Optimizing Mobile Performance with jQuery Mobile
In today’s fast-paced digital landscape, mobile devices have become the primary means of accessing the internet. As a result, it’s crucial for web developers to ensure that their mobile websites are not only visually appealing but also performant. Slow-loading websites can lead to frustrated users and high bounce rates. This is where jQuery Mobile comes into play, offering a powerful framework to create responsive and efficient mobile web applications. In this article, we’ll explore essential strategies, tips, and code samples for optimizing mobile performance using jQuery Mobile.
Table of Contents
1. Understanding the Importance of Mobile Performance
Before diving into the details of optimizing mobile performance with jQuery Mobile, let’s briefly discuss why it matters. Mobile users have different expectations and needs compared to desktop users. They’re often on the go and looking for quick access to information. Slow-loading websites can lead to:
- Increased Bounce Rates: Users are more likely to leave a website if it takes too long to load, leading to higher bounce rates.
- Negative User Experience: Slow performance can result in a frustrating user experience, tarnishing your brand’s reputation.
- SEO Impact: Page load speed is a crucial factor in search engine rankings. Slow websites might rank lower in search results.
- Reduced Conversions: Performance issues can hinder users from completing desired actions like purchases or sign-ups.
Considering these factors, optimizing mobile performance is not just a technical concern; it directly affects user engagement and business outcomes.
2. Leveraging jQuery Mobile for Mobile Performance
jQuery Mobile is a powerful JavaScript framework designed specifically for building mobile web applications. It provides a variety of features and tools to streamline the development process and enhance user experience. Here are some key aspects of jQuery Mobile that contribute to mobile performance:
2.1. Responsive Design and Layouts
Responsive design is at the core of jQuery Mobile’s philosophy. It ensures that your website looks and functions seamlessly across various screen sizes and orientations. By using responsive grids and components, you can create layouts that adapt to the device’s screen, minimizing the need for unnecessary content scaling and reducing load times.
html <div data-role="page"> <div data-role="header"> <h1>My Mobile App</h1> </div> <div data-role="content"> <p>Welcome to my responsive app!</p> </div> <div data-role="footer"> <h4>© 2023 My App</h4> </div> </div>
2.2. Lazy Loading of Content
Loading all content upfront can slow down your mobile website. jQuery Mobile offers lazy loading capabilities, allowing you to load content as users scroll down the page. This technique reduces initial load times and provides a smoother user experience.
javascript $(document).on("pagecreate", "#myPage", function() { $("#lazyContent").load("lazy-content.html"); });
2.3. Optimized Image Handling
Images are often the heaviest assets on a web page. jQuery Mobile provides tools to optimize image loading, such as automatically resizing images based on device capabilities and screen sizes.
html <img src="image.jpg" alt="Optimized Image" class="ui-responsive">
2.4. Minimized JavaScript and CSS
Large JavaScript and CSS files can slow down rendering times. jQuery Mobile allows you to create custom builds that include only the components you need, reducing the overall file size and boosting performance.
html <link rel="stylesheet" href="jquery.mobile.custom.min.css"> <script src="jquery.mobile.custom.min.js"></script>
3. Best Practices for Optimizing jQuery Mobile Performance
While jQuery Mobile provides tools to enhance mobile performance, it’s essential to follow best practices to ensure optimal results. Here are some tips to consider:
3.1. Reduce HTTP Requests
Each HTTP request adds to the load time of your website. Combine and minimize your JavaScript and CSS files to reduce the number of requests made by the browser.
3.2. Use Hardware Acceleration
Leverage hardware acceleration for animations and transitions. This offloads the rendering process from the CPU to the GPU, resulting in smoother animations and improved performance.
css .ui-page { transform: translate3d(0, 0, 0); }
3.3. Optimize Touch Events
jQuery Mobile provides touch events to enhance the user experience. However, using too many touch event listeners can impact performance. Delegate touch events to parent elements to minimize the listener count.
javascript $(document).on("tap", ".myButton", function() { // Handle tap event });
3.4. Minimize DOM Manipulation
Excessive DOM manipulation can lead to reflows and repaints, slowing down your application. Update the DOM efficiently and avoid unnecessary changes.
3.5. Cache Data
Utilize local storage or session storage to cache data that doesn’t change frequently. This reduces the need to fetch data from the server, resulting in quicker load times.
javascript // Store data in local storage localStorage.setItem("cachedData", JSON.stringify(data));
3.6. Test on Real Devices
Always test your mobile website on real devices to identify performance bottlenecks and user experience issues that might not be evident on emulators.
Conclusion
In a mobile-first world, optimizing the performance of your mobile website is paramount. jQuery Mobile offers an array of features and strategies to help developers create fast, responsive, and user-friendly mobile web applications. By embracing responsive design, lazy loading, image optimization, and other best practices, you can deliver a seamless mobile experience that keeps users engaged and satisfied. Remember, a performant mobile website not only enhances user experience but also contributes to your website’s search engine ranking and overall business success. So, dive into the world of jQuery Mobile and take your mobile performance optimization to the next level.
Table of Contents
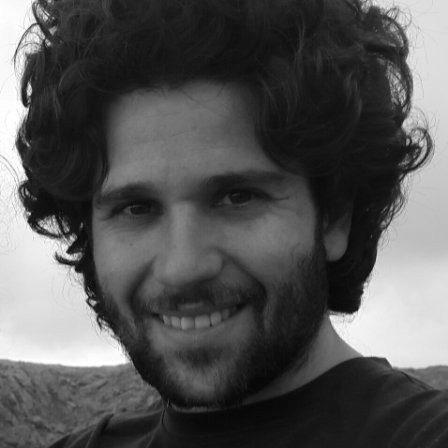
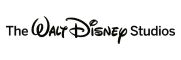