jQuery and JSONP: Cross-Domain Data Retrieval Made Easy
In the dynamic landscape of web development, the need to retrieve data from external sources has become an essential aspect of creating rich and interactive websites. However, the browser’s same-origin policy often poses a challenge when trying to access data from a different domain. This is where jQuery and JSONP come to the rescue, providing a seamless way to overcome cross-domain limitations and retrieve data effortlessly.
Table of Contents
1. Understanding Cross-Domain Data Retrieval
Cross-domain data retrieval refers to the process of fetching data from a different domain or server than the one hosting the current web page. Browsers implement a security feature known as the same-origin policy, which restricts these requests to prevent potential security vulnerabilities. While this policy is crucial for maintaining user privacy and security, it can hinder the functionality of web applications that require data from various sources.
Consider a scenario where you want to display live weather updates on your website. The weather data might be hosted on a different server. Without the proper mechanism, the same-origin policy would block your request, and you wouldn’t be able to fetch the data directly from that server.
2. Introducing JSONP
JSONP, or “JSON with Padding,” is a technique that allows data to be retrieved from different domains while circumventing the same-origin policy limitation. It works by creating a script tag dynamically to load data from an external source. JSONP leverages the fact that script tags are exempt from the same-origin policy, making it possible to request data from other domains without encountering security issues.
Let’s take a closer look at how JSONP works with the help of an example. Assume you want to retrieve and display data from an external API that returns JSON-formatted data. Here’s how you can achieve this using jQuery and JSONP:
Step 1: Include jQuery Library
To get started, make sure you have the jQuery library included in your project. You can download it and link to it in your HTML file or use a content delivery network (CDN) for quicker access:
html <script src="https://code.jquery.com/jquery-3.5.1.min.js"></script>
Step 2: Making a JSONP Request
Now, let’s make a JSONP request to fetch data from an external API. In this example, we’ll retrieve weather data from a hypothetical API:
javascript function handleWeatherData(data) { // Process and display the weather data console.log(data); } $(document).ready(function() { // Create a script tag with the API URL var apiUrl = "https://external-api.com/weather?callback=handleWeatherData"; var script = document.createElement("script"); script.src = apiUrl; // Append the script tag to the document document.body.appendChild(script); });
In the above code, the handleWeatherData function will be called when the data is loaded. The API URL includes a callback parameter with the value set to the name of the function that should be executed with the retrieved data. This is a crucial step in the JSONP process, as it allows the external server to wrap the data in the specified function call.
3. Benefits of JSONP
JSONP offers several benefits when it comes to cross-domain data retrieval:
- Simplicity: Implementing JSONP is straightforward and requires minimal code changes. It’s an excellent choice for quick integration.
- Widely Supported: JSONP is supported by most browsers, making it a reliable choice for cross-domain data retrieval.
- Compatibility: JSONP can be used with APIs that support it, making it a viable option for accessing data from various sources.
- No Server-Side Changes: JSONP doesn’t require any changes to the server’s configuration or code, making it easy to use even with external APIs you don’t control.
4. Potential Drawbacks and Security Considerations
While JSONP offers a convenient way to retrieve data from different domains, it’s essential to be aware of its limitations and potential security risks:
- Security: Since JSONP requires executing external scripts on your page, there’s a risk of running malicious code. Always ensure that you trust the source of the data you’re fetching.
- Limited to GET Requests: JSONP only supports GET requests, which may limit the types of interactions you can have with the external server.
- No Error Handling: JSONP lacks robust error handling. If the request fails, it might not provide detailed error messages, making troubleshooting challenging.
- Dependency on Server Support: JSONP relies on the server’s ability to wrap data in a callback function. Not all APIs support JSONP, which could limit its usability in some cases.
Conclusion
In the world of modern web development, cross-domain data retrieval is a common requirement, but it comes with its share of challenges due to the same-origin policy. JSONP, with its ability to bypass this limitation, provides a simple and effective solution for fetching data from different domains seamlessly. By integrating JSONP with jQuery, developers can enhance the interactivity and functionality of their web applications without sacrificing security.
Remember that while JSONP offers a convenient way to access cross-domain data, it’s crucial to weigh its benefits against its limitations and potential security risks. Always prioritize security and ensure you’re using reputable data sources when implementing JSONP in your projects. With the right considerations in place, jQuery and JSONP can be powerful tools in your toolkit for creating dynamic and feature-rich web applications.
Table of Contents
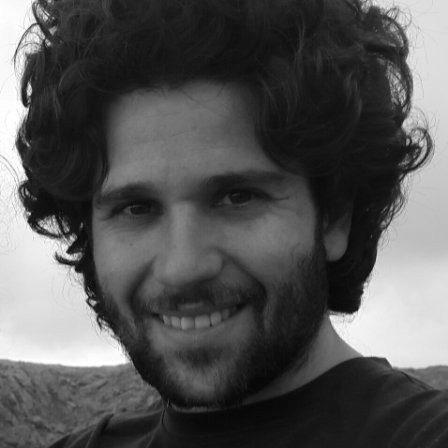
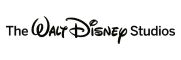