Creating Interactive Forms with jQuery Form Validation
Forms are a fundamental part of web applications, serving as a bridge for user interaction with your website. However, if not handled correctly, forms can lead to a frustrating user experience and unreliable data submission. jQuery form validation is a powerful tool that allows you to enhance the interactivity and reliability of your forms. In this comprehensive guide, we will delve into the world of interactive forms and explore how to implement jQuery form validation for seamless user experiences.
Table of Contents
1. Why jQuery for Form Validation?
jQuery is a popular JavaScript library known for its simplicity and versatility. It’s an ideal choice for form validation due to its ease of use and robust set of features. By leveraging jQuery, you can create dynamic, interactive forms that respond to user input in real-time, improving the overall user experience and data quality.
1.1. Getting Started with jQuery
Before diving into form validation, let’s ensure you have the basics in place. Include jQuery in your project by adding the following script tag to your HTML document’s <head> section:
html <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
Now, you’re ready to start creating interactive forms with jQuery form validation.
2. Basic Form Structure
Before applying validation, let’s set up a basic HTML form. Here’s a simple example:
html <form id="myForm"> <label for="name">Name:</label> <input type="text" id="name" name="name" required> <label for="email">Email:</label> <input type="email" id="email" name="email" required> <label for="password">Password:</label> <input type="password" id="password" name="password" required> <input type="submit" value="Submit"> </form>
This form includes fields for name, email, and password, with the required attribute ensuring that users cannot submit the form without filling in these fields.
3. jQuery Form Validation Basics
jQuery form validation is all about defining rules and behaviors for your form elements. You can specify which fields are required, set minimum and maximum lengths, and even create custom validation functions.
3.1. Selecting Form Elements
To begin, you need to select the form and its elements using jQuery. This allows you to attach validation rules and actions to specific fields. Here’s how you can select the form and input fields from our previous example:
javascript $(document).ready(function() { var form = $('#myForm'); var nameInput = $('#name'); var emailInput = $('#email'); var passwordInput = $('#password'); });
By wrapping your code in $(document).ready(), you ensure that your JavaScript runs after the DOM has fully loaded.
3.2. Adding Validation Rules
Now, let’s define some basic validation rules. For instance, you can ensure that the name field contains only letters:
javascript nameInput.on('input', function() { var value = $(this).val(); var pattern = /^[A-Za-z]+$/; if (!pattern.test(value)) { // Display an error message or take other actions } else { // Clear any previous error messages } });
In this code, we use the on method to listen for input events on the name field. We then check if the input matches our regular expression pattern. If not, you can display an error message or perform other actions as needed.
3.3. Handling Form Submission
Validation is essential, but you also need to handle form submission correctly. Prevent the form from submitting if there are validation errors:
javascript form.submit(function(event) { // Your validation code here if (/* Validation fails */) { event.preventDefault(); // Prevent form submission } });
In the submit event handler, you can perform your validation checks. If any checks fail, call event.preventDefault() to stop the form from being submitted.
3.4. Displaying Error Messages
To provide a better user experience, it’s essential to display error messages when validation fails. You can create a <div> element to hold these messages and dynamically show or hide it as needed:
html <div id="nameError" class="error-message"></div> javascript Copy code nameInput.on('input', function() { var value = $(this).val(); var pattern = /^[A-Za-z]+$/; var errorContainer = $('#nameError'); if (!pattern.test(value)) { errorContainer.text('Name must contain only letters'); } else { errorContainer.text(''); } });
In this example, we use the text method to update the error message in the errorContainer <div>.
4. Advanced Validation Techniques
While the basics cover many scenarios, you may encounter more complex validation requirements. Let’s explore some advanced techniques.
4.1. Custom Validation Functions
Sometimes, you need to perform custom validation that can’t be achieved with regular expressions or built-in HTML attributes. In such cases, define your custom validation function:
javascript function isStrongPassword(password) { // Implement your custom password strength check } passwordInput.on('input', function() { var value = $(this).val(); var errorContainer = $('#passwordError'); if (!isStrongPassword(value)) { errorContainer.text('Password must be at least 8 characters and include numbers and special characters.'); } else { errorContainer.text(''); } });
Here, isStrongPassword is a custom function that checks if the password meets specific criteria.
4.2. Real-Time Validation
Enhance user experience by providing real-time feedback as users fill out the form. For instance, you can check if an email address is available as the user types:
javascript var emailAvailable = false; emailInput.on('input', function() { var value = $(this).val(); var errorContainer = $('#emailError'); if (!isValidEmail(value)) { errorContainer.text('Invalid email address'); } else if (!emailAvailable) { errorContainer.text('Email address already in use'); } else { errorContainer.text(''); } });
In this example, we assume there’s a function isValidEmail that checks if the email format is valid and a variable emailAvailable that indicates whether the email address is available.
5. Handling Form Submission
Once you’ve implemented all necessary validation checks, it’s time to handle form submission. Ensure that the form only submits when all validations pass:
javascript form.submit(function(event) { var nameValue = nameInput.val(); var emailValue = emailInput.val(); var passwordValue = passwordInput.val(); var nameValid = /* Check if name is valid */; var emailValid = /* Check if email is valid */; var passwordValid = /* Check if password is valid */; if (!nameValid || !emailValid || !passwordValid) { event.preventDefault(); // Prevent form submission // Display error messages if necessary } });
In this code, we check the validity of each field and prevent form submission if any validation fails. You can also display error messages as needed.
6. Styling Your Form
A well-styled form not only looks appealing but also enhances the user experience. You can use CSS to style your form elements and error messages:
css .error-message { color: red; font-size: 14px; margin-top: 5px; }
Apply this CSS class to your error message containers to make them visually distinct.
Conclusion
jQuery form validation is a powerful tool for creating interactive forms that provide a smooth user experience. By implementing validation rules, handling form submission, and providing real-time feedback, you can ensure that users submit accurate and complete data.
Remember that while jQuery simplifies the process, it’s essential to consider accessibility and user-friendliness when designing your forms. Additionally, always test your forms thoroughly to catch any edge cases or unexpected behavior.
Now that you’ve learned the basics of jQuery form validation, you’re well-equipped to build interactive forms that elevate your web applications.
So, go ahead and start creating forms that not only function flawlessly but also provide an enjoyable user experience!
In this blog post, we explored the art of creating interactive forms with jQuery form validation. We started with the basics of setting up a form and then delved into jQuery’s powerful features for adding validation rules, handling form submission, and displaying error messages. Additionally, we covered advanced techniques like custom validation functions and real-time validation, ensuring that your forms meet even the most complex requirements. Remember to style your forms to enhance their visual appeal and user-friendliness. With the knowledge gained from this guide, you can confidently design forms that provide a seamless user experience and reliable data submission. Happy form building!
Table of Contents
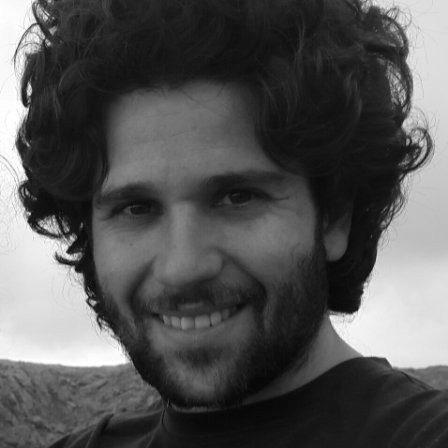
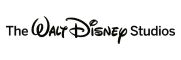