Integrating jQuery with Backend Technologies: PHP, Ruby, and More
In today’s web development landscape, creating interactive and dynamic user interfaces is crucial to delivering a seamless user experience. jQuery, a popular JavaScript library, has been a go-to choice for developers seeking to add rich functionalities and interactivity to their websites. While jQuery primarily focuses on the frontend, it can be seamlessly integrated with various backend technologies to create powerful web applications.
This blog post will guide you through the process of integrating jQuery with backend technologies like PHP, Ruby, and more. We’ll explore how these technologies can work together to build feature-rich applications that respond to user interactions, handle server-side tasks, and deliver a delightful user experience. Let’s dive in!
Understanding jQuery: A Brief Overview
Before we delve into integrating jQuery with backend technologies, let’s quickly review what jQuery is and why it’s so widely used. jQuery is a fast and lightweight JavaScript library that simplifies HTML document traversal, event handling, and animation. It abstracts away many complexities of JavaScript, making it easier for developers to interact with the DOM and create dynamic web pages effortlessly.
Prerequisites
To follow along with the examples in this blog post, you’ll need a basic understanding of HTML, CSS, JavaScript, and the backend technology of your choice (PHP, Ruby, etc.). Ensure that you have jQuery included in your project by either downloading it from the jQuery website or using a CDN.
Now, let’s explore how to integrate jQuery with various backend technologies.
Section 1: Integrating jQuery with PHP
Step 1: Setting up a PHP Backend
To get started with jQuery and PHP integration, you need a working PHP backend. For simplicity, let’s assume you have a basic PHP server set up with a file named backend.php that will handle the backend tasks.
php // backend.php <?php if ($_POST['action'] === 'get_data') { // Perform server-side tasks, such as querying a database. $data = ['item1', 'item2', 'item3']; // Return JSON response to the frontend. echo json_encode($data); } ?>
Step 2: Making AJAX Requests
With the PHP backend in place, let’s use jQuery to make an AJAX request to fetch data from the server and display it on the frontend.
html <!DOCTYPE html> <html> <head> <title>jQuery PHP Integration</title> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> </head> <body> <h1>Fetching Data from PHP Backend</h1> <ul id="dataList"></ul> <script> $(document).ready(function () { // Making an AJAX request to the backend. $.ajax({ url: 'backend.php', type: 'POST', data: { action: 'get_data' }, dataType: 'json', success: function (data) { // Displaying the received data on the frontend. data.forEach(function (item) { $('#dataList').append('<li>' + item + '</li>'); }); }, error: function (xhr, status, error) { console.error(error); } }); }); </script> </body> </html>
In this example, we use $.ajax to send a POST request to backend.php with the action get_data. The backend processes the request and returns a JSON response with some sample data. The received data is then displayed as a list on the frontend.
Section 2: Integrating jQuery with Ruby on Rails
Step 1: Create a Ruby on Rails Project
First, make sure you have Ruby and Ruby on Rails installed on your system. Create a new Rails project and set up a controller to handle the AJAX requests.
bash $ rails new my_rails_app $ cd my_rails_app $ rails generate controller DataController
Step 2: Implementing the Backend
In the DataController, define an action to handle the AJAX request and return data.
ruby # app/controllers/data_controller.rb class DataController < ApplicationController def get_data # Perform server-side tasks, such as querying a database. data = ['item1', 'item2', 'item3'] # Return JSON response to the frontend. render json: data end end
Step 3: Setting up Routes
Configure the routes to map the get_data action to a URL.
ruby # config/routes.rb Rails.application.routes.draw do post 'get_data', to: 'data#get_data' end
Step 4: Making AJAX Requests
Finally, let’s use jQuery to make AJAX requests to the Ruby on Rails backend and display the data on the frontend.
html <!DOCTYPE html> <html> <head> <title>jQuery Ruby on Rails Integration</title> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> </head> <body> <h1>Fetching Data from Ruby on Rails Backend</h1> <ul id="dataList"></ul> <script> $(document).ready(function () { // Making an AJAX request to the backend. $.ajax({ url: '/get_data', type: 'POST', dataType: 'json', success: function (data) { // Displaying the received data on the frontend. data.forEach(function (item) { $('#dataList').append('<li>' + item + '</li>'); }); }, error: function (xhr, status, error) { console.error(error); } }); }); </script> </body> </html>
Here, we use $.ajax to send a POST request to the /get_data URL mapped to the get_data action in the DataController. The backend processes the request, returns a JSON response with the sample data, and the frontend displays it as a list.
Section 3: Integrating jQuery with Other Backend Technologies
The process of integrating jQuery with other backend technologies is quite similar to what we’ve seen with PHP and Ruby on Rails. The main difference lies in how the backend handles AJAX requests and returns data.
For instance, if you’re using Node.js with Express, you would create an endpoint in your Express app to handle AJAX requests and send the response accordingly.
Conclusion
Integrating jQuery with backend technologies like PHP, Ruby, and more is essential for creating feature-rich web applications that offer seamless user experiences. By combining the power of jQuery’s frontend interactivity with the backend’s server-side capabilities, developers can build dynamic and responsive web applications.
In this blog post, we explored how to integrate jQuery with PHP and Ruby on Rails, two popular backend technologies. Remember, the process is quite similar with other backend technologies as well. So, whether you’re using Node.js, Python, or any other backend language, you can follow the same principles and techniques to achieve successful jQuery integration.
Now that you have a solid understanding of jQuery integration with backend technologies, go ahead and experiment with different frameworks and languages to build the next generation of interactive web applications! Happy coding!
Table of Contents
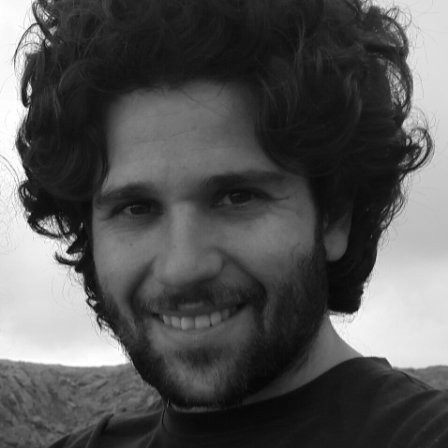
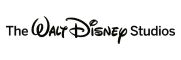