scripts in your project:
html <!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>Dynamic Forms with jQuery Form Plugin</title> <!-- Include jQuery library --> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> <!-- Include jQuery Form Plugin --> <script src="https://cdn.jsdelivr.net/npm/jquery.form@4.3.0"></script> </head> <body> <!-- Your form goes here --> </body> </html>
3. Creating a Basic Form
Let’s start by creating a basic HTML form that we’ll later enhance with the jQuery Form Plugin functionalities. For simplicity, we’ll build a contact form with a name, email, and message fields. Below is the HTML code for our initial form:
html <form id="contactForm" action="submit.php" method="post"> <label for="name">Name:</label> <input type="text" id="name" name="name" required> <label for="email">Email:</label> <input type="email" id="email" name="email" required> <label for="message">Message:</label> <textarea id="message" name="message" required></textarea> <input type="submit" value="Submit"> </form>
4. Initializing the jQuery Form Plugin
To enable the jQuery Form Plugin for our form, we need to initialize it with some options. The most common option is the url, which specifies the server-side script to handle the form submission. In this example, we’ll use a simple PHP script named “submit.php.” This script will receive the form data and process it on the server.
html <script> $(document).ready(function() { $('#contactForm').ajaxForm({ url: 'submit.php', type: 'post', success: function(response) { // Handle the server response here }, error: function(error) { // Handle errors here } }); }); </script>
5. AJAX Form Submission
With the jQuery Form Plugin configured, our form will now be submitted via AJAX. When the user clicks the “Submit” button, the form data will be sent to the server in the background without requiring a page refresh. This provides a more seamless and responsive user experience.
6. Handling Form Submissions on the Server (submit.php)
On the server side, we need a script (in this case, “submit.php”) to process the form data and send a response back to the client. For demonstration purposes, we’ll keep the server-side script simple and assume the data is successfully processed:
php <?php // Get form data $name = $_POST['name']; $email = $_POST['email']; $message = $_POST['message']; // Process the data (you can add your logic here) // Send a response back to the client $response = array('message' => 'Form submitted successfully!'); echo json_encode($response); ?>
7. Form Validation
Form validation is a crucial aspect of user input. It ensures that the data submitted is correct and meets specific criteria. The jQuery Form Plugin supports form validation using the beforeSubmit option. We can add a function to this option that performs validation before the form is submitted.
In this example, we’ll implement basic client-side validation for the name and email fields:
html <script> $(document).ready(function() { $('#contactForm').ajaxForm({ url: 'submit.php', type: 'post', beforeSubmit: validateForm, success: function(response) { // Handle the server response here }, error: function(error) { // Handle errors here } }); function validateForm(formData, jqForm, options) { var name = $('#name').val(); var email = $('#email').val(); var message = $('#message').val(); var valid = true; if (name === '') { alert('Please enter your name.'); valid = false; } if (email === '' || !isValidEmail(email)) { alert('Please enter a valid email address.'); valid = false; } return valid; } function isValidEmail(email) { // Basic email validation regex var pattern = /^[a-zA-Z0-9._-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,4}$/; return pattern.test(email); } }); </script>
In this code, we first set up the beforeSubmit option with the validateForm function, which performs the validation checks for the name and email fields. If any validation fails, an alert will be shown, and the form submission will be halted.
8. File Uploads
jQuery Form Plugin also provides support for handling file uploads in forms. By setting the dataType option to “json” and adding the success callback function, we can handle file uploads gracefully and receive the server response in JSON format.
Let’s modify our form to include a file input field for image uploads:
html <form id="imageUploadForm" action="upload.php" method="post" enctype="multipart/form-data"> <input type="file" id="image" name="image" required> <input type="submit" value="Upload"> </form>
And update the jQuery initialization script:
html <script> $(document).ready(function() { $('#imageUploadForm').ajaxForm({ url: 'upload.php', type: 'post', dataType: 'json', success: function(response) { // Handle the server response here (e.g., display uploaded image) }, error: function(error) { // Handle errors here } }); }); </script>
On the server side (in our example, “upload.php”), we can process the uploaded file using PHP and send a response back to the client:
php <?php $targetDir = "uploads/"; $targetFile = $targetDir . basename($_FILES["image"]["name"]); $uploadOk = 1; $imageFileType = strtolower(pathinfo($targetFile, PATHINFO_EXTENSION)); // Check if image file is a valid image if (isset($_POST["submit"])) { $check = getimagesize($_FILES["image"]["tmp_name"]); if ($check !== false) { echo json_encode(array('message' => 'File is an image - ' . $check["mime"] . '.')); $uploadOk = 1; } else { echo json_encode(array('message' => 'File is not an image.')); $uploadOk = 0; } } // Check if file already exists if (file_exists($targetFile)) { echo json_encode(array('message' => 'Sorry, file already exists.')); $uploadOk = 0; } // Check file size (in this example, the limit is set to 1MB) if ($_FILES["image"]["size"] > 1000000) { echo json_encode(array('message' => 'Sorry, your file is too large.')); $uploadOk = 0; } // Allow certain file formats (in this example, only JPG, JPEG, PNG, and GIF are allowed) $allowedFormats = array('jpg', 'jpeg', 'png', 'gif'); if (!in_array($imageFileType, $allowedFormats)) { echo json_encode(array('message' => 'Sorry, only JPG, JPEG, PNG, and GIF files are allowed.')); $uploadOk = 0; } // Check if $uploadOk is set to 0 by an error if ($uploadOk == 0) { echo json_encode(array('message' => 'Sorry, your file was not uploaded.')); } else { // If everything is fine, try to upload the file if (move_uploaded_file($_FILES["image"]["tmp_name"], $targetFile)) { echo json_encode(array('message' => 'The file ' . basename($_FILES["image"]["name"]) . ' has been uploaded.')); } else { echo json_encode(array('message' => 'Sorry, there was an error uploading your file.')); } } ?>
9. Dynamic Form Elements
With jQuery Form Plugin, you can easily add or remove form elements dynamically. This can be useful when dealing with forms that require variable numbers of input fields based on user selections. For example, consider a form where users can add multiple email addresses:
html <form id="dynamicForm" action="process.php" method="post"> <div id="emailContainer"> <input type="email" name="email[]" required> <button type="button" id="addEmail">Add Email</button> </div> <input type="submit" value="Submit"> </form>
In the above form, we have a container div with an input field for an email address and a button labeled “Add Email.” The idea is that users can click the “Add Email” button to create additional email input fields.
Let’s implement this functionality using the jQuery Form Plugin:
html <script> $(document).ready(function() { var maxEmails = 5; // Maximum number of email fields allowed $('#dynamicForm').ajaxForm({ url: 'process.php', type: 'post', success: function(response) { // Handle the server response here }, error: function(error) { // Handle errors here } }); $('#addEmail').click(function() { var emailContainer = $('#emailContainer'); var numEmails = emailContainer.find('input[type="email"]').length; if (numEmails < maxEmails) { var newEmailField = $('<input type="email" name="email[]" required>'); emailContainer.append(newEmailField); } }); }); </script>
In this example, we set a maximum limit of five email input fields (you can adjust this as needed). When the “Add Email” button is clicked, we check if the current number of email fields is less than the maximum allowed. If it is, we create a new email input field and append it to the container.
Conclusion
In this blog post, we’ve explored the powerful jQuery Form Plugin and its various functionalities for building dynamic and interactive forms. We covered how to initialize the plugin, perform AJAX form submissions, implement form validation, handle file uploads, and dynamically add form elements. By using this plugin, web developers can enhance user experience, simplify form handling, and create more engaging web applications.
Building dynamic forms with the jQuery Form Plugin opens up a world of possibilities for web developers, enabling them to create sophisticated and seamless user interactions. As you continue to explore this powerful tool, don’t hesitate to experiment with different options and combinations to tailor it to your specific project needs.
Remember that form validation and server-side processing are crucial for handling user input securely and accurately. Always validate data on both the client and server sides to ensure data integrity and protect your application from potential vulnerabilities.
Now it’s time to get hands-on and start building your own dynamic forms with the jQuery Form Plugin. Happy coding!
Table of Contents
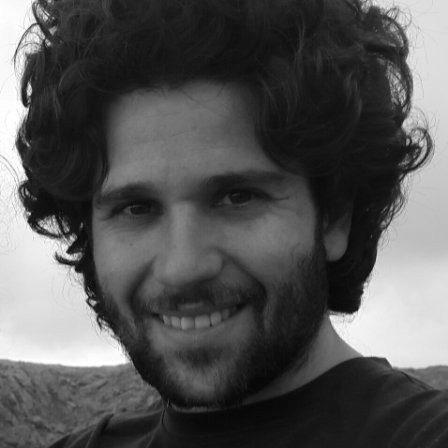
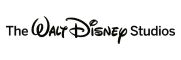