Creating Beautiful Image Galleries with jQuery Lightbox
In the ever-evolving landscape of web design, aesthetics play a pivotal role in capturing the attention of users and keeping them engaged. One effective way to enhance your website’s visual appeal is by incorporating captivating image galleries. These galleries not only showcase your content but also provide a seamless and immersive experience for your visitors. One popular tool for achieving this is jQuery Lightbox – a versatile and user-friendly solution that allows you to create beautiful and interactive image galleries. In this article, we’ll guide you through the process of creating stunning image galleries using jQuery Lightbox, complete with code samples and step-by-step instructions.
Table of Contents
1. Why jQuery Lightbox?
Before delving into the process of creating image galleries, it’s important to understand the merits of using jQuery Lightbox. This lightweight and feature-rich JavaScript library provides an elegant solution for displaying images and other multimedia content in a visually appealing manner. Some of the key advantages include:
1.1. User-Friendly Interaction
jQuery Lightbox offers a user-friendly way to present images. When a thumbnail is clicked, the full-size image is displayed in a popup overlay, allowing users to focus solely on the content without navigating to a new page.
1.2. Mobile Responsiveness
Modern websites must cater to users on various devices. jQuery Lightbox ensures that your image galleries remain responsive and accessible on smartphones, tablets, and desktops, providing a consistent experience across different platforms.
1.3. Customization Options
Customization is key to aligning the image gallery with your website’s design. jQuery Lightbox allows you to configure various settings, such as transitions, animations, and captions, enabling you to maintain a consistent visual identity.
1.4. Improved Loading Performance
By loading images dynamically in a popup, jQuery Lightbox optimizes the loading performance of your website. This prevents users from waiting for large images to load when accessing your galleries.
2. Getting Started: Implementing jQuery Lightbox
Let’s dive into the practical aspects of creating image galleries using jQuery Lightbox. Follow these steps to get started:
Step 1: Include jQuery and jQuery Lightbox
To begin, make sure you have jQuery and jQuery Lightbox included in your HTML file. You can include them via a content delivery network (CDN) or by downloading the files and hosting them on your server. Here’s an example of how to include them using CDNs:
html <!DOCTYPE html> <html> <head> <!-- Include jQuery --> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> <!-- Include jQuery Lightbox CSS --> <link rel="stylesheet" href="path/to/jquery.lightbox.css"> <!-- Include jQuery Lightbox JS --> <script src="path/to/jquery.lightbox.min.js"></script> <title>Image Gallery with jQuery Lightbox</title> </head> <body> <!-- Your gallery content goes here --> </body> </html>
Replace “path/to/jquery.lightbox.css” and “path/to/jquery.lightbox.min.js” with the actual paths to the jQuery Lightbox CSS and JS files on your server.
Step 2: Structure Your Gallery
Create the structure for your image gallery. This usually involves a list of thumbnail images that users can click on to view the full-size versions. Here’s an example structure:
html <div class="image-gallery"> <a href="path/to/image1.jpg" data-lightbox="gallery"> <img src="path/to/thumbnail1.jpg" alt="Image 1"> </a> <a href="path/to/image2.jpg" data-lightbox="gallery"> <img src="path/to/thumbnail2.jpg" alt="Image 2"> </a> <!-- Add more images here --> </div>
In this example, each <a> tag wraps an <img> tag. The href attribute of the <a> tag points to the full-size image, while the data-lightbox attribute specifies the gallery name. This allows jQuery Lightbox to group related images together.
Step 3: Initialize jQuery Lightbox
Initialize jQuery Lightbox by writing a short JavaScript snippet. Place this script just before the closing </body> tag in your HTML:
html <script> $(document).ready(function() { $('.image-gallery a').lightbox(); }); </script>
This code snippet waits for the document to be ready, then selects all <a> tags within the .image-gallery class and applies the jQuery Lightbox functionality to them.
3. Customizing Your Image Gallery
One of the standout features of jQuery Lightbox is its customization options. You can tailor the appearance and behavior of your image gallery to match your website’s aesthetics. Here are some customization possibilities:
3.1. Adding Captions
Captions provide context to your images and enhance the user experience. To add captions to your images, modify the <a> tags like this:
html <a href="path/to/image1.jpg" data-lightbox="gallery" data-title="Beautiful Sunset"> <img src="path/to/thumbnail1.jpg" alt="Image 1"> </a>
The data-title attribute adds a caption that will be displayed when the image is viewed in the lightbox.
3.2. Transition Effects
You can choose from various transition effects to make the opening and closing of the lightbox more engaging. Customize the transitions by initializing the lightbox like this:
html <script> $(document).ready(function() { $('.image-gallery a').lightbox({ animation: 'fade' }); }); </script>
Here, ‘fade’ is just one example of the available transition effects.
3.3. Keyboard Navigation
jQuery Lightbox also supports keyboard navigation, allowing users to navigate through images using the arrow keys. This feature enhances the overall user experience. It’s automatically enabled, so you don’t need to add any extra code.
Conclusion
Incorporating visually appealing image galleries can significantly enhance your website’s aesthetic appeal and user engagement. jQuery Lightbox provides an elegant solution for creating such galleries with minimal effort. By following the steps outlined in this article, you can easily implement jQuery Lightbox on your website and customize it to align with your design preferences. Remember to consider the user experience and responsiveness while building your galleries, as these factors contribute to a seamless and enjoyable browsing experience for your visitors. Elevate your website’s visual storytelling by harnessing the power of jQuery Lightbox – a versatile tool that turns ordinary image displays into captivating visual experiences.
Table of Contents
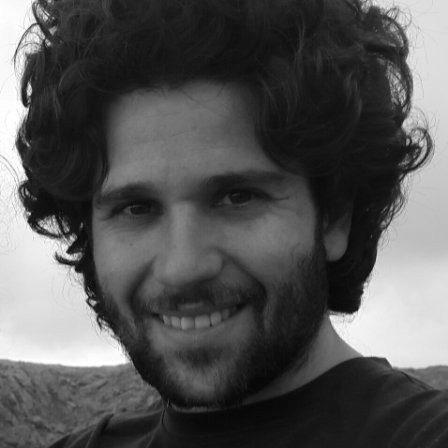
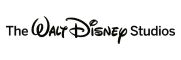