jQuery and FullCalendar: Building Event Scheduling Applications
In today’s fast-paced world, effective event scheduling is crucial for businesses and individuals alike. Whether you’re managing meetings, conferences, or personal appointments, having a well-designed event scheduling application can streamline your life and enhance your productivity.
Table of Contents
One of the best ways to create such applications is by combining the power of jQuery and FullCalendar. In this comprehensive guide, we’ll walk you through the process of building event scheduling applications with these two potent tools. You’ll discover how to create interactive and responsive calendars, manage events efficiently, and customize your application to suit your specific needs.
1. Introduction Event Scheduling Applications
1.1. The Importance of Event Scheduling Applications
Event scheduling applications have become an integral part of modern life. From personal calendars that help us keep track of birthdays and appointments to business applications that manage meetings and conferences, these tools enhance our organization and time management.
Such applications offer a range of benefits, including:
- Efficient Time Management: Event schedulers help individuals and organizations allocate their time effectively, reducing the risk of overbooking or missed appointments.
- Enhanced Collaboration: In business settings, scheduling applications facilitate collaboration by allowing teams to coordinate meetings and deadlines seamlessly.
- Improved Productivity: With event scheduling applications, you can prioritize tasks and allocate time for essential activities, increasing overall productivity.
- Data Insights: These applications often come with reporting and analytics features, providing valuable insights into your schedule and helping you make data-driven decisions.
1.2. Why Choose jQuery and FullCalendar?
Building an event scheduling application from scratch can be a daunting task, but the right tools can make it more manageable. jQuery and FullCalendar are two robust libraries that can simplify the process and empower you to create feature-rich, interactive calendars.
jQuery is a fast, small, and feature-rich JavaScript library. It simplifies HTML document traversal and manipulation, event handling, animation, and much more. By using jQuery, you can easily add interactivity and functionality to your web application without writing extensive JavaScript code.
FullCalendar is a powerful and customizable JavaScript calendar library that allows you to create dynamic event scheduling applications. It provides a wide range of features, including customizable views, drag-and-drop support, event rendering, and event editing. Combining jQuery with FullCalendar allows you to harness the strengths of both libraries to build a compelling event scheduling solution.
In the following sections, we’ll dive into the practical aspects of using jQuery and FullCalendar to create your event scheduling application.
2. Getting Started
2.1. Setting Up Your Development Environment
Before diving into code, it’s essential to set up your development environment. Ensure you have the following tools and resources in place:
- Code Editor:
Choose a code editor that suits your preferences. Popular options include Visual Studio Code, Sublime Text, and Atom.
- Web Server:
You’ll need a web server to serve your application locally during development. Consider using tools like XAMPP, WAMP, or simply Python’s built-in HTTP server.
- Browser:
For testing and debugging, have multiple browsers on hand. Chrome, Firefox, and Edge are commonly used for web development.
2.2. Including jQuery and FullCalendar in Your Project
jQuery and FullCalendar can be included in your project via Content Delivery Networks (CDNs) or by downloading the libraries and adding them locally.
Using CDNs:
html <!-- jQuery --> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> <!-- FullCalendar CSS --> <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/fullcalendar@5.9.0/main.css" /> <!-- FullCalendar JavaScript --> <script src="https://cdn.jsdelivr.net/npm/fullcalendar@5.9.0/main.js"></script>
Local Installation:
- Download jQuery from the official website and include it in your project directory.
- Download FullCalendar from the official website and include it in your project directory.
- Link the downloaded files in your HTML:
html <!-- jQuery --> <script src="path/to/jquery.min.js"></script> <!-- FullCalendar CSS --> <link rel="stylesheet" href="path/to/fullcalendar/main.css" /> <!-- FullCalendar JavaScript --> <script src="path/to/fullcalendar/main.js"></script>
2.3. Creating the Basic HTML Structure
Now that you have jQuery and FullCalendar set up in your project let’s create the basic HTML structure for your event scheduling application:
html <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Event Scheduler</title> <!-- Include FullCalendar CSS here --> </head> <body> <div id="calendar"></div> <!-- Include FullCalendar JavaScript here --> <script src="your-calendar-script.js"></script> </body> </html>
In this structure, we’ve added a div with the ID calendar where our FullCalendar will be rendered. We’ve also included placeholders for FullCalendar CSS and JavaScript, which we’ll add in the next sections as we start working on our calendar.
3. Creating a Simple Calendar
Now that we have the foundation set up, it’s time to create a simple calendar using FullCalendar and jQuery.
3.1. Initializing FullCalendar
In your your-calendar-script.js file, initialize FullCalendar with the following code:
javascript $(document).ready(function() { $('#calendar').fullCalendar({ // Options and configurations go here }); });
This code ensures that FullCalendar is initialized when the document is ready.
3.2. Displaying Events on the Calendar
To display events on the calendar, you need to provide event data. This data can come from various sources, such as a database or an API. For now, let’s create a static array of events for demonstration purposes:
javascript var events = [ { title: 'Meeting with Client', start: '2023-09-10T09:00:00', end: '2023-09-10T11:00:00', }, { title: 'Team Standup', start: '2023-09-11T10:30:00', end: '2023-09-11T11:00:00', }, // Add more events here ];
Now, let’s update the FullCalendar initialization code to include this event data:
javascript $(document).ready(function() { $('#calendar').fullCalendar({ events: events, // Other options and configurations }); });
With this code, FullCalendar will render the events on the calendar based on the provided data.
3.3. Customizing the Calendar’s Appearance
FullCalendar offers extensive customization options to tailor the calendar’s appearance to your needs. You can modify the header, change the default view, and apply custom styles.
For example, to change the default view to a month view and customize the header, you can use the following code:
javascript $(document).ready(function() { $('#calendar').fullCalendar({ events: events, defaultView: 'month', // Set the default view to 'month' header: { left: 'prev,next today', center: 'title', right: 'month,agendaWeek,agendaDay', }, // Other options and configurations }); });
In this code:
defaultView is set to ‘month’, making the month view the default when the calendar loads.
The header object defines the buttons and title in the calendar header. You can customize it to include the views you want.
With these changes, your calendar will display a month view by default, and you can switch between different views using the buttons in the header.
This is just the beginning of what you can do with FullCalendar. In the next section, we’ll explore how to manage events on the calendar.
4. Managing Events
4.1. Adding, Editing, and Deleting Events
Event management is a core feature of any event scheduling application. FullCalendar simplifies event management by providing built-in mechanisms for adding, editing, and deleting events.
4.2. Adding Events
To allow users to add new events to the calendar, you can create a form or a modal where they can input event details. When the user submits the form, you can capture the data and add a new event to the FullCalendar instance.
Here’s a simplified example of how you can add an event:
javascript // Assume you have a form with inputs for event title, start date, and end date $('#add-event-form').submit(function(e) { e.preventDefault(); var eventData = { title: $('#event-title').val(), start: $('#event-start').val(), end: $('#event-end').val(), }; $('#calendar').fullCalendar('renderEvent', eventData, true); // Add the event to the calendar // You can also save eventData to your data source (e.g., a database) // Clear the form fields or close the modal });
In this code:
- We prevent the default form submission to handle it via JavaScript.
- We capture the event data from the form inputs.
- We use the renderEvent method to add the event to the calendar. The third argument (true) indicates that the event should be “stickied” to the calendar, meaning it will persist even after navigating away from the page.
- You can optionally save the event data to your data source, such as a database.
4.3. Editing and Deleting Events
Editing and deleting events are also straightforward with FullCalendar. You can enable users to edit events by allowing them to click on an event and modify its details in a modal or a form.
To delete events, you can provide a delete button or an action menu within the event details, allowing users to remove events from the calendar.
Here’s a simplified example of how you can handle event click for editing and deletion:
javascript $('#calendar').fullCalendar({ // Other options and configurations eventClick: function(event) { // Handle event click for editing or deletion if (confirm('Do you want to delete this event?')) { $('#calendar').fullCalendar('removeEvents', event._id); // Delete the event from the calendar // You can also delete the event from your data source } else { // Open a modal or form for editing the event // Populate the modal/form with event data for editing } }, });
In this code:
- We use the eventClick callback to handle when an event is clicked on the calendar.
- If the user confirms the deletion, we use the removeEvents method to delete the event from the calendar. You can also delete the event from your data source if needed.
- If the user chooses not to delete the event, you can open a modal or form for editing the event. Populate the modal/form with the event’s data to allow the user to make changes.
- This covers the basic aspects of event management with FullCalendar. In a real-world application, you would likely implement more robust forms, error handling, and data validation.
5. Advanced Customization
While FullCalendar provides a lot of functionality out of the box, you might want to customize your calendar further to match your application’s unique requirements.
5.1. Styling Your Calendar
Customizing the calendar’s appearance can enhance the user experience and ensure it aligns with your application’s branding. You can style your calendar using CSS to change colors, fonts, borders, and more.
Here’s an example of how to change the background color of the calendar:
css /* Custom CSS for FullCalendar */ .fc { background-color: #f0f0f0; /* Set the background color */ }
You can override FullCalendar’s default styles by targeting specific CSS classes or elements.
5.2. Adding Event Categories and Colors
Categorizing events and using different colors to represent categories can make your calendar more visually informative. For instance, you can use different colors for personal, work, and family events.
First, add a category property to your event data:
javascript var events = [ { title: 'Meeting with Client', start: '2023-09-10T09:00:00', end: '2023-09-10T11:00:00', category: 'work', // Assign a category to the event }, // Add more events here ];
Next, you can define CSS classes and apply them to events based on their categories:
javascript $('#calendar').fullCalendar({ events: events, eventRender: function(event, element) { element.addClass(event.category); // Add a CSS class based on the event's category }, // Other options and configurations });
Now, you can use CSS to style events based on their categories:
css /* Custom CSS for event categories */ .work { background-color: #ff5733; /* Work events are red */ } .personal { background-color: #3498db; /* Personal events are blue */ } .family { background-color: #2ecc71; /* Family events are green */ }
By assigning categories and applying corresponding CSS classes, you can make it easier for users to distinguish between different types of events.
5.3. Implementing Filters and Views
To enhance the usability of your event scheduling application, consider adding filters and different calendar views.
5.3.1. Filters
Filters allow users to narrow down the events they see on the calendar. You can implement filters based on categories, dates, or other criteria.
Here’s an example of how to add a category filter:
html <!-- Filter buttons --> <div id="category-filters"> <button data-category="work">Work</button> <button data-category="personal">Personal</button> <button data-category="family">Family</button> </div>
And in your JavaScript code:
javascript // Filter events when a category button is clicked $('#category-filters button').on('click', function() { var category = $(this).data('category'); $('#calendar').fullCalendar('removeEventSource', events); // Remove current event source $('#calendar').fullCalendar('addEventSource', events.filter(function(event) { return event.category === category; })); });
In this code, when a category button is clicked, we filter the events based on the selected category and update the calendar accordingly.
5.3.2. Different Calendar Views
FullCalendar supports various calendar views, such as month view, week view, and day view. Users may prefer different views depending on their scheduling needs.
You can provide view buttons to allow users to switch between these views easily:
javascript $('#calendar').fullCalendar({ events: events, defaultView: 'month', // Set the default view views: { week: { titleFormat: 'MMMM D, YYYY', columnFormat: 'ddd D', }, day: { titleFormat: 'MMMM D, YYYY', columnFormat: 'dddd', }, }, header: { left: 'prev,next today', center: 'title', right: 'month,agendaWeek,agendaDay', }, // Other options and configurations });
In this code:
- We define different views (‘week’ and ‘day’) with customized title and column formats to make them more user-friendly.
- In the header section, we provide view buttons for month, week, and day views.
- With these additions, your users can easily switch between different calendar views and apply filters to focus on specific event categories.
6. Integrating User Authentication
Adding user authentication to your event scheduling application is essential if you want to provide personalized experiences and secure access to user-specific data.
6.1. Why User Authentication Matters
User authentication enables you to:
- Secure Data: Authenticate users to ensure that they can only access their own events and data. This prevents unauthorized access to sensitive information.
- Personalize User Experience: Authentication allows you to provide personalized calendars, settings, and preferences for each user.
- Track User Activity: With user accounts, you can track user activity, analyze usage patterns, and gain insights into how people interact with your application.
6.2. Implementing User Registration and Login
To implement user authentication, you’ll need a backend system that handles user registration, login, and session management. Common technologies for this purpose include Node.js with Express, Django, Ruby on Rails, and more.
Here’s an overview of the steps to implement user authentication:
6.2.1. User Registration:
- Create a registration form where users can provide their email, password, and other required information.
- When a user submits the form, send a POST request to your server to create a new user account in your database.
- Hash and securely store the user’s password using a strong hashing algorithm like bcrypt.
- Generate and store a unique session token or JWT (JSON Web Token) on the server and return it to the client as a response.
- Store the session token securely in the user’s browser, typically as an HTTP cookie.
6.2.2. User Login:
- Create a login form where users can enter their email and password.
- When a user submits the login form, send a POST request to your server with the provided credentials.
- Verify the user’s email and password against the stored hashed password in your database.
- If the credentials are valid, generate a new session token or JWT, update the user’s session on the server, and return the token to the client.
- Store the session token securely in the user’s browser upon successful login.
6.2.3. Securing Event Data:
- Associate each event with a user by storing the user’s unique identifier (e.g., user ID) along with the event data in your database.
- When a user requests their calendar data, authenticate the user by checking their session token or JWT.
- Retrieve and send only the events associated with the authenticated user.
- Ensure that your API endpoints for event data are protected and can only be accessed by authenticated users.
Implementing user authentication can be a complex task, and the specifics may vary depending on the programming language and framework you’re using on the backend. Consider using established authentication libraries and following security best practices to ensure the safety of user data.
7. Optimizing Performance
Performance optimization is crucial for ensuring that your event scheduling application runs smoothly, especially when dealing with large datasets or many concurrent users.
7.1. Dealing with Large Event Datasets
As your application grows, you may encounter performance challenges when handling a large number of events. To address this, consider the following strategies:
7.1.1. Lazy Loading:
Implement lazy loading to load events only when they are needed. For example, load events for the currently displayed month and fetch additional events as the user navigates to other months or views.
7.1.2. Pagination:
Implement event pagination to limit the number of events displayed on a single calendar view. Allow users to navigate between pages or load more events when needed.
7.1.3. Data Caching:
Cache event data on the client side to reduce the need for frequent server requests. When an event is updated, deleted, or added, update the cache accordingly.
7.2. Deployment and Hosting
Deploying your event scheduling application to a production environment involves several considerations:
7.2.1. Preparing Your Application for Deployment:
- Minify and bundle your JavaScript and CSS files to reduce load times.
- Optimize images and other assets for the web.
- Ensure that your application is running in production mode, which typically disables development-specific features and debugging tools.
7.2.2. Choosing a Hosting Solution:
Select a hosting solution that suits your needs. Common choices include:
- Shared Hosting: Suitable for small-scale applications with moderate traffic.
- Virtual Private Servers (VPS): Provides more control and resources compared to shared hosting.
- Cloud Hosting (e.g., AWS, Azure, Google Cloud): Offers scalability and resources on-demand.
- Platform as a Service (PaaS): Streamlines deployment and management but may have limitations on customization.
- Dedicated Servers: Provides full control and resources but requires more maintenance.
7.2.3. Deployment Best Practices:
- Configure your server to use HTTPS to secure data transmission.
- Set up regular backups of your application and database to prevent data loss.
- Monitor server performance and implement scaling strategies to handle increased traffic.
- Implement security measures such as firewalls, intrusion detection systems, and regular security audits.
Conclusion
In this comprehensive guide, we’ve explored the process of building event scheduling applications using jQuery and FullCalendar. We started with the basics, setting up your development environment and creating a simple calendar. Then, we delved into event management, advanced customization, user authentication, performance optimization, and deployment.
By harnessing the power of jQuery and FullCalendar, you can create feature-rich, interactive, and user-friendly event scheduling applications that cater to a wide range of needs. Whether you’re building a personal calendar or a business scheduling tool, these libraries provide a solid foundation for your project.
Remember that building a robust event scheduling application is an iterative process. Continuously gather user feedback and consider adding additional features and enhancements to meet the evolving needs of your users.
Now, armed with this knowledge, you’re ready to embark on your journey to create the next great event scheduling application. Happy coding!
Table of Contents
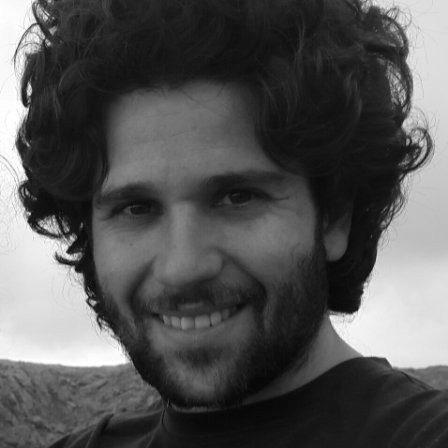
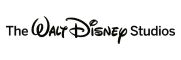