Handling Events with jQuery: A Deep Dive
Before we delve into jQuery’s event handling capabilities, let’s briefly understand what events are. In web development, events are occurrences that happen within the browser as a result of user actions or system triggers. These actions can be as simple as clicking a button, hovering over an element, pressing a key, or even resizing the window.
Event handling involves writing code that responds to these events, enabling developers to create interactive and responsive web applications. Handling events effectively is crucial for providing a seamless and enjoyable user experience.
1. Why Use jQuery for Event Handling?
jQuery, a fast and lightweight JavaScript library, has been a popular choice for event handling for several reasons:
- Cross-browser compatibility: jQuery abstracts away many browser-specific inconsistencies, ensuring that your event handling code works uniformly across different browsers.
- Simplified syntax: jQuery’s easy-to-read syntax allows developers to write concise code, reducing the amount of boilerplate required for event handling tasks.
- Broad community support: jQuery has a large and active community, providing ample resources, plugins, and support for developers.
- Chaining methods: jQuery’s method chaining enables developers to perform multiple operations on an element in a single line of code, making event handling more efficient.
Now that we understand the benefits of using jQuery for event handling, let’s explore some of the key concepts and techniques.
2. Event Binding with jQuery
Event binding is the process of associating an event with an element on the web page. In jQuery, the on() method is commonly used for event binding. The basic syntax for event binding with on() is as follows:
javascript $(selector).on(eventName, handlerFunction);
Where:
- selector: Specifies the element(s) to which the event should be bound.
- eventName: Represents the event to listen for, such as “click,” “hover,” “keydown,” etc.
- handlerFunction: The function that gets executed when the event is triggered.
Let’s look at an example of binding a “click” event to a button:
html <button id="myButton">Click Me</button> javascript $("#myButton").on("click", function() { // Code to execute when the button is clicked alert("Button clicked!"); });
In this example, when the button with the ID “myButton” is clicked, the anonymous function inside the on() method will be executed, showing an alert with the message “Button clicked!”.
3. Event Delegation
Event delegation is a powerful technique that allows us to attach a single event handler to a parent element to handle events for its child elements. This is particularly useful when working with dynamically generated content or large lists of items. By delegating the event handling to a parent element, we can reduce memory consumption and improve performance.
To use event delegation in jQuery, we apply the same on() method but specify the child selector as the second argument. This tells jQuery to listen for the specified event on the child elements that match the selector within the parent element.
javascript $("#parentElement").on("click", ".childElement", function() { // Code to execute when a child element is clicked });
In this example, any click on a child element within the “parentElement” will trigger the event handler.
4. Event Propagation: Bubbling and Capturing
In the DOM, events propagate through the tree structure of elements. Understanding event propagation is crucial when dealing with event handling, as it can affect how events are handled and where they are caught.
There are two phases of event propagation:
- Capturing phase: The event starts from the root element and moves towards the target element.
- Bubbling phase: The event moves from the target element back up towards the root element.
By default, most events in jQuery use the bubbling phase, meaning the event is first triggered on the target element and then propagated to its parent elements. However, in some cases, we may want to use the capturing phase, where the event is triggered on the root element and then propagated down to the target element. To use the capturing phase, we can set the third parameter of the on() method to true:
javascript $(selector).on(eventName, handlerFunction, true);
In most scenarios, capturing is not required, and using the bubbling phase is sufficient.
5. Event Object and Preventing Default Behavior
When an event is triggered, jQuery passes an event object to the event handler function. This object contains information about the event and methods to interact with it.
For example, if we want to prevent the default behavior of an event (e.g., prevent a form submission or a link click), we can use the preventDefault() method on the event object:
javascript $("#myLink").on("click", function(event) { event.preventDefault(); // Additional code to execute when the link is clicked });
In this example, clicking on the link with the ID “myLink” will not perform its default action, and the custom function will be executed instead.
6. Event Types and Examples
jQuery supports a wide range of events to cater to different user interactions. Here are some common event types with examples:
6.1. Click Event:
The “click” event is triggered when an element is clicked. It is widely used for buttons, links, and other interactive elements.
html <button id="clickButton">Click Me</button> javascript $("#clickButton").on("click", function() { // Code to execute when the button is clicked });
6.2. Hover Event:
The “hover” event is triggered when the mouse pointer enters or leaves an element.
html <div id="hoverElement">Hover over me</div> javascript $("#hoverElement").hover( function() { // Code to execute when the mouse enters the element }, function() { // Code to execute when the mouse leaves the element } );
6.3. Keydown Event:
The “keydown” event is triggered when a key on the keyboard is pressed.
html <input type="text" id="myInput" /> javascript $("#myInput").on("keydown", function(event) { console.log("Key pressed: " + event.key); });
These are just a few examples, and jQuery provides many other events to handle various user interactions effectively.
Conclusion
In this deep dive into handling events with jQuery, we’ve explored the importance of events in web development, the advantages of using jQuery for event handling, event binding, event delegation, event propagation, and examples of different event types. By mastering the art of handling events, you can create interactive, responsive, and user-friendly web applications.
jQuery’s event handling capabilities provide a robust and efficient way to manage user interactions, enabling you to build seamless and delightful web experiences. As you continue your journey in web development, practice and experiment with different event handling techniques to become a proficient jQuery developer.
Remember, the key to successful event handling is understanding your users’ needs and designing interactions that make their journey on your website enjoyable and intuitive. Happy coding!
Table of Contents
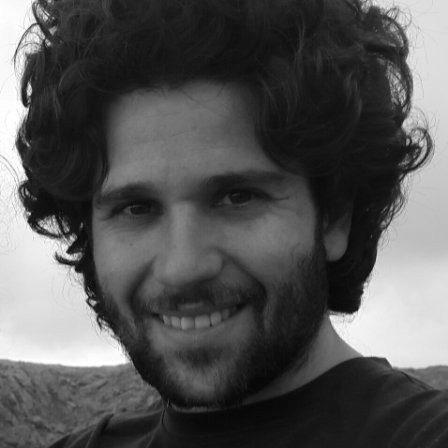
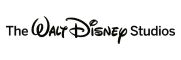