Simplifying Form Styling with jQuery Form Styler
Forms are an essential component of web applications and websites. They allow users to input information, make selections, and interact with digital content. However, creating visually appealing and user-friendly forms can be a challenging task. This is where jQuery Form Styler comes to the rescue.
Table of Contents
In this comprehensive guide, we will explore how to simplify form styling using jQuery Form Styler. Whether you are a seasoned developer or just starting with web development, you will find this tool incredibly useful for enhancing the aesthetics and user experience of your web forms.
1. What is jQuery Form Styler?
jQuery Form Styler is a JavaScript library that simplifies the styling of HTML form elements. It allows you to apply custom styles to checkboxes, radio buttons, select boxes, and file inputs. This means you can easily transform the default, often bland, form elements into visually appealing, user-friendly components.
2. Getting Started
Let’s get started by integrating jQuery Form Styler into your project. You can include it in your HTML file using a script tag. You also need to include jQuery because jQuery Form Styler depends on it.
html <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Form Styling with jQuery Form Styler</title> <link rel="stylesheet" href="jquery.formstyler.css"> </head> <body> <!-- Your form goes here --> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> <script src="jquery.formstyler.min.js"></script> <script> $(document).ready(function () { $('select').styler(); // Initialize the styler for select boxes // You can also initialize other form elements here }); </script> </body> </html>
Now that you have included the necessary scripts, you are ready to start styling your form elements.
3. Styling Select Boxes
Select boxes, also known as dropdowns, are commonly used in forms. By default, their appearance can vary from browser to browser and may not always align with your design. With jQuery Form Styler, you can easily customize their appearance.
Here’s how you can style a select box:
html <select> <option value="option1">Option 1</option> <option value="option2">Option 2</option> <option value="option3">Option 3</option> </select>
After initializing the styler as shown in the previous section, the select box will automatically be transformed into a more user-friendly component. You can further customize its appearance by modifying the provided CSS classes.
4. Customizing Checkboxes and Radio Buttons
Checkboxes and radio buttons are crucial for user interactions, but their default appearance can be dull. Let’s see how you can style them using jQuery Form Styler.
4.1. Styling Checkboxes
html <input type="checkbox" id="checkbox1"> <label for="checkbox1">Checkbox 1</label> <input type="checkbox" id="checkbox2"> <label for="checkbox2">Checkbox 2</label>
After initializing the styler, these checkboxes will have a modern and sleek appearance, making them more visually appealing.
4.2. Styling Radio Buttons
html <input type="radio" id="radio1" name="radioGroup"> <label for="radio1">Radio 1</label> <input type="radio" id="radio2" name="radioGroup"> <label for="radio2">Radio 2</label>
Just like checkboxes, radio buttons will also be restyled to fit your design seamlessly.
5. Enhancing File Inputs
File inputs, which allow users to upload files, are often overlooked when it comes to styling. jQuery Form Styler ensures that even file inputs can be made more presentable.
html <input type="file" id="fileInput" class="styler"> <label for="fileInput">Choose a file</label>
By adding the “styler” class and initializing the styler, you can make your file input element blend harmoniously with the rest of your styled form.
6. Customization Options
jQuery Form Styler provides various customization options to tailor the appearance of your form elements according to your project’s needs.
6.1. Custom Styles
You can apply custom styles to your form elements by modifying the provided CSS classes. For example, you can change the background color, text color, and border styles to match your website’s theme.
css /* Custom CSS for select boxes */ .selectbox { background-color: #f0f0f0; color: #333; border: 2px solid #ccc; }
6.2. Disabled Elements
Disabled form elements can be styled differently to indicate their inactive state.
css /* Styling for disabled select boxes */ .selectbox:disabled { background-color: #eee; color: #999; }
6.3. Custom Icons
You can also add custom icons to your checkboxes and radio buttons for a unique look.
css /* Custom checkbox icon */ .checkbox-icon::before { content: "\f00c"; /* Unicode for a checkmark icon */ font-family: FontAwesome; /* You can use any icon font */ color: green; }
6.4. Placeholder Text
Styling for placeholder text can be adjusted to make it more visible.
css /* Styling for select box placeholder text */ .selectbox::placeholder { color: #999; }
7. Handling Events
jQuery Form Styler doesn’t just enhance the visual aspects of your form elements; it also allows you to handle events easily.
For instance, if you want to perform some action when a user selects an option from a styled select box, you can use jQuery’s event handling functions.
javascript $('select').on('change', function () { var selectedOption = $(this).val(); // Perform your desired action here });
Similarly, you can attach event handlers to styled checkboxes and radio buttons to respond to user interactions.
8. Benefits of Using jQuery Form Styler
Now that you’ve seen how easy it is to style form elements with jQuery Form Styler, let’s explore the benefits of using this library.
8.1. Improved User Experience
Styled form elements not only look better but also enhance the overall user experience. Users can easily identify and interact with them, which can lead to higher user satisfaction and increased form submissions.
8.2. Consistent Design
jQuery Form Styler ensures that your form elements have a consistent design across different browsers and platforms. This helps maintain your website’s visual identity.
8.3. Accessibility
jQuery Form Styler is designed with accessibility in mind. It ensures that your styled form elements remain accessible to users with disabilities, making your website more inclusive.
8.4. Time-Saving
Styling form elements from scratch can be time-consuming. jQuery Form Styler saves you time by providing a quick and easy way to style form elements without the need for extensive CSS and JavaScript coding.
8.5. Cross-Browser Compatibility
jQuery Form Styler is compatible with a wide range of browsers, ensuring that your styled forms will work consistently for all your users.
Conclusion
Incorporating stylish and user-friendly forms is a crucial aspect of web development. jQuery Form Styler simplifies this process by allowing you to easily style form elements and enhance the user experience. Whether you’re working on a personal blog or a complex web application, this library can be a valuable addition to your toolkit. So, start using jQuery Form Styler today and take your web forms to the next level of aesthetics and usability. Your users will thank you for it!
Table of Contents
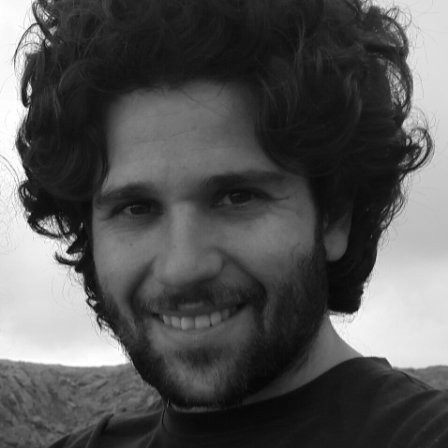
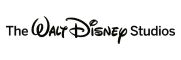