Implementing Infinite Scrolling with jQuery
Infinite scrolling is a popular web design technique that allows you to load content continuously as users scroll down a web page. This not only enhances the user experience by eliminating the need for pagination but also makes your website feel more dynamic and engaging. In this tutorial, we will explore how to implement infinite scrolling with jQuery, a widely-used JavaScript library, and provide you with step-by-step instructions and code samples to get you started.
Table of Contents
1. Prerequisites
Before we dive into the implementation, make sure you have the following prerequisites in place:
2. Basic knowledge of HTML, CSS, and JavaScript.
- A text editor or integrated development environment (IDE) for writing code.
- A web server or a local development environment for testing your code.
3. Setting Up Your Project
Let’s start by setting up a basic project structure. Create a directory for your project and set up the following files:
- index.html: The main HTML file.
- style.css: The CSS file for styling.
- script.js: The JavaScript file where we’ll implement infinite scrolling.
- data.js (optional): A file containing the data you want to load dynamically. For this tutorial, we’ll use a simple array as our data source.
Your project directory structure should look like this:
plaintext project-folder/ ??? index.html ??? style.css ??? script.js ??? data.js
4. Building the HTML Structure
In your index.html file, create a basic HTML structure. We’ll create a container for our content and an initial set of items to load.
html <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Infinite Scrolling with jQuery</title> <link rel="stylesheet" href="style.css"> </head> <body> <div class="content"> <div class="item">Item 1</div> <div class="item">Item 2</div> <div class="item">Item 3</div> <!-- More items will be added dynamically --> </div> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> <script src="script.js"></script> </body> </html>
In this example, we have a container with some initial items (Item 1, Item 2, and Item 3). The goal is to load additional items as the user scrolls down the page.
5. Styling Your Content
To make your content visually appealing, you can style it using CSS. Create a style.css file and define some basic styles for your items and the container:
css /* style.css */ .content { width: 80%; max-width: 600px; margin: 0 auto; padding: 20px; } .item { border: 1px solid #ccc; padding: 10px; margin: 10px 0; background-color: #f9f9f9; }
This CSS code styles the content container and items. Feel free to customize the styles to match your website’s design.
6. Implementing Infinite Scrolling with jQuery
Now, let’s dive into the JavaScript part and implement infinite scrolling using jQuery in the script.js file. We’ll use jQuery to detect when the user reaches the bottom of the page and load more items dynamically.
javascript // script.js $(document).ready(function() { const content = $('.content'); const data = [ 'Item 4', 'Item 5', 'Item 6', 'Item 7', 'Item 8', 'Item 9', 'Item 10' ]; const itemsPerLoad = 3; // Number of items to load at a time let currentIndex = 0; // Index to keep track of the data array // Function to load more items function loadItems() { const startIndex = currentIndex; const endIndex = currentIndex + itemsPerLoad; for (let i = startIndex; i < endIndex && i < data.length; i++) { content.append(`<div class="item">${data[i]}</div>`); } currentIndex = endIndex; } // Initial load loadItems(); // Detect when the user scrolls to the bottom $(window).scroll(function() { if ($(window).scrollTop() + $(window).height() >= content.height() - 100) { loadItems(); } }); });
In this script:
- We use $(document).ready() to ensure that our JavaScript code runs after the HTML document is fully loaded.
- We define the content variable to store the content container and create an array called data to hold our sample data.
- itemsPerLoad specifies the number of items to load each time the user reaches the bottom of the page.
- currentIndex keeps track of the current index in the data array.
- The loadItems function loads more items into the content container by appending them to it. It starts from the current index and adds items up to the endIndex. It also updates the currentIndex accordingly.
- We call loadItems once to initially load some items.
- The $(window).scroll() function detects when the user scrolls down the page. When the user is close to the bottom (within 100 pixels in this example), we call loadItems again to load more items.
7. Testing Your Infinite Scrolling
Now that you’ve implemented infinite scrolling with jQuery, it’s time to test your project. Open your index.html file in a web browser, and you should see the initial items loaded. As you scroll down the page, more items will be loaded automatically, providing a seamless scrolling experience.
8. Customization and Enhancements
You can customize and enhance your infinite scrolling implementation in several ways:
8.1. Loading Animation
You can add a loading animation or spinner to indicate to users that more content is being loaded. This provides visual feedback and improves the user experience.
8.2. Data Source
In this example, we used a simple array as the data source. In a real-world scenario, you might fetch data from a server using AJAX calls. Make sure to update the loadItems function to handle your specific data source.
8.3. Load Trigger
You can adjust the trigger point for loading more items by changing the $(window).height() >= content.height() – 100 condition. Experiment with different values to find the optimal user experience.
8.4. Endless Scrolling
If you have a large amount of data, consider implementing endless scrolling, where new data is fetched as the user scrolls, without reaching an end point. This can create a more immersive experience.
8.5. Error Handling
Handle errors gracefully, especially when fetching data from external sources. Display an error message or retry loading if the initial request fails.
Conclusion
Infinite scrolling with jQuery is a powerful technique that can significantly improve the user experience on your website by eliminating the need for traditional pagination. With the steps outlined in this tutorial, you can easily implement infinite scrolling in your projects, making your content more engaging and dynamic. Remember to customize and enhance the implementation to suit your specific requirements, and don’t forget to test thoroughly to ensure a seamless user experience. Happy coding!
Table of Contents
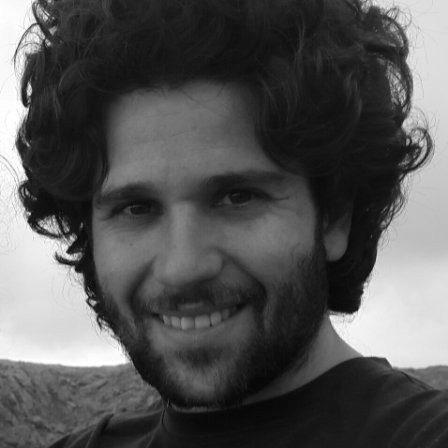
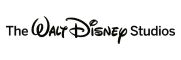