Building Multi-Level Navigation Menus with jQuery
Navigation menus play a crucial role in website design, providing visitors with a clear and structured way to access various sections of a website. While simple navigation menus are effective for smaller websites, as websites grow in complexity, multi-level navigation menus become essential. These menus allow you to organize content hierarchically and provide users with a seamless browsing experience. In this tutorial, we’ll explore how to build dynamic multi-level navigation menus using jQuery, a popular JavaScript library.
Table of Contents
1. Understanding the Need for Multi-Level Navigation Menus
Modern websites often house a vast amount of content, ranging from articles, products, and categories to different sections and features. As a result, presenting this content in an organized and accessible manner is essential to keep users engaged and satisfied. Multi-level navigation menus, also known as dropdown menus or flyout menus, enable websites to structure content hierarchically, making it easier for users to find what they’re looking for.
2. The Challenge of Multi-Level Menus
Building multi-level navigation menus from scratch can be challenging due to various factors:
- Complexity: Multi-level menus involve more than just a list of links. They require the ability to display submenus, handle user interactions, and manage various states.
- Responsive Design: With the increasing use of mobile devices, it’s crucial to ensure that multi-level menus work seamlessly on both desktop and mobile screens.
- User Experience: Creating menus that are intuitive and easy to navigate enhances user satisfaction. It’s important to consider user interactions and animations to create a delightful experience.
3. Getting Started with jQuery
jQuery is a fast, small, and feature-rich JavaScript library that simplifies many tasks involving HTML document traversal and manipulation, event handling, and animation. It provides an efficient way to interact with the Document Object Model (DOM) and create dynamic web content.
4. Linking jQuery to Your Webpage
Before you start building your multi-level navigation menu, make sure you have jQuery linked to your webpage. You can include jQuery by adding the following code within the <head> section of your HTML document:
html <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
5. Creating the HTML Structure
Let’s begin by creating a simple HTML structure for our navigation menu. We’ll use an unordered list (<ul>) to represent the menu and list items (<li>) for each menu item. Submenus will also be represented using nested <ul> elements. Here’s an example:
html <nav id="multi-level-menu"> <ul> <li><a href="#">Home</a></li> <li><a href="#">About Us</a> <ul> <li><a href="#">History</a></li> <li><a href="#">Team</a></li> <li><a href="#">Mission</a></li> </ul> </li> <li><a href="#">Services</a> <ul> <li><a href="#">Web Design</a></li> <li><a href="#">Development</a></li> <li><a href="#">SEO</a></li> </ul> </li> <li><a href="#">Portfolio</a></li> <li><a href="#">Contact</a></li> </ul> </nav>
In this example, we’ve created a basic menu structure with two levels of nesting. The main menu items have associated submenus that appear when the user hovers over or clicks on the main item.
6. Adding Interactivity with jQuery
Now that we have our HTML structure in place, let’s add interactivity to our multi-level navigation menu using jQuery.
7. Show and Hide Submenus
We’ll start by making the submenus appear and disappear when the user interacts with the main menu items. We’ll use jQuery’s .hover() function to achieve this effect. Add the following jQuery code within a <script> tag:
html <script> $(document).ready(function() { $('#multi-level-menu li').hover( function() { $(this).find('ul:first').slideDown(); }, function() { $(this).find('ul:first').slideUp(); } ); }); </script>
In this code, we’re using the .hover() function to define two functions: one for when the user hovers over a menu item (mouseenter) and another for when they move the cursor away from the menu item (mouseleave). When the user hovers over a menu item, the associated submenu is slid down, and when they move away, the submenu is slid up to hide it.
8. Adding Smooth Animations
To make our menu feel more polished, we can add smooth animations when showing and hiding submenus. We’ll use jQuery’s .slideDown() and .slideUp() functions with a duration parameter. Update the jQuery code as follows:
html <script> $(document).ready(function() { $('#multi-level-menu li').hover( function() { $(this).find('ul:first').slideDown(200); }, function() { $(this).find('ul:first').slideUp(200); } ); }); </script>
By specifying a duration of 200 milliseconds, we’re creating a subtle animation effect that enhances the user experience.
9. Adding Click Functionality for Mobile
On mobile devices, hovering isn’t feasible. Instead, we can use jQuery to toggle submenus when a user clicks on a menu item. This is particularly important for responsive design. Update the jQuery code to include click functionality:
html <script> $(document).ready(function() { // Hide all submenus initially $('#multi-level-menu ul').hide(); // Toggle submenu on click $('#multi-level-menu li').click(function(e) { e.stopPropagation(); // Prevent parent item's hover effect $(this).find('ul:first').slideToggle(200); }); }); </script>
In this code, we’re first hiding all submenus using .hide(). Then, when a menu item is clicked, we use .slideToggle() to show or hide the associated submenu.
10. Styling the Menu with CSS
While jQuery handles the interactivity, CSS plays a crucial role in styling the menu to make it visually appealing and aligned with your website’s design. You can use CSS to customize the appearance of the menu items, submenus, and animations.
10.1. Basic Styling
Here’s an example of basic CSS styling for our multi-level menu:
css #multi-level-menu { background-color: #333; color: #fff; } #multi-level-menu ul { list-style: none; padding: 0; margin: 0; } #multi-level-menu li { display: inline-block; position: relative; padding: 10px 20px; } #multi-level-menu li:hover { background-color: #555; } #multi-level-menu ul ul { display: none; position: absolute; top: 100%; left: 0; background-color: #444; z-index: 1; } #multi-level-menu ul ul li { display: block; width: 200px; } #multi-level-menu ul ul li a { color: #fff; padding: 10px; text-decoration: none; display: block; } #multi-level-menu ul ul li:hover { background-color: #666; }
In this CSS code, we’re styling the menu’s background, colors, spacing, and animations. You can customize these styles to match your website’s branding.
Conclusion
Building multi-level navigation menus with jQuery is a powerful way to enhance the user experience on your website. By providing organized access to content, you can keep users engaged and satisfied while making it easier for them to navigate through your website’s offerings. Remember to consider responsive design principles and user-friendly animations to create a seamless browsing experience. With jQuery’s capabilities and a bit of CSS styling, you can create dynamic and visually appealing multi-level menus that cater to the needs of your audience. Experiment, iterate, and refine your menus to provide a user-friendly interface that guides visitors through your website’s content effortlessly.
Table of Contents
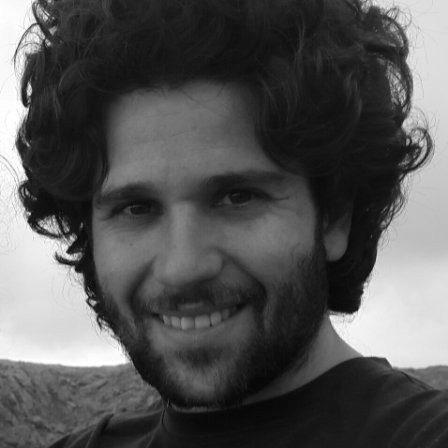
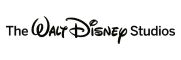