Creating Interactive Image Maps with jQuery
Images are a powerful way to convey information and engage users on a website. However, static images can sometimes fall short when it comes to interactivity and engagement. That’s where interactive image maps come into play. Interactive image maps allow you to create clickable regions within an image, turning it into an interactive canvas that users can explore and interact with. In this tutorial, we’ll explore how to create these engaging interactive image maps using jQuery, a popular JavaScript library.
Table of Contents
1. Why Use Interactive Image Maps?
Interactive image maps offer a plethora of benefits that can enhance the user experience on your website. They provide a visually appealing way to present information and can be particularly useful for:
- Navigation: Instead of a traditional menu, you can use an interactive image map to let users click on different regions of an image to navigate to different pages or sections of your site.
- Product Showcase: If you’re running an e-commerce website, you can use image maps to highlight specific features of a product. Users can click on different parts of an image to learn more about its functionalities.
- Educational Content: Interactive image maps are an excellent tool for creating educational content. You can use them to label different parts of an image and provide detailed explanations when users click on those labels.
2. Getting Started with Interactive Image Maps
To create interactive image maps, we’ll be using jQuery along with HTML and CSS. jQuery simplifies the process of handling user interactions and DOM manipulation. Here’s a step-by-step guide to creating your own interactive image map:
Step 1: Prepare Your Image
Start by choosing the image you want to make interactive. This could be a map, a diagram, an artwork, or any image that you want users to engage with. Make sure the image is clear and has distinct regions that you want to make clickable.
Step 2: HTML Structure
html <!DOCTYPE html> <html> <head> <title>Interactive Image Map with jQuery</title> <link rel="stylesheet" href="styles.css"> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> <script src="script.js"></script> </head> <body> <div class="image-container"> <img src="your-image.jpg" alt="Interactive Image"> <div class="clickable-area" data-target="region1"></div> <div class="clickable-area" data-target="region2"></div> <!-- Add more clickable areas as needed --> </div> </body> </html>
In this example, we’ve created a basic HTML structure. We have an image container that holds the image and multiple div elements with the class clickable-area. We’re using the data-target attribute to specify the target region for each clickable area.
Step 3: CSS Styling
Style your clickable areas using CSS to make them visible and overlay them on top of your image.
css /* styles.css */ .image-container { position: relative; } .clickable-area { position: absolute; background-color: rgba(0, 0, 0, 0.3); cursor: pointer; /* Define dimensions and positions for each clickable area */ } /* Style each clickable area differently if needed */ .clickable-area[data-target="region1"] { top: 20%; left: 30%; width: 20%; height: 15%; } .clickable-area[data-target="region2"] { top: 50%; left: 50%; width: 15%; height: 10%; }
Adjust the positioning and dimensions of the clickable areas according to your image’s layout.
Step 4: jQuery Magic
Now, let’s add interactivity using jQuery.
javascript // script.js $(document).ready(function() { $('.clickable-area').click(function() { var target = $(this).data('target'); // Perform actions when a clickable area is clicked // You can show a tooltip, navigate to a page, or display information }); });
In this code, we use jQuery to target all elements with the class .clickable-area. When a user clicks on a clickable area, the specified function is triggered. You can customize this function to perform actions like showing a tooltip or navigating to a specific page.
3. Customizing and Enhancing Interaction
To make your interactive image map even more engaging, consider these additional features:
- Tooltips: Display tooltips with information about the clicked regions. You can use CSS and jQuery to create and show tooltips when a user clicks on a clickable area.
- Highlighting: Change the appearance of the clickable areas when a user hovers over them. This visual feedback enhances the user experience and indicates interactivity.
- Responsive Design: Ensure that your interactive image map works well on various screen sizes. Use CSS media queries to adapt the layout for different devices.
Conclusion
Interactive image maps are a creative way to engage users and provide them with an interactive experience on your website. With jQuery, you can easily add interactivity to your images, making them more than just static visuals. By following the steps outlined in this tutorial, you’ll be able to create your own interactive image maps and customize them to suit your website’s needs. Whether you’re building a navigation tool, showcasing products, or creating educational content, interactive image maps can significantly enhance user engagement and interaction. So, go ahead and start transforming your images into captivating interactive experiences using jQuery!
Table of Contents
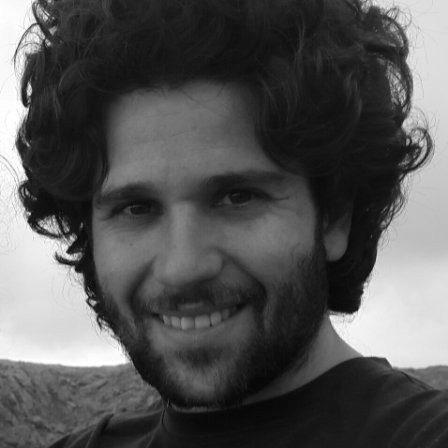
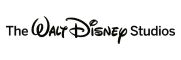