jQuery and Local Storage: Storing Data on the Client Side
In today’s web development landscape, the ability to store data on the client side has become an essential aspect of creating dynamic and interactive web applications. jQuery, a popular JavaScript library, offers a convenient and efficient way to manipulate the Document Object Model (DOM) and interact with various aspects of web pages. When coupled with the power of Local Storage, developers can seamlessly store and retrieve data on the client side, enhancing user experiences and improving application performance.
Table of Contents
1. Understanding Local Storage
Before diving into the integration of jQuery with Local Storage, let’s first understand what Local Storage is. Local Storage is a web storage solution that allows developers to store key-value pairs in a web browser. This storage persists even after the user closes the browser or navigates away from the page. This makes it an ideal choice for storing small amounts of data that need to be accessed across different sessions.
2. Local Storage has several advantages:
2.1. Persistent Data Storage
Unlike session storage, Local Storage retains data even after the browser window is closed. This makes it suitable for storing preferences, settings, or other persistent user data.
2.2. Larger Data Capacity
Local Storage offers a larger storage capacity compared to cookies, allowing developers to store more substantial amounts of data on the client side.
2.3. Simplicity
Local Storage is easy to use and doesn’t require complex configurations. It provides a straightforward key-value storage mechanism.
3. Integrating jQuery with Local Storage
jQuery simplifies many aspects of client-side scripting, including DOM manipulation and event handling. When combined with Local Storage, it provides a robust solution for managing data on the client side.
3.1. Setting Up Local Storage
Before you can start using Local Storage with jQuery, you need to understand how to set it up. Here’s a step-by-step guide:
3.1.1. Checking for Browser Support
Before utilizing Local Storage, ensure that the user’s browser supports it. Modern browsers provide excellent support, but it’s always a good practice to perform a quick check:
javascript if (typeof(Storage) !== "undefined") { // Local Storage is supported } else { // Local Storage is not supported in this browser }
3.1.2. Storing Data
Storing data in Local Storage is a breeze. Use the localStorage.setItem() method to store key-value pairs:
javascript // Storing data localStorage.setItem("username", "john_doe");
3.1.3. Retrieving Data
Retrieving data is just as straightforward. Utilize the localStorage.getItem() method to access the stored value:
javascript // Retrieving data var username = localStorage.getItem("username");
3.1.4. Removing Data
If you need to remove a stored item, the localStorage.removeItem() method comes in handy:
javascript // Removing data localStorage.removeItem("username");
4. Leveraging jQuery for Enhanced Functionality
Now that you have a grasp of how Local Storage works, let’s explore how jQuery can enhance the functionality of data storage on the client side.
4.1. Simplified DOM Manipulation
jQuery’s core strength lies in its ability to simplify complex DOM manipulation tasks. Instead of writing verbose JavaScript code to manipulate elements, you can achieve the same results with fewer lines of code using jQuery. This ease of use extends to handling Local Storage as well.
4.2. Event Handling
jQuery provides a streamlined way to handle various events in your web application. Whether it’s listening for clicks, form submissions, or keyboard interactions, jQuery’s event handling mechanisms make it easy to respond to user actions and update Local Storage accordingly.
4.3. Animation and Effects
If your application involves animations or visual effects, jQuery’s animation capabilities can greatly enhance the user experience. These animations can be used to provide feedback when data is being stored or retrieved from Local Storage, creating a more polished interface.
5. Implementing jQuery and Local Storage: A Practical Example
Let’s walk through a practical example of using jQuery and Local Storage to create a simple “to-do list” application. In this example, we’ll demonstrate how to store and display tasks using Local Storage and leverage jQuery for smooth interactions.
5.1. HTML Structure
First, let’s define the basic HTML structure for our “to-do list” application:
html <!DOCTYPE html> <html> <head> <title>jQuery and Local Storage Example</title> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> </head> <body> <h1>To-Do List</h1> <input type="text" id="taskInput" placeholder="Enter a new task"> <button id="addTask">Add Task</button> <ul id="taskList"></ul> </body> </html>
5.2. JavaScript Implementation
Next, let’s implement the JavaScript functionality using jQuery and Local Storage:
javascript $(document).ready(function() { // Load tasks from Local Storage var tasks = JSON.parse(localStorage.getItem("tasks")) || []; // Display tasks on the page function displayTasks() { $("#taskList").empty(); tasks.forEach(function(task) { $("#taskList").append("<li>" + task + "</li>"); }); } // Add new task $("#addTask").click(function() { var newTask = $("#taskInput").val(); if (newTask !== "") { tasks.push(newTask); localStorage.setItem("tasks", JSON.stringify(tasks)); displayTasks(); $("#taskInput").val(""); } }); // Initial display of tasks displayTasks(); });
In this example, we’ve created a simple interface where users can input tasks, add them to the list, and see the tasks persist even after the browser is closed. jQuery simplifies the process of handling user interactions and updating Local Storage with the new task data.
Conclusion
jQuery and Local Storage are powerful tools for enhancing client-side web development. By integrating jQuery’s simplicity and efficiency with Local Storage’s persistent data storage capabilities, developers can create engaging and dynamic applications that offer a seamless user experience. Whether you’re building a to-do list application or a more complex web application, mastering the combination of jQuery and Local Storage opens up a world of possibilities for data management on the client side. So, dive in, experiment, and take your web development skills to new heights!
Table of Contents
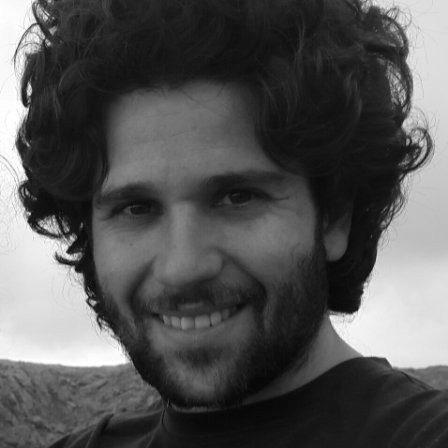
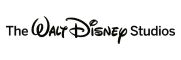