Mastering AJAX with jQuery: Seamless Asynchronous Communication
In the ever-evolving world of web development, creating smooth and responsive user experiences is paramount. One of the key technologies that enable this is AJAX (Asynchronous JavaScript and XML). AJAX allows websites to fetch and exchange data with a server in the background without requiring a page refresh. This not only enhances user experience by providing real-time updates but also improves performance by reducing server load.
jQuery, a fast, feature-rich JavaScript library, simplifies the process of implementing AJAX functionality. In this comprehensive guide, we’ll delve into mastering AJAX with jQuery to achieve seamless asynchronous communication on your website. By the end, you’ll have the skills to take your web projects to the next level.
1. Introduction to AJAX and jQuery
1.1 What is AJAX?
AJAX, or Asynchronous JavaScript and XML, is a set of web development techniques that allow you to update parts of a web page without requiring a full page reload. This means that the user can interact with the website while the data is fetched or submitted in the background. AJAX accomplishes this by using JavaScript to communicate with the server and manipulate the DOM (Document Object Model) based on the server’s response.
1.2 The Role of jQuery in AJAX
jQuery simplifies the process of implementing AJAX functionality by providing a set of easy-to-use methods. Instead of writing complex JavaScript code to handle AJAX requests, jQuery allows you to achieve the same functionality with just a few lines of code. It provides a simple and intuitive API to make AJAX requests, handle responses, and deal with errors effectively.
2. Setting Up the Environment
2.1 Integrating jQuery into Your Project
Before diving into AJAX with jQuery, you need to include the jQuery library in your project. You have several options for doing this, such as downloading the jQuery library and hosting it locally, using a CDN (Content Delivery Network), or installing it via a package manager like npm or yarn.
For example, to include jQuery using a CDN, add the following script tag to the head section of your HTML file:
html <!DOCTYPE html> <html> <head> <title>My Website</title> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> </head> <body> <!-- Your website content here --> </body> </html>
2.2 The Basics of HTML and CSS
Since AJAX is primarily about dynamically updating the content of a webpage, a fundamental understanding of HTML and CSS is essential. Ensure that you are familiar with creating HTML elements, setting up forms, and applying CSS styles. This knowledge will be crucial as we proceed with AJAX integration.
3. Making Your First AJAX Request
3.1 Sending Data to the Server
Making an AJAX request with jQuery is straightforward. The jQuery.ajax() method is the core of handling AJAX requests. To make a basic GET request to a server, you can use the following code:
javascript $.ajax({ url: "https://api.example.com/data", method: "GET", success: function(data) { // Handle the successful response here }, error: function(xhr, status, error) { // Handle errors here } });
In this example, we specify the URL we want to fetch data from, set the HTTP method to GET, and provide two callback functions: one for handling successful responses and another for handling errors.
3.2 Handling the Server Response
Once the server responds to the AJAX request, the success function is executed if the request was successful. In this function, you can manipulate the data received from the server and update the DOM accordingly.
javascript success: function(data) { // Process the data and update the DOM $("#result").text("Data received: " + data); }
On the other hand, if an error occurs during the AJAX request, the error function will be executed. This function allows you to handle error scenarios gracefully and provide meaningful feedback to the user.
javascript error: function(xhr, status, error) { // Handle errors and display a message $("#error").text("An error occurred: " + error); }
With this basic AJAX setup, you can already create dynamic web pages that fetch data from the server asynchronously. However, AJAX has much more to offer, and we’ll explore further capabilities in the following sections.
4. Performing GET and POST Requests
4.1 Fetching Data with GET
The example in the previous section demonstrated a basic GET request, which is ideal for retrieving data from the server. However, you often need to include parameters in your requests to fetch specific data or filter results. You can achieve this by appending the parameters to the URL using the data option in the ajax() method.
javascript $.ajax({ url: "https://api.example.com/data", method: "GET", data: { key: "value", param: "data" }, success: function(data) { // Handle the successful response here }, error: function(xhr, status, error) { // Handle errors here } });
In this example, we are appending two parameters, “key” and “param,” to the URL. On the server side, you can access these parameters and process the request accordingly.
4.2 Submitting Data with POST
In contrast to GET requests, which are used for data retrieval, POST requests are used to send data to the server, such as form submissions. When making a POST request with jQuery, you need to specify the HTTP method as “POST” and provide the data in the data option.
javascript $.ajax({ url: "https://api.example.com/submit", method: "POST", data: { name: "John Doe", email: "john@example.com" }, success: function(response) { // Handle the successful response here }, error: function(xhr, status, error) { // Handle errors here } });
In this example, we are sending form data (name and email) to the server. Remember that sensitive data, such as passwords, should never be sent via a GET request, as it would be visible in the URL. Always use a POST request for such data.
Handling Parameters and Payloads
When dealing with complex requests that require additional headers, custom configurations, or authentication tokens, you can provide more options in the ajax() method. For instance, you can set custom headers using the headers option:
javascript $.ajax({ url: "https://api.example.com/data", method: "GET", headers: { "Authorization": "Bearer your_auth_token" }, success: function(data) { // Handle the successful response here }, error: function(xhr, status, error) { // Handle errors here } });
Similarly, you can send JSON data as the request payload by setting the contentType and data options:
javascript $.ajax({ url: "https://api.example.com/submit", method: "POST", contentType: "application/json", data: JSON.stringify({ name: "John Doe", email: "john@example.com" }), success: function(response) { // Handle the successful response here }, error: function(xhr, status, error) { // Handle errors here } });
The contentType option ensures that the server correctly interprets the payload as JSON data.
5. Managing JSON Data
5.1 Understanding JSON
JSON (JavaScript Object Notation) is a lightweight data-interchange format commonly used for data exchange between a server and a web application. JSON is easy for humans to read and write and easy for machines to parse and generate. It consists of key-value pairs and arrays, making it an ideal format for transferring structured data.
For example, here’s a simple JSON object representing a person:
json { "name": "John Doe", "age": 30, "email": "john@example.com" }
5.2 Parsing JSON Responses
When the server responds to an AJAX request with JSON data, you need to parse it to access its contents and use them on your web page. jQuery makes JSON parsing easy with the $.parseJSON() method.
javascript $.ajax({ url: "https://api.example.com/data", method: "GET", success: function(data) { // Parse the JSON response var parsedData = $.parseJSON(data); // Access individual properties var name = parsedData.name; var age = parsedData.age; var email = parsedData.email; // Update the DOM with the parsed data $("#name").text("Name: " + name); $("#age").text("Age: " + age); $("#email").text("Email: " + email); }, error: function(xhr, status, error) { // Handle errors here } });
In this example, we receive a JSON response from the server, parse it into a JavaScript object, and then update the DOM with the extracted data.
5.3 Stringifying Data for Server Communication
When sending complex data to the server, such as nested objects or arrays, you need to convert the JavaScript object to a JSON string. jQuery provides the JSON.stringify() method for this purpose.
javascript var dataToSend = { name: "Jane Doe", age: 25, hobbies: ["Reading", "Painting", "Traveling"] }; $.ajax({ url: "https://api.example.com/submit", method: "POST", contentType: "application/json", data: JSON.stringify(dataToSend), success: function(response) { // Handle the successful response here }, error: function(xhr, status, error) { // Handle errors here } });
In this example, we stringify the dataToSend object and send it as the payload in a POST request to the server.
6. Handling AJAX Events
6.1 AJAX Event Handlers in jQuery
jQuery provides a set of event handlers that allow you to hook into various stages of the AJAX process. These handlers are useful for performing actions before and after the AJAX request, as well as handling global AJAX events that apply to all AJAX requests on the page.
beforeSend Event
The beforeSend event is triggered before the AJAX request is sent to the server. It is often used to set up custom headers, show loading spinners, or perform any preprocessing before the request is initiated.
javascript $.ajax({ url: "https://api.example.com/data", method: "GET", beforeSend: function(xhr) { // Perform actions before sending the request $("#loader").show(); xhr.setRequestHeader("Authorization", "Bearer your_auth_token"); }, success: function(data) { // Handle the successful response here $("#loader").hide(); }, error: function(xhr, status, error) { // Handle errors here $("#loader").hide(); } });
In this example, we show a loading spinner (#loader) before sending the AJAX request and hide it once the response is received.
complete Event
The complete event is triggered after the AJAX request is completed, regardless of whether it was successful or encountered an error. It is useful for performing cleanup tasks or actions that need to be executed regardless of the request’s outcome.
javascript $.ajax({ url: "https://api.example.com/data", method: "GET", success: function(data) { // Handle the successful response here }, error: function(xhr, status, error) { // Handle errors here }, complete: function() { // Perform actions after the request is complete $("#loader").hide(); } });
In this example, we hide the loading spinner (#loader) once the request is complete, whether it succeeded or failed.
6.2 Global AJAX Event Handlers
In addition to the per-request event handlers, jQuery also allows you to define global AJAX event handlers that apply to all AJAX requests made on the page. These global handlers can be useful for implementing common functionalities that apply across your entire website.
To set up global event handlers, you can use the $.ajaxSetup() method. For example, to display a loading spinner for all AJAX requests, you can do the following:
javascript $(document).ajaxStart(function() { // Show the loading spinner when any AJAX request starts $("#loader").show(); }); $(document).ajaxStop(function() { // Hide the loading spinner when all AJAX requests are complete $("#loader").hide(); });
In this example, the ajaxStart event is triggered when any AJAX request starts, and the ajaxStop event is triggered when all AJAX requests are complete. We use these events to show and hide the loading spinner (#loader) accordingly.
6.3 Error Handling and Debugging
Error handling is a critical aspect of AJAX development. When an AJAX request fails, you’ll want to provide clear and helpful feedback to the user and possibly log the error for debugging purposes.
javascript $.ajax({ url: "https://api.example.com/data", method: "GET", success: function(data) { // Handle the successful response here }, error: function(xhr, status, error) { // Handle errors here if (xhr.status === 404) { $("#error").text("Resource not found."); } else if (xhr.status === 500) { $("#error").text("Internal server error."); } else { $("#error").text("An unknown error occurred."); } console.log("AJAX Error: " + error); } });
In this example, we check the status code of the AJAX response to determine the type of error that occurred. Based on the status code, we display an appropriate error message to the user. Additionally, we log the error to the console for debugging purposes.
7. Implementing AJAX with Forms
7.1 Validating Form Data
When using AJAX to submit forms, it’s crucial to validate the form data on the client-side before sending it to the server. Client-side validation helps ensure that only valid data is submitted and reduces unnecessary server requests.
html <form id="myForm"> <input type="text" name="username" required> <input type="email" name="email" required> <button type="submit">Submit</button> </form> javascript $("#myForm").submit(function(event) { event.preventDefault(); if (this.checkValidity()) { // Form is valid, proceed with AJAX submission submitFormWithAJAX(); } else { // Display validation errors to the user this.reportValidity(); } }); function submitFormWithAJAX() { var formData = $("#myForm").serialize(); $.ajax({ url: "https://api.example.com/submit", method: "POST", data: formData, success: function(response) { // Handle the successful response here }, error: function(xhr, status, error) { // Handle errors here } }); }
In this example, we first prevent the default form submission behavior using event.preventDefault(). We then check the form’s validity using this.checkValidity(). If the form is valid, we proceed with AJAX form submission by calling the submitFormWithAJAX() function. Otherwise, we display validation errors to the user using this.reportValidity().
7.2 AJAX Form Submission
Once the form data is validated, we can use AJAX to submit the form data to the server. We serialize the form data using the serialize() method, which converts it into a query string that can be sent in the request.
javascript function submitFormWithAJAX() { var formData = $("#myForm").serialize(); $.ajax({ url: "https://api.example.com/submit", method: "POST", data: formData, success: function(response) { // Handle the successful response here alert("Form submitted successfully!"); }, error: function(xhr, status, error) { // Handle errors here alert("An error occurred: " + error); } }); }
In this example, we send the serialized form data to the server using a POST request. Upon a successful submission, we display an alert to the user, and in case of an error, we display an alert with the error message.
7.3 Displaying Server-Side Validation Errors
When submitting forms via AJAX, the server may respond with validation errors if the data is invalid. To handle server-side validation errors, we need to parse the JSON response and display the error messages to the user.
javascript function submitFormWithAJAX() { var formData = $("#myForm").serialize(); $.ajax({ url: "https://api.example.com/submit", method: "POST", data: formData, success: function(response) { // Handle the successful response here alert("Form submitted successfully!"); }, error: function(xhr, status, error) { // Handle errors here if (xhr.status === 422) { // Parse the JSON error response var errors = $.parseJSON(xhr.responseText); // Display validation errors to the user for (var key in errors) { $("#" + key + "-error").text(errors[key][0]); } } else { alert("An error occurred: " + error); } } }); }
In this example, we handle a possible 422 status code, which is typically used to indicate validation errors on the server side. We parse the JSON error response and display the validation error messages next to the corresponding form fields.
8. Enhancing User Experience with AJAX
8.1 Implementing Infinite Scroll
Infinite scroll is a popular technique used to improve user experience when dealing with long lists of data. Instead of loading all the data at once, you load a small portion of it initially and then fetch more data as the user scrolls down the page.
html <div id="posts"> <!-- Initially loaded posts go here --> </div> <div id="loading" style="display: none;"> Loading more posts... </div> javascript var isLoading = false; var page = 1; $(window).scroll(function() { if ($(window).scrollTop() + $(window).height() >= $("#posts").height() && !isLoading) { loadMorePosts(); } }); function loadMorePosts() { isLoading = true; $("#loading").show(); $.ajax({ url: "https://api.example.com/posts", method: "GET", data: { page: page }, success: function(data) { // Append the new posts to the existing list $("#posts").append(data); // Increment the page number for the next request page++; // Reset the loading state isLoading = false; $("#loading").hide(); }, error: function(xhr, status, error) { // Handle errors here isLoading = false; $("#loading").hide(); } }); }
In this example, we use the $(window).scroll() event to detect when the user has scrolled to the bottom of the page. If the page has not been reached before and the loading indicator is not already visible, we trigger the loadMorePosts() function. This function fetches additional posts from the server, appends them to the existing list, and updates the page number for the next request.
8.2 Dynamic Content Loading
Dynamic content loading involves fetching and injecting specific parts of a page dynamically without the need to reload the entire page. This technique is often used for implementing tabbed navigation, expanding content sections, or showing pop-ups.
html <div id="tabContent"> <!-- Dynamic content will be loaded here --> </div> <ul id="tabs"> <li><a href="#" data-tab="tab1">Tab 1</a></li> <li><a href="#" data-tab="tab2">Tab 2</a></li> <li><a href="#" data-tab="tab3">Tab 3</a></li> </ul> javascript $("#tabs a").click(function(event) { event.preventDefault(); var tab = $(this).data("tab"); loadTabContent(tab); }); function loadTabContent(tab) { $.ajax({ url: "https://api.example.com/tab/" + tab, method: "GET", success: function(data) { // Inject the fetched content into the tabContent div $("#tabContent").html(data); }, error: function(xhr, status, error) { // Handle errors here } }); }
In this example, we use the click() event to handle clicks on the tab links. When a tab link is clicked, we prevent the default link behavior with event.preventDefault(). We then extract the data-tab attribute of the clicked link and use it to determine which tab’s content to load. The loadTabContent() function fetches the corresponding content from the server and injects it into the #tabContent div.
8.3 Real-Time Notifications
Real-time notifications keep users informed about important updates or events on the website. With AJAX and jQuery, you can easily implement real-time notifications that automatically update without the need to refresh the page.
html <div id="notifications"> <!-- Real-time notifications will be displayed here --> </div> javascript Copy code function fetchNotifications() { $.ajax({ url: "https://api.example.com/notifications", method: "GET", success: function(data) { // Display the new notifications $("#notifications").html(data); }, error: function(xhr, status, error) { // Handle errors here } }); } // Fetch notifications every 10 seconds setInterval(fetchNotifications, 10000);
In this example, we define the fetchNotifications() function to fetch the latest notifications from the server. We then use setInterval() to call this function every 10 seconds, refreshing the notifications dynamically without the user needing to manually refresh the page.
Conclusion
Mastering AJAX with jQuery opens up a world of possibilities for creating seamless, interactive, and responsive web applications. By harnessing the power of asynchronous communication, you can enhance user experience, improve performance, and keep your website up-to-date with real-time data.
In this guide, we covered the basics of AJAX, integrating jQuery into your project, making AJAX requests, handling JSON data, and implementing various AJAX functionalities. Additionally, we explored error handling, debugging, and enhancing user experience through dynamic content loading, infinite scrolling, and real-time notifications.
Remember to always optimize your AJAX requests, handle errors gracefully, and validate data both on the client and server sides. With these skills in your toolkit, you are well on your way to becoming a master of AJAX with jQuery and creating cutting-edge web experiences for your users. Happy coding!
Table of Contents
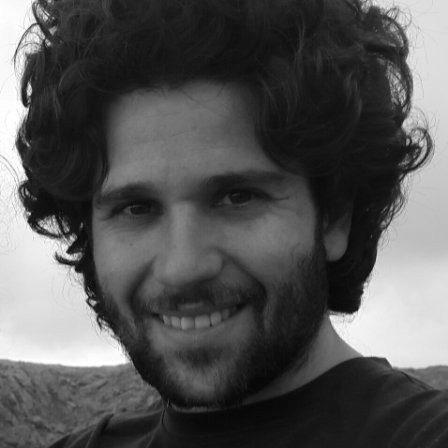
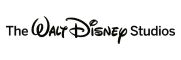