jQuery and GraphQL: Fetching Data with Ease
In the ever-evolving landscape of web development, staying on top of the latest technologies is crucial. Two such technologies that have gained immense popularity are jQuery and GraphQL. jQuery, a JavaScript library, simplifies HTML document traversal, event handling, and AJAX interactions. GraphQL, on the other hand, is a query language for APIs that offers a more efficient and flexible approach to fetching data from your server. In this blog post, we will explore how to harness the power of jQuery and GraphQL together to fetch data with ease in your web applications.
Table of Contents
Modern web applications demand efficient and flexible ways to fetch data from servers. jQuery and GraphQL are two powerful tools that can make this task significantly easier. By combining the simplicity of jQuery’s AJAX capabilities with the precision of GraphQL queries, you can streamline your data fetching process and create responsive, data-driven web applications.
In this comprehensive guide, we will dive into both technologies, explore their individual strengths, and then demonstrate how to use them together. Whether you’re a seasoned developer or just getting started, this blog will provide you with the knowledge and tools to fetch data with ease.
1. Understanding jQuery
1.1. What is jQuery?
jQuery is a fast, small, and feature-rich JavaScript library. It simplifies many of the complex tasks involved in web development, making it easier to navigate HTML documents, handle events, create animations, and interact with a server using AJAX (Asynchronous JavaScript and XML) requests.
1.2. Why use jQuery for Data Fetching?
jQuery’s AJAX functions are a game-changer when it comes to fetching data from a server. They provide a simple and consistent API for making asynchronous requests, whether it’s fetching data in JSON, XML, HTML, or even plain text. jQuery abstracts away many of the browser-specific quirks, making cross-browser compatibility a breeze.
2. Introducing GraphQL
2.1. What is GraphQL?
GraphQL is a query language for your API and a server-side runtime for executing those queries by using a type system you define for your data. Unlike traditional REST APIs, where the server defines the structure of the response, GraphQL allows clients to specify exactly what data they need, and nothing more.
2.2. Why use GraphQL for Data Fetching?
GraphQL offers several advantages over traditional REST APIs:
- Efficiency: Clients fetch only the data they need, reducing over-fetching and under-fetching of data.
- Flexibility: Clients can request multiple resources in a single query, reducing the number of requests to the server.
- Strongly Typed: GraphQL APIs are strongly typed, meaning the schema is well-defined, providing better tooling and documentation.
- Real-time Data: GraphQL supports real-time data with subscriptions, making it ideal for applications that require live updates.
Now that we have a basic understanding of jQuery and GraphQL, let’s set up our development environment.
3. Setting Up Your Environment
3.1. Installing jQuery
Before we dive into the code, you need to include jQuery in your project. You can do this by either downloading it and including it locally or by using a Content Delivery Network (CDN). Here’s how to include jQuery via a CDN:
html <!DOCTYPE html> <html> <head> <title>jQuery and GraphQL</title> <!-- Include jQuery via CDN --> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> </head> <body> <!-- Your HTML content here --> </body> </html>
3.2. Setting Up a GraphQL Server
To work with GraphQL, you’ll need a server that exposes a GraphQL API. There are various ways to set up a GraphQL server, but for this example, we’ll use Apollo Server, a popular choice among developers.
You can set up an Apollo Server with Node.js and Express with just a few lines of code:
javascript const { ApolloServer, gql } = require('apollo-server-express'); const express = require('express'); const typeDefs = gql` type Query { hello: String } `; const resolvers = { Query: { hello: () => 'Hello, GraphQL!' } }; const server = new ApolloServer({ typeDefs, resolvers }); const app = express(); server.applyMiddleware({ app }); app.listen({ port: 4000 }, () => console.log(`Server ready at http://localhost:4000${server.graphqlPath}`) );
With jQuery and Apollo Server set up, we can now move on to fetching data.
4. Fetching Data with jQuery
4.1. Making AJAX Requests
jQuery provides a straightforward way to make AJAX requests using the $.ajax() function. You can specify the URL you want to fetch data from and the HTTP method (GET, POST, PUT, DELETE, etc.).
Here’s a basic example of fetching data using jQuery:
javascript $.ajax({ url: '/api/data', method: 'GET', success: function (data) { console.log('Data received:', data); }, error: function (error) { console.error('Error:', error); } });
This code sends a GET request to ‘/api/data’ and logs the response data to the console.
4.2. Handling Responses
jQuery provides callback functions for handling responses, such as success and error. You can also use the .done() and .fail() methods for the same purpose. This makes it easy to process the data once it’s returned from the server.
Now, let’s explore how to fetch data with GraphQL.
5. Fetching Data with GraphQL
5.1. Constructing GraphQL Queries
In GraphQL, data fetching begins with constructing a query. Queries are structured to match the shape of the data you want to retrieve. Here’s an example of a GraphQL query for fetching a user’s name and email:
graphql { user(id: 123) { name email } }
This query requests the name and email fields for a user with the ID 123.
5.2. Sending GraphQL Queries
To send a GraphQL query, you make a POST request to the GraphQL server’s endpoint with the query as the request body. Here’s an example using JavaScript’s fetch API:
javascript fetch('/graphql', { method: 'POST', headers: { 'Content-Type': 'application/json', // You may need to include authentication headers if required }, body: JSON.stringify({ query: 'your-graphql-query-here' }) }) .then(response => response.json()) .then(data => console.log('Data received:', data)) .catch(error => console.error('Error:', error));
This code sends a GraphQL query to the ‘/graphql’ endpoint and logs the response data to the console.
5.3. Handling GraphQL Responses
GraphQL responses typically include a data property containing the requested data or an errors property if there are any issues with the query. It’s essential to handle these responses gracefully in your application.
Now that we know how to fetch data with both jQuery and GraphQL, let’s combine these technologies to create a sample application.
6. Combining jQuery and GraphQL
6.1. Building a Sample Application
For our sample application, let’s create a simple webpage that fetches and displays a list of books from a GraphQL server. We’ll use jQuery to make the AJAX request to the server.
First, let’s create a basic HTML structure:
html <!DOCTYPE html> <html> <head> <title>Book List</title> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> </head> <body> <h1>Book List</h1> <ul id="book-list"></ul> <script> // JavaScript code will go here </script> </body> </html>
Now, in the <script> section, let’s use jQuery to fetch data from our GraphQL server:
javascript $(document).ready(function () { $.ajax({ url: '/graphql', method: 'POST', headers: { 'Content-Type': 'application/json' }, data: JSON.stringify({ query: ` { books { title author } } ` }), success: function (response) { const books = response.data.books; const bookList = $('#book-list'); books.forEach(book => { const listItem = $('<li>'); listItem.text(`${book.title} by ${book.author}`); bookList.append(listItem); }); }, error: function (error) { console.error('Error:', error); } }); });
This code fetches a list of books from the GraphQL server and dynamically populates an unordered list with the book titles and authors.
Conclusion
In this blog post, we’ve explored how to harness the power of both jQuery and GraphQL to fetch data with ease in your web applications. jQuery simplifies AJAX requests and response handling, while GraphQL provides efficient and flexible data querying.
By combining these technologies, you can create web applications that fetch data precisely as needed, leading to improved performance and a better user experience. Whether you’re building a simple website or a complex web application, understanding how to use jQuery and GraphQL together can be a valuable skill in your web development toolkit.
So, go ahead and experiment with jQuery and GraphQL in your projects. As you gain more experience, you’ll discover countless ways to leverage their capabilities to build powerful and responsive web applications. Happy coding!
Table of Contents
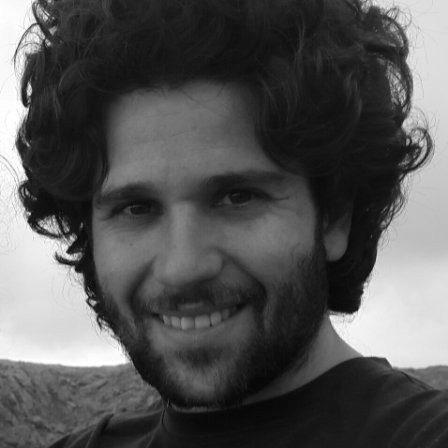
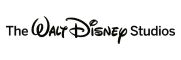