Mastering jQuery Events: From Basics to Advanced Concepts
In the world of web development, interactivity is key to creating engaging user experiences. jQuery, a fast, small, and feature-rich JavaScript library, has been a cornerstone of web development for years, enabling developers to efficiently manipulate the DOM and handle various events with ease. In this comprehensive guide, we will take you on a journey through the realm of jQuery events, starting from the fundamentals and progressing to advanced concepts that will empower you to create dynamic and interactive web applications.
Table of Contents
1. Introduction to jQuery Events
1.1. What are jQuery Events?
Events are actions or occurrences that happen in the browser, such as a user clicking a button, hovering over an element, or typing into a text input. jQuery events provide a way to capture and respond to these interactions, making your web applications more interactive and engaging.
1.2. Event Binding
Event binding is the process of attaching an event handler function to a specific element on your webpage. This function will be executed when the specified event occurs on that element. For instance, you can bind a click event handler to a button so that when a user clicks the button, a particular action is performed.
javascript // Binding a click event handler to a button $("#myButton").click(function() { alert("Button clicked!"); });
1.3. Common Event Types
jQuery supports a wide range of events, including but not limited to:
- click: Occurs when an element is clicked.
- hover: Triggers when the mouse enters or leaves an element.
- keydown and keyup: Fire when a key is pressed down or released.
- submit: Fires when a form is submitted.
- focus and blur: Triggered when an element gains or loses focus.
2. Basic Event Handling
2.1. Click Events
Click events are among the most commonly used events in web development. They allow you to respond to user clicks on elements like buttons, links, or images. Let’s say you have a button with the ID #submitButton. Here’s how you can bind a click event to it:
javascript $("#submitButton").click(function() { // Perform actions when the button is clicked });
2.2. Hover Events
Hover events are used to detect when the mouse enters or leaves an element. These events are useful for creating interactive effects like tooltips or changing an element’s appearance when hovered over.
javascript $("#myElement").hover( function() { // Code to run when mouse enters the element }, function() { // Code to run when mouse leaves the element } );
2.3. Input Events
Input events are triggered when the user interacts with input fields, such as text inputs, checkboxes, or radio buttons. You can use these events to validate user input in real-time or update other parts of the page.
javascript $("input[type='text']").on("input", function() { // Code to validate or respond to input changes });
2.4. Event Object
When an event occurs, jQuery automatically passes an event object to the event handler function. This object contains information about the event, including the target element, event type, and any additional data related to the event. You can access this information within the event handler.
javascript $("button").click(function(event) { console.log("Clicked element:", event.target); console.log("Event type:", event.type); // Other event-related properties });
3. Event Propagation and Delegation
3.1. Event Bubbling and Capturing
Event propagation refers to the way events travel through the DOM tree. When an event occurs on an element, it can propagate either in a bubbling phase (from the target element up to the root) or in a capturing phase (from the root down to the target element). Understanding event propagation is crucial for managing event interactions effectively.
3.2. Event Delegation Advantages
Event delegation is a powerful technique in jQuery where you attach a single event handler to a common ancestor element of multiple target elements. This technique offers several benefits, such as better memory usage, efficient management of dynamically added elements, and improved performance.
3.3. Implementing Event Delegation
Suppose you have a list of items, and you want to perform a specific action when any of the items are clicked. Instead of binding an individual event handler to each item, you can use event delegation:
javascript $("#itemList").on("click", "li", function() { // Code to handle the click event for list items });
4. Advanced Event Concepts
4.1. Custom Events
In addition to the built-in events, jQuery allows you to create custom events. These events can be triggered manually and provide a way to define your own interactions and behaviors.
javascript // Creating and triggering a custom event $("#myElement").on("customEvent", function() { // Custom event handler logic }); $("#myElement").trigger("customEvent");
4.2. Event Chaining
jQuery allows you to chain multiple event handlers together. This can be especially useful when you want to perform a sequence of actions in response to a single event.
javascript $("#myElement").click(function() { $(this).hide().fadeIn().addClass("active"); });
4.3. Namespaced Events
Event namespaces allow you to organize and manage your event handlers more efficiently. This is particularly handy when you need to unbind specific event handlers without affecting others.
javascript $("#myElement").on("click.myNamespace", function() { // Event handler logic }); // Unbind only the events in the "myNamespace" namespace $("#myElement").off("click.myNamespace");
4.4. Debouncing and Throttling
Debouncing and throttling are techniques to control the frequency of event handler execution. Debouncing ensures that an event handler is triggered only after a specified delay of inactivity, while throttling limits the rate at which the handler is executed.
javascript // Debouncing an input event var debounceTimer; $("input").on("input", function() { clearTimeout(debounceTimer); debounceTimer = setTimeout(function() { // Code to run after a delay }, 300); });
5. Best Practices for Efficient Event Handling
5.1. Limiting Global Event Handlers
Avoid attaching event handlers directly to the document or window objects, as this can lead to performance issues. Instead, attach handlers to specific elements whenever possible.
5.2. Unbinding Events
When you’re done with an element or no longer need its event handlers, it’s important to unbind the events to prevent memory leaks. You can use the off() method to remove specific event handlers.
5.3. Using on() vs bind()
While both on() and bind() can be used to attach event handlers, it’s recommended to use on(), as it supports event delegation and is more versatile.
5.4. Performance Considerations
Excessive use of event handlers, especially on elements with frequent updates, can impact the performance of your web application. Always consider performance implications and optimize your event handling code when necessary.
6. Real-World Examples
6.1. Creating Interactive Image Galleries
Learn how to build an image gallery that responds to clicks, enabling users to view images in a larger format or in a lightbox overlay.
javascript $(".image-thumbnail").click(function() { var imageURL = $(this).attr("src"); // Display the clicked image });
6.2. Building Dynamic Form Validation
Enhance user experience by providing real-time feedback on form input validity using input event handlers.
javascript $("input[type='email']").on("input", function() { var email = $(this).val(); // Validate email format and provide feedback });
6.3. Implementing Infinite Scroll
Create a seamless scrolling experience by loading more content as the user scrolls down the page.
javascript $(window).on("scroll", function() { if ($(window).scrollTop() + $(window).height() >= $(document).height()) { // Load more content } });
6.4. Enhancing User Experience with Tooltips
Utilize hover events to show tooltips with additional information when users hover over certain elements.
javascript $(".tooltip-trigger").hover( function() { var tooltipText = $(this).data("tooltip"); // Show tooltip with tooltipText }, function() { // Hide tooltip } );
Conclusion
Mastering jQuery events takes your web development skills to the next level. From understanding the basics of event handling to implementing advanced concepts like event delegation and custom events, you now have the tools to create dynamic and interactive web applications. By following best practices and exploring real-world examples, you’ll be able to craft seamless user experiences that keep visitors engaged and delighted. So, dive into the world of jQuery events and elevate your web development prowess today!
Table of Contents
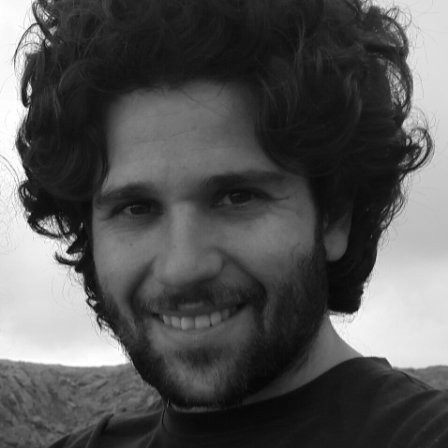
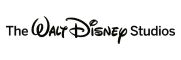