jQuery and JSON: Manipulating Data Like a Pro
In the world of web development, efficient data manipulation is a cornerstone of creating dynamic and interactive applications. Enter jQuery and JSON, two powerful tools that, when combined, offer a potent solution for handling and manipulating data seamlessly. Whether you’re a seasoned developer or just getting started, understanding how to utilize these tools can significantly enhance your web development prowess. In this article, we’ll delve into the world of jQuery and JSON, exploring their features and demonstrating how to wield them effectively for data manipulation.
Table of Contents
1. What is jQuery?
1.1. Introduction to jQuery
jQuery, often referred to as a “write less, do more” library, is a fast and concise JavaScript library designed to simplify the interaction between HTML documents and JavaScript code. It abstracts many complex and cross-browser compatible tasks into easily manageable functions, making it an indispensable tool for web developers aiming to streamline their projects.
1.2. The Power of Selectors
One of jQuery’s standout features is its powerful selectors. Selectors allow you to target specific HTML elements based on their attributes, classes, IDs, and more. This makes manipulating and interacting with elements a breeze. Consider the following example:
javascript $(document).ready(function() { // Select all paragraphs and change their text color $("p").css("color", "blue"); });
In this code snippet, jQuery’s $ function selects all <p> elements and applies a blue text color to them. This simplicity and efficiency of selectors significantly reduce the amount of code you need to write.
1.3. Event Handling Made Easy
jQuery also excels in simplifying event handling. Attaching event listeners to elements and executing functions based on user interactions becomes much more straightforward with jQuery’s syntax. Here’s a basic example of attaching a click event to a button:
javascript $(document).ready(function() { // Attach a click event to a button $("button").click(function() { alert("Button clicked!"); }); });
In this snippet, when any <button> element is clicked, an alert with the message “Button clicked!” will appear. jQuery abstracts the complexities of cross-browser event handling, making your code more readable and maintainable.
2. What is JSON?
2.1. JSON Basics
JSON, short for JavaScript Object Notation, is a lightweight data interchange format. It’s widely used for structuring data that can be easily understood by humans and processed by machines. JSON is often used for transmitting data between a server and a web application, making it a crucial component in modern web development.
2.2. The JSON Structure
JSON data is organized into key-value pairs, similar to JavaScript objects. Each key is a string enclosed in double quotes, followed by a colon and its corresponding value. Values can be strings, numbers, booleans, arrays, or even nested objects. Here’s a simple example:
json { "name": "John Doe", "age": 30, "isStudent": false, "hobbies": ["reading", "gaming", "swimming"] }
JSON’s straightforward structure makes it easy to parse and generate data programmatically, making it an excellent choice for exchanging information between different systems.
2.3. JSON vs. Other Data Formats
JSON is often compared to XML (eXtensible Markup Language), another data interchange format. While both have their merits, JSON has gained significant popularity due to its simplicity and ease of use. JSON’s syntax is more lightweight, making it easier to read and generate, especially for developers. Additionally, JSON’s compatibility with JavaScript means that parsing and handling JSON data in a web environment is seamless.
3. Manipulating Data with jQuery and JSON
3.1. Loading External Data with jQuery
Fetching data from external sources, such as APIs, is a common requirement in web development. jQuery’s AJAX functionality simplifies this process. Let’s say you want to retrieve and display data from a JSON API:
javascript $(document).ready(function() { $.ajax({ url: "https://api.example.com/data", method: "GET", dataType: "json", success: function(data) { // Manipulate and display the retrieved data $("#result").text("Fetched data: " + data.name); }, error: function() { $("#result").text("Error fetching data"); } }); });
In this example, jQuery’s AJAX function is used to make a GET request to an API. Upon success, the retrieved data’s “name” property is displayed on the page. On error, an error message is shown instead.
3.2. Modifying HTML with jQuery
jQuery’s DOM manipulation capabilities are a game-changer when it comes to dynamically updating and modifying HTML content. Whether you want to add new elements, change text, or modify attributes, jQuery provides a simple and efficient way to accomplish these tasks. Consider the following example:
javascript $(document).ready(function() { // Add a new list item to an unordered list $("button").click(function() { $("ul").append("<li>New item</li>"); }); });
In this snippet, clicking a button triggers the addition of a new list item to an unordered list. This dynamic interaction enhances user experience and engagement on your web page.
3.3. Transforming Data with JSON
Combining jQuery’s DOM manipulation with JSON data can lead to impressive outcomes. Let’s say you have retrieved JSON data and want to display it in an organized manner on your webpage:
javascript $(document).ready(function() { $.ajax({ url: "data.json", method: "GET", dataType: "json", success: function(data) { // Iterate through JSON data and create HTML elements data.forEach(function(item) { var listItem = "<li>Name: " + item.name + ", Age: " + item.age + "</li>"; $("ul").append(listItem); }); }, error: function() { $("#result").text("Error fetching data"); } }); });
In this example, the retrieved JSON data containing names and ages is iterated through, and corresponding list items are dynamically created and added to an unordered list.
4. Advanced Techniques
4.1. Chaining jQuery Methods
One of the key benefits of jQuery is the ability to chain multiple methods together, resulting in more concise and readable code. This technique is often referred to as “method chaining.” Consider the following snippet:
javascript $(document).ready(function() { // Chain multiple jQuery methods $("p") .css("color", "green") .slideUp(1000) .slideDown(1000); });
In this example, the selected <p> elements have their text color changed to green, then slide up, and finally slide down, all in a smooth sequence.
4.2. Error Handling with JSON
While JSON is a robust data format, error handling is essential when working with external data sources. When using jQuery to fetch and parse JSON data, the .error() method can be used to handle any issues that arise during the process. Here’s an example:
javascript $(document).ready(function() { $.ajax({ url: "data.json", method: "GET", dataType: "json", success: function(data) { // Process JSON data }, error: function() { $("#result").text("Error fetching or parsing data"); } }); });
By incorporating error handling, you ensure that your application gracefully handles situations where data retrieval or parsing encounters problems.
4.3. Optimizing Performance
When working with large datasets or numerous elements on a page, optimizing performance becomes crucial. Utilize jQuery’s built-in optimization techniques, such as caching selectors, minimizing DOM manipulation, and avoiding excessive animations. These practices can significantly enhance the responsiveness and speed of your web application.
Conclusion
jQuery and JSON are invaluable tools for modern web developers seeking efficient and effective ways to manipulate data. By harnessing the power of jQuery’s selectors, event handling capabilities, and DOM manipulation functions, combined with JSON’s simplicity and versatility in structuring data, you can elevate your web development projects to new heights. Whether you’re loading external data, dynamically updating HTML content, or transforming JSON data into user-friendly displays, these technologies empower you to create seamless and interactive user experiences. As you delve deeper into these tools and explore advanced techniques, you’ll discover a world of possibilities for data manipulation that truly allow you to work like a pro.
Table of Contents
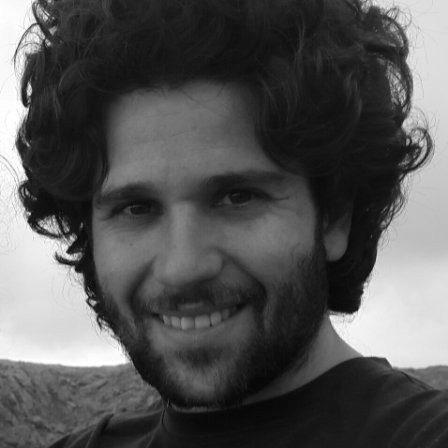
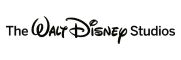