Image Galleries Made Easy with jQuery
When it comes to designing a visually appealing and interactive website, image galleries play a crucial role. They not only showcase your content but also engage your visitors, creating a lasting impression. However, creating image galleries from scratch can be a challenging task, especially for web developers who are not well-versed in advanced coding. Thankfully, jQuery comes to the rescue!
In this blog, we’ll explore how jQuery makes the process of building image galleries easier and more enjoyable. We’ll cover the fundamental concepts and provide practical examples to help you create beautiful, responsive, and user-friendly image galleries with minimal effort. Let’s dive in!
1. What is jQuery?
Before we delve into building image galleries, let’s take a moment to understand what jQuery is. jQuery is a fast, lightweight, and feature-rich JavaScript library that simplifies client-side scripting and HTML document traversal. It allows you to manipulate the HTML DOM (Document Object Model) with ease, making it an excellent choice for web developers looking to enhance the interactivity of their websites.
With jQuery, you can perform various operations, such as event handling, animation, and AJAX, but in this blog, we will focus on using jQuery for creating stunning image galleries.
2. Getting Started: Setting Up jQuery
To get started, you’ll need jQuery added to your project. You have a couple of options for doing this:
2.1. Downloading jQuery
Visit the official jQuery website (https://jquery.com/) and download the latest version of jQuery. Once downloaded, include it in your HTML file using the following script tag:
html <!DOCTYPE html> <html> <head> <title>Image Gallery with jQuery</title> <!-- Add the path to your local jQuery file --> <script src="path/to/jquery.js"></script> </head> <body> <!-- Your image gallery content goes here --> </body> </html>
2.2. Using a Content Delivery Network (CDN)
Alternatively, you can use a CDN to include jQuery in your project. Simply add the following script tag in your HTML file:
html <!DOCTYPE html> <html> <head> <title>Image Gallery with jQuery</title> <!-- Use the jQuery CDN link --> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> </head> <body> <!-- Your image gallery content goes here --> </body> </html>
Using a CDN can provide benefits such as faster loading times and increased chances of jQuery being cached by users who have previously visited other websites using the same CDN.
3. Creating a Basic Image Gallery
Let’s start by creating a basic image gallery. We’ll keep it simple and add more functionality as we progress. Here’s the basic HTML structure for our image gallery:
html <!DOCTYPE html> <html> <head> <title>Basic Image Gallery</title> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> </head> <body> <div class="image-gallery"> <img src="image1.jpg" alt="Image 1"> <img src="image2.jpg" alt="Image 2"> <img src="image3.jpg" alt="Image 3"> <!-- Add more images as needed --> </div> <script> // jQuery code for the image gallery goes here </script> </body> </html>
In the code above, we have a simple div with a class of “image-gallery” containing several img tags representing the images in our gallery.
4. Styling the Image Gallery
Before we add the jQuery code to make our image gallery interactive, let’s apply some basic CSS to improve its appearance:
css body { font-family: Arial, sans-serif; text-align: center; margin: 20px; } .image-gallery { display: flex; flex-wrap: wrap; gap: 10px; justify-content: center; } .image-gallery img { max-width: 200px; border: 1px solid #ccc; border-radius: 5px; cursor: pointer; transition: transform 0.2s ease; } .image-gallery img:hover { transform: scale(1.1); }
In the above CSS code, we center the gallery on the page, give it some margin, and use flexbox to align the images in a responsive grid layout. Additionally, we apply a border and a subtle border-radius to the images to make them visually appealing. When hovering over an image, we add a slight zoom-in effect using the transform property.
5. Creating a Lightbox for Image Display
A common feature in image galleries is a lightbox, which displays the selected image in a larger view when clicked. We’ll use jQuery to implement this feature. To do that, we need to update our HTML and add some more CSS:
html <!DOCTYPE html> <html> <head> <title>Image Gallery with Lightbox</title> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> </head> <body> <div class="image-gallery"> <img src="image1.jpg" alt="Image 1" data-large="large-image1.jpg"> <img src="image2.jpg" alt="Image 2" data-large="large-image2.jpg"> <img src="image3.jpg" alt="Image 3" data-large="large-image3.jpg"> <!-- Add more images as needed --> </div> <div class="lightbox"> <span class="close-btn">×</span> <img class="lightbox-img" src="" alt="Image"> </div> <script> // jQuery code for the image gallery and lightbox goes here </script> </body> </html>
In the updated HTML, we added a new div with a class of “lightbox” to hold the enlarged image and a close button. We also included a data-large attribute in each img tag to store the URL of the larger version of the image.
Next, let’s add some CSS for the lightbox:
css .lightbox { display: none; position: fixed; top: 0; left: 0; width: 100%; height: 100%; background-color: rgba(0, 0, 0, 0.9); z-index: 999; padding: 20px; box-sizing: border-box; } .lightbox-img { max-width: 100%; max-height: 80vh; margin: 0 auto; display: block; } .close-btn { position: absolute; top: 10px; right: 10px; color: #fff; font-size: 24px; cursor: pointer; }
In the CSS above, we style the lightbox container with a black semi-transparent background, position it at the top-left corner of the viewport, and set its width and height to cover the entire screen. The close button is styled to appear on the top-right corner of the lightbox. The max-height property ensures that the image scales down if it exceeds 80% of the viewport height, maintaining its aspect ratio.
6. Implementing the Lightbox Functionality with jQuery
Now that we have set up the HTML and CSS for the lightbox, it’s time to add the jQuery code to make it functional:
html <!DOCTYPE html> <html> <head> <title>Image Gallery with Lightbox</title> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> </head> <body> <div class="image-gallery"> <img src="image1.jpg" alt="Image 1" data-large="large-image1.jpg"> <img src="image2.jpg" alt="Image 2" data-large="large-image2.jpg"> <img src="image3.jpg" alt="Image 3" data-large="large-image3.jpg"> <!-- Add more images as needed --> </div> <div class="lightbox"> <span class="close-btn">×</span> <img class="lightbox-img" src="" alt="Image"> </div> <script> $(document).ready(function() { $(".image-gallery img").on("click", function() { const largeImageUrl = $(this).data("large"); $(".lightbox-img").attr("src", largeImageUrl); $(".lightbox").fadeIn(); }); $(".close-btn").on("click", function() { $(".lightbox").fadeOut(); }); }); </script> </body> </html>
The jQuery code above wraps the event handling logic inside $(document).ready() to ensure it runs after the document has finished loading. We use the .on(“click”) method to handle the click event on the gallery images. When an image is clicked, we retrieve its data-large attribute value, which holds the URL of the larger image, and update the src attribute of the lightbox image accordingly. The lightbox is then displayed using .fadeIn().
Additionally, we handle the click event on the close button (with the class “close-btn”) to hide the lightbox using .fadeOut().
7. Making the Image Gallery Responsive
A modern website needs to be responsive to accommodate various screen sizes and devices. Let’s update our image gallery and lightbox CSS to make them responsive:
css /* Previous CSS rules remain the same */ /* Responsive adjustments */ @media screen and (max-width: 768px) { .image-gallery { justify-content: flex-start; } .image-gallery img { max-width: 100%; } .lightbox-img { max-height: 60vh; } }
In the CSS above, we use a media query to adjust the image gallery and lightbox for screen widths up to 768 pixels. We change the justify-content property of the .image-gallery to flex-start so that the images stack vertically on smaller screens. The max-width of the images is set to 100%, allowing them to scale down and fit the screen width.
We also reduce the max-height of the .lightbox-img to 60% of the viewport height for better visibility on mobile devices.
8. Adding Image Captions
To enhance our image gallery further, let’s add captions to each image. We’ll use the alt attribute of the img tag to store the captions and display them in the lightbox:
html <!DOCTYPE html> <html> <head> <title>Image Gallery with Lightbox and Captions</title> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> </head> <body> <div class="image-gallery"> <img src="image1.jpg" alt="Image 1|Caption for Image 1" data-large="large-image1.jpg"> <img src="image2.jpg" alt="Image 2|Caption for Image 2" data-large="large-image2.jpg"> <img src="image3.jpg" alt="Image 3|Caption for Image 3" data-large="large-image3.jpg"> <!-- Add more images as needed --> </div> <div class="lightbox"> <span class="close-btn">×</span> <img class="lightbox-img" src="" alt="Image"> <div class="caption"></div> </div> <script> $(document).ready(function() { $(".image-gallery img").on("click", function() { const imageData = $(this).attr("alt").split("|"); const largeImageUrl = $(this).data("large"); const caption = imageData[1]; $(".lightbox-img").attr("src", largeImageUrl); $(".caption").text(caption); $(".lightbox").fadeIn(); }); $(".close-btn").on("click", function() { $(".lightbox").fadeOut(); }); }); </script> </body> </html>
We modified the alt attribute of each image by appending the caption after a pipe character (|). For example, alt=”Image 1|Caption for Image 1″. In the jQuery code, when an image is clicked, we split the alt attribute value to extract the caption and display it in the lightbox using .text().
Conclusion
Congratulations! You’ve successfully created a beautiful and interactive image gallery using jQuery. Starting with a basic gallery, you added a lightbox to display enlarged images and implemented responsiveness for optimal viewing across devices. Additionally, you enhanced the gallery by adding captions for each image.
jQuery provides a powerful set of tools that simplifies the process of creating interactive web elements, like image galleries, without requiring extensive knowledge of JavaScript or complex coding. Experiment further with jQuery and explore its vast library to bring even more exciting features to your website!
Remember to keep your code organized and maintainable as your gallery grows. Happy coding!
Table of Contents
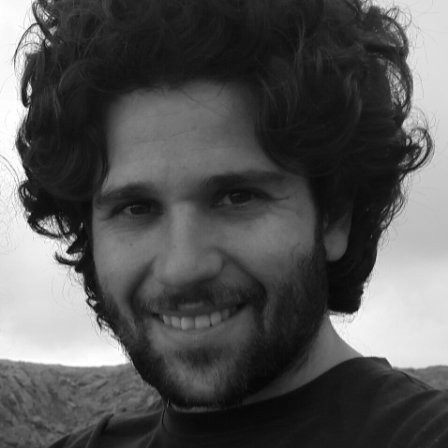
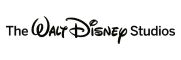