Simplifying Navigation Menus with jQuery Responsive Nav
In today’s digital age, having a user-friendly and responsive website is paramount. One critical aspect of user experience is navigation menus. A well-organized and responsive navigation menu ensures that your visitors can effortlessly explore your website, regardless of the device they’re using. In this blog post, we’ll dive into the world of jQuery Responsive Nav and discover how it can help simplify navigation menus, making them adaptable and visually appealing across all screen sizes.
Table of Contents
1. Why Navigation Menus Matter
Navigation menus are the backbone of any website. They guide users to different sections, pages, and content. A poorly designed or inefficient navigation menu can frustrate users and lead to high bounce rates. Here’s why navigation menus are crucial:
1.1. User Experience
An intuitive navigation menu enhances the user experience. Users can quickly find what they’re looking for, improving their overall satisfaction.
1.2. Mobile Responsiveness
With the increasing use of smartphones and tablets, it’s vital to have a navigation menu that adapts to smaller screens. Responsive navigation ensures that your website is accessible on all devices.
1.3. SEO Benefits
Search engines like Google consider the structure and user-friendliness of your website when ranking it. A well-structured navigation menu can improve your site’s SEO.
1.4. Branding and Consistency
A well-designed navigation menu can reinforce your brand identity and maintain consistency throughout your website.
Now that we understand the importance of navigation menus, let’s explore how jQuery Responsive Nav can help us simplify the process.
2. Introducing jQuery Responsive Nav
jQuery Responsive Nav is a lightweight JavaScript library that makes it easy to create responsive navigation menus. It simplifies the process of designing and implementing menus that work seamlessly on both desktop and mobile devices. Here are some of its key features:
2.1. Easy Setup
jQuery Responsive Nav is incredibly easy to set up. You don’t need to be a coding expert to implement it on your website.
2.2. Customization
It offers a high degree of customization, allowing you to tailor the menu’s appearance and behavior to match your website’s design.
2.3. Cross-Browser Compatibility
The library ensures cross-browser compatibility, so your navigation menu will work consistently across different browsers.
2.4. Smooth Animations
jQuery Responsive Nav includes smooth animations that enhance the user experience when opening and closing the menu.
2.5. Accessibility
It is built with accessibility in mind, ensuring that users with disabilities can navigate your website with ease.
Now, let’s get into the nitty-gritty of implementing jQuery Responsive Nav on your website.
3. Implementation Steps
Follow these steps to simplify your navigation menus using jQuery Responsive Nav:
3.1. Include jQuery and Responsive Nav
First, make sure you include jQuery and the Responsive Nav library in your HTML file. You can either download them and host them locally or use a Content Delivery Network (CDN). Here’s an example of including them via CDN:
html <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Your Website</title> <link rel="stylesheet" href="styles.css"> </head> <body> <nav id="myMenu"> <!-- Your navigation menu items go here --> </nav> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> <script src="https://cdn.jsdelivr.net/responsive-nav/1.0.39/responsive-nav.min.js"></script> <script src="script.js"></script> </body> </html>
In this example, we’ve included jQuery, Responsive Nav, and a custom JavaScript file (script.js) that we’ll create next.
3.2. Initialize Responsive Nav
Create a JavaScript file (in this case, script.js) and initialize Responsive Nav like this:
javascript $(document).ready(function() { var navigation = responsiveNav("#myMenu"); });
This code initializes Responsive Nav on an element with the ID myMenu. Make sure the ID matches your actual navigation menu’s ID.
3.3. HTML Structure
Now, let’s set up the HTML structure for your navigation menu. Here’s a basic example:
html <nav id="myMenu"> <ul> <li><a href="#">Home</a></li> <li><a href="#">About</a></li> <li><a href="#">Services</a></li> <li><a href="#">Portfolio</a></li> <li><a href="#">Contact</a></li> </ul> </nav>
You can customize this structure to suit your website’s needs.
3.4. CSS Styling
To make your navigation menu visually appealing, add some CSS styles. Here’s a simple example:
css /* Basic styling for the navigation menu */ #myMenu { background-color: #333; } #myMenu ul { list-style: none; padding: 0; } #myMenu ul li { display: inline-block; margin-right: 20px; } #myMenu ul li a { color: #fff; text-decoration: none; } /* Media query for mobile responsiveness */ @media screen and (max-width: 768px) { #myMenu ul { text-align: center; } #myMenu ul li { display: block; margin: 10px 0; } }
This CSS code provides a basic style for the navigation menu and includes a media query to ensure it’s responsive on smaller screens.
3.5. Customize Menu Behavior
You can further customize the menu behavior by passing options to the responsiveNav function. For example, to change the menu button’s label and toggle animation, you can do the following:
javascript $(document).ready(function() { var navigation = responsiveNav("#myMenu", { label: "Menu", // Change the menu button label animate: true, // Enable menu toggle animation }); });
These are just a few customization options available with jQuery Responsive Nav. Explore the library’s documentation to tailor the menu to your specific requirements.
4. Testing and Optimization
After implementing your responsive navigation menu, it’s essential to test it thoroughly on different devices and screen sizes. Ensure that it looks and functions as expected. Here are some tips for testing and optimization:
4.1. Mobile-Friendly Testing
Use tools like Google’s Mobile-Friendly Test to check if your menu is mobile responsive.
4.2. Cross-Browser Testing
Test your navigation menu on various web browsers to ensure compatibility.
4.3. Accessibility Testing
Check the menu’s accessibility using tools like WAVE or Axe to make sure it’s usable for all users.
4.4. Performance Optimization
Optimize your website’s performance by minifying and compressing your CSS and JavaScript files.
4.5. User Feedback
Collect user feedback to identify any issues or areas for improvement regarding your navigation menu.
Conclusion
In conclusion, jQuery Responsive Nav is a powerful tool for simplifying navigation menus on your website. With its ease of use, customization options, and responsiveness, you can create a user-friendly and visually appealing menu system that enhances the overall user experience. Remember to test and optimize your menu to ensure it performs flawlessly across all devices. So, why wait? Start implementing jQuery Responsive Nav today and elevate your website’s navigation to the next level.
Table of Contents
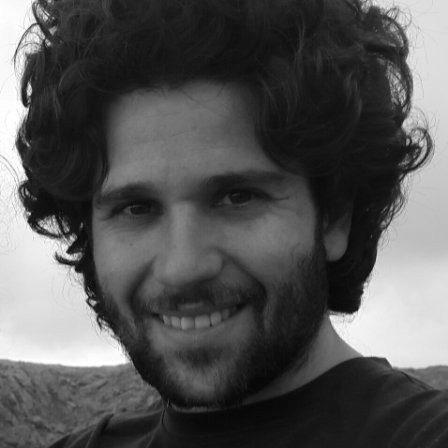
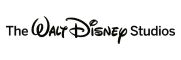